JDK 14 Update: Decoding the Early Signs & Impact!
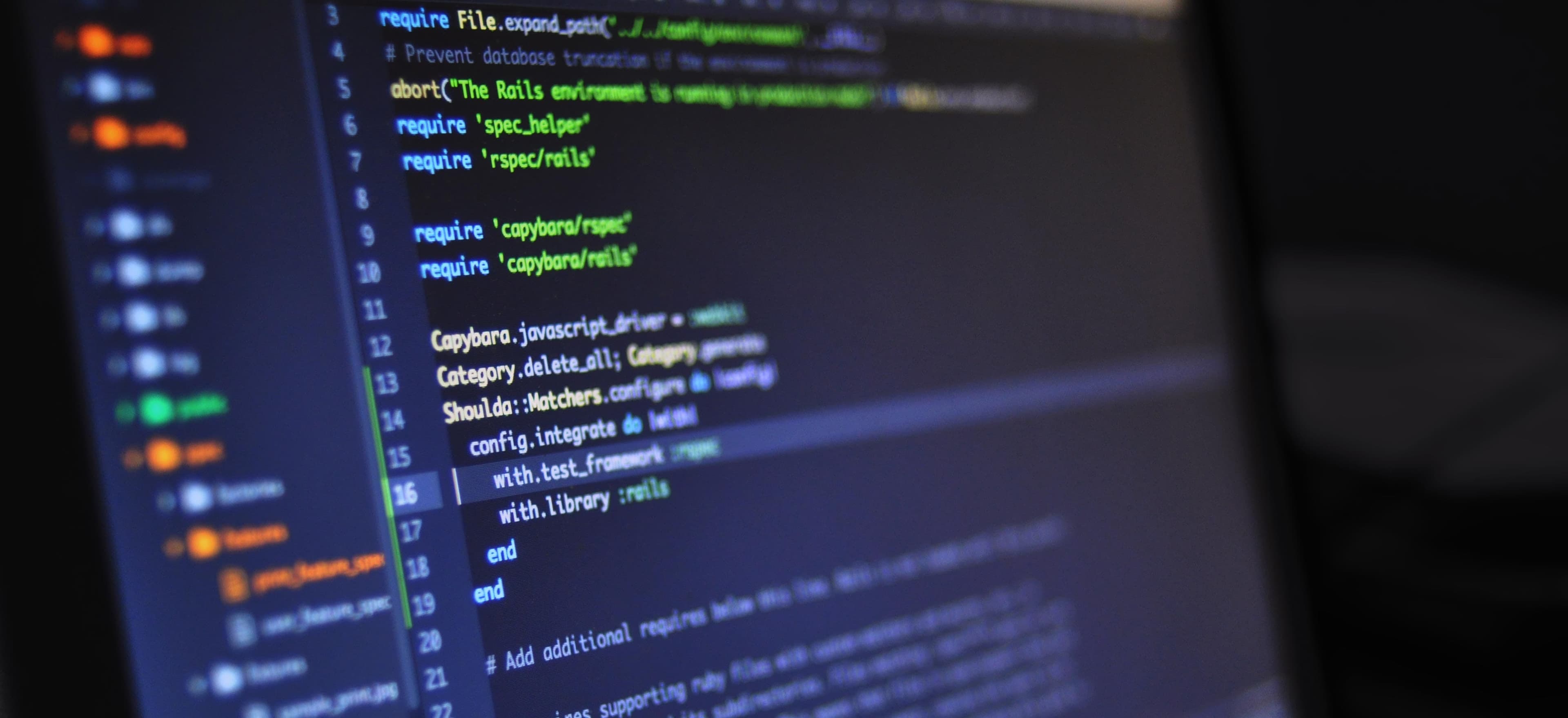
- Published on
JDK 14 Update: Decoding the Early Signs & Impact
Java, the perennial juggernaut of the programming world, continues to evolve with the release of JDK 14. With each new release, Java not only introduces new features and enhancements but also paves the way for new possibilities and improved developer experiences. In this blog post, we'll delve into the early signs and the potential impact of JDK 14.
Pattern Matching for instanceof
One of the most anticipated features in JDK 14 is the addition of pattern matching for the instanceof
operator. This feature allows developers to both test the type of an object and cast it to a variable in a single, concise operation. Let's take a look at an example to understand its significance:
if (obj instanceof String s) {
// s is now a locally scoped variable of type String
// No need to cast obj to String separately
System.out.println(s.length());
}
The addition of pattern matching simplifies the code, making it more readable and expressive. This aligns with Java's ongoing efforts to reduce boilerplate code and enhance developer productivity.
Switch Expressions
JDK 14 also brings improvements to switch expressions. Previously, switch statements were extended to support both statements and expressions. Now, JDK 14 takes this further by making switch expressions a standard feature. They can be used as statements as well as in more concise forms as expressions, resulting in more readable code.
Consider the following example:
int day = 3;
String dayType = switch (day) {
case 1, 2, 3, 4, 5 -> "Weekday";
case 6, 7 -> "Weekend";
default -> throw new IllegalArgumentException("Invalid day: " + day);
};
The introduction of switch expressions aims to simplify the syntax, reduce the propensity for mistakes, and enhance the overall readability of the code.
Records
JDK 14 introduces records as a preview feature. Records provide a compact syntax for declaring classes that are transparent holders for shallowly immutable data. A record, by default, includes compact constructors, accessors, equals()
, hashCode()
, and toString()
methods. This reduces the verbosity of the code and allows developers to focus on the data rather than the implementation details.
public record Point(int x, int y) {}
The above record declaration represents a record Point
with two components: x
and y
. This concise syntax, coupled with the automatically generated methods, streamlines the creation of simple data-centric classes.
The early feedback on records has been quite positive, with developers appreciating the reduction in boilerplate code and the increased expressiveness.
Helpful Null Pointer Exceptions
JDK 14 comes with a highly anticipated feature that aims to mitigate the frustrations caused by ubiquitous null pointer exceptions. This feature involves enhancing the NullPointerException
error messages to provide more helpful diagnostic information, such as the name of the variable that is null.
String name = person.getName();
Previously, encountering a null pointer in the above code would simply reveal that the person
object was null. With the improvement in JDK 14, the error message will specify that it is the name
variable that is null, leading to more straightforward debugging and issue resolution.
Impact on Developer Productivity
The introduction of these new features and improvements in JDK 14 holds significant implications for developer productivity and code quality. The streamlined syntax, reduction in boilerplate code, and enhanced error diagnostics contribute to a more developer-friendly ecosystem.
By allowing developers to write more expressive and succinct code, JDK 14 empowers them to focus on problem-solving and innovation rather than getting bogged down by cumbersome syntax and boilerplate code.
How to Get Started
It's imperative for Java developers to stay abreast of the latest updates and features in JDK 14. If you haven't already, it's a good idea to start exploring the early access builds and familiarize yourself with the new enhancements. Additionally, ensuring compatibility of your existing codebase with JDK 14 and taking advantage of its new features can position you for greater efficiency and maintainability.
My Closing Thoughts on the Matter
JDK 14 brings forth a host of enhancements aimed at simplifying syntax, improving diagnostics, and boosting developer productivity. The early signs indicate that these improvements are well-received by the Java developer community, paving the way for a more refined and efficient programming experience.
As with any major update, it's crucial for developers to adapt and embrace the changes brought about by JDK 14, leveraging its new features to their advantage. By staying informed and proactive, developers can ensure a seamless transition to JDK 14 and harness its capabilities to elevate their coding endeavors.
In conclusion, JDK 14 sets the stage for a more expressive, concise, and developer-friendly Java programming landscape.
Remember to check out the JDK 14 early access builds and start exploring the latest features!
This blog post is a part of our ongoing effort to keep you informed and empowered as a Java developer. Stay tuned for more insights, tutorials, and updates from our Java experts.
Checkout our other articles