Spring Integration Simplified: Real-Life Scenarios Explained
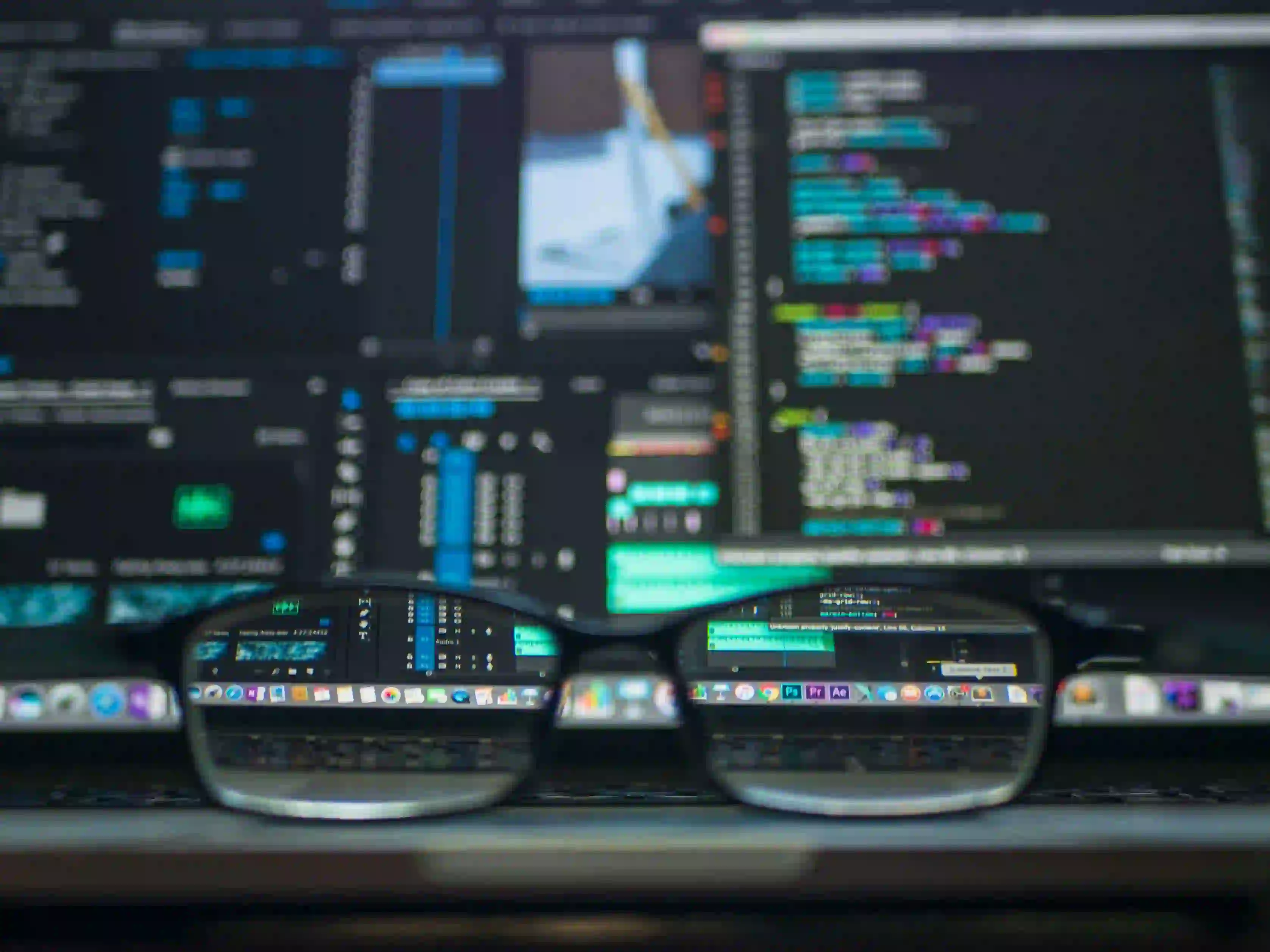
Spring Integration Simplified: Real-Life Scenarios Explained
When it comes to building robust, scalable, and maintainable applications, integrating different systems and components is a common requirement. This is where Spring Integration comes into play. Spring Integration is an extension of the Spring framework that facilitates the integration of enterprise applications.
In this blog post, we will delve into Spring Integration, exploring real-life scenarios and providing simplified explanations. We will walk through common integration challenges and demonstrate how Spring Integration can elegantly address them.
What is Spring Integration?
Spring Integration is an open-source framework that provides an implementation of the Enterprise Integration Patterns. It enables the creation of messaging and integration solutions in a lightweight and straightforward manner. By embracing the principles of Spring, it allows seamless integration with other Spring technologies.
Real-Life Scenarios Explained
Let's explore two common real-life scenarios where Spring Integration shines:
Scenario 1: File Transfer
Many applications require the transfer of files between different systems. This could involve receiving files from an FTP server, transforming them, and then storing them in a database. Spring Integration provides a robust solution for handling such file transfer scenarios.
Code Example: Configuring an Inbound Channel Adapter for FTP
@Bean
public IntegrationFlow ftpInboundFlow() {
return IntegrationFlows.from(Files.inboundAdapter(new File("input"))
.filter(new SimplePatternFileListFilter("*.txt")),
e -> e.poller(Pollers.fixedDelay(1000)))
.transform(Transformers.fileToString())
.handle(System.out::println)
.get();
}
Why: Here, we configure an inbound channel adapter for FTP using Spring Integration. The Files.inboundAdapter
sets up a polling inbound channel adapter for the specified directory, while the transform
and handle
functions handle the file transformation and subsequent processing.
Scenario 2: Message Routing
In a microservices architecture, message routing is a fundamental component. Different services may need to communicate with each other through messaging systems such as Apache Kafka or RabbitMQ. Spring Integration offers powerful message routing capabilities that streamline the handling of such scenarios.
Code Example: Configuring a Message Router
@Bean
public IntegrationFlow messageRoutingFlow() {
return f -> f
.<String, Boolean>route(payload -> payload.contains("important"),
mapping -> mapping
.subFlowMapping(true, sf -> sf.handle(m -> ...))
.subFlowMapping(false, sf -> sf.channel("ignoreChannel")));
}
Why: In this example, we configure a message router using Spring Integration. The route
function routes messages based on a condition, in this case, whether the message contains "important". This directs messages to different sub-flows for further processing or ignores them altogether, showcasing the versatility of message routing in Spring Integration.
Advantages of Using Spring Integration
Now that we've explored real-life scenarios, let's discuss the advantages of using Spring Integration for enterprise application integration.
-
Abstraction of Complex Integration Logic: Spring Integration provides a set of pre-built components and patterns that abstract away the complexity of integrating different systems, allowing developers to focus on the business logic.
-
Testability and Maintainability: With its modular and loosely coupled architecture, Spring Integration promotes testability and maintainability, making it easier to extend and modify integration flows.
-
Interoperability and Extensibility: By leveraging Spring's ecosystem and support for various messaging protocols, Spring Integration offers interoperability and extensibility, enabling integration with diverse systems.
-
Scalability and Performance: Spring Integration is designed to handle high volumes of messages and scale with the application, ensuring robust performance in demanding integration scenarios.
-
Error Handling and Monitoring: It provides comprehensive error handling capabilities and supports integration with monitoring tools, aiding in the identification and resolution of integration issues.
Wrapping Up
In this blog post, we've explored Spring Integration and its application in real-life integration scenarios. From file transfer to message routing, Spring Integration proves to be a versatile and robust framework for addressing enterprise integration challenges. Its intuitive design, seamless integration with other Spring technologies, and powerful capabilities make it a valuable asset for building resilient and efficient integration solutions.
To delve deeper into Spring Integration, check out the official Spring Integration Documentation.
So, the next time you're faced with complex integration requirements, remember that Spring Integration simplifies the intricate world of enterprise integration and empowers you to craft elegant solutions with ease.