Avoid Deadlocks: Mastering Semaphore Locking Techniques
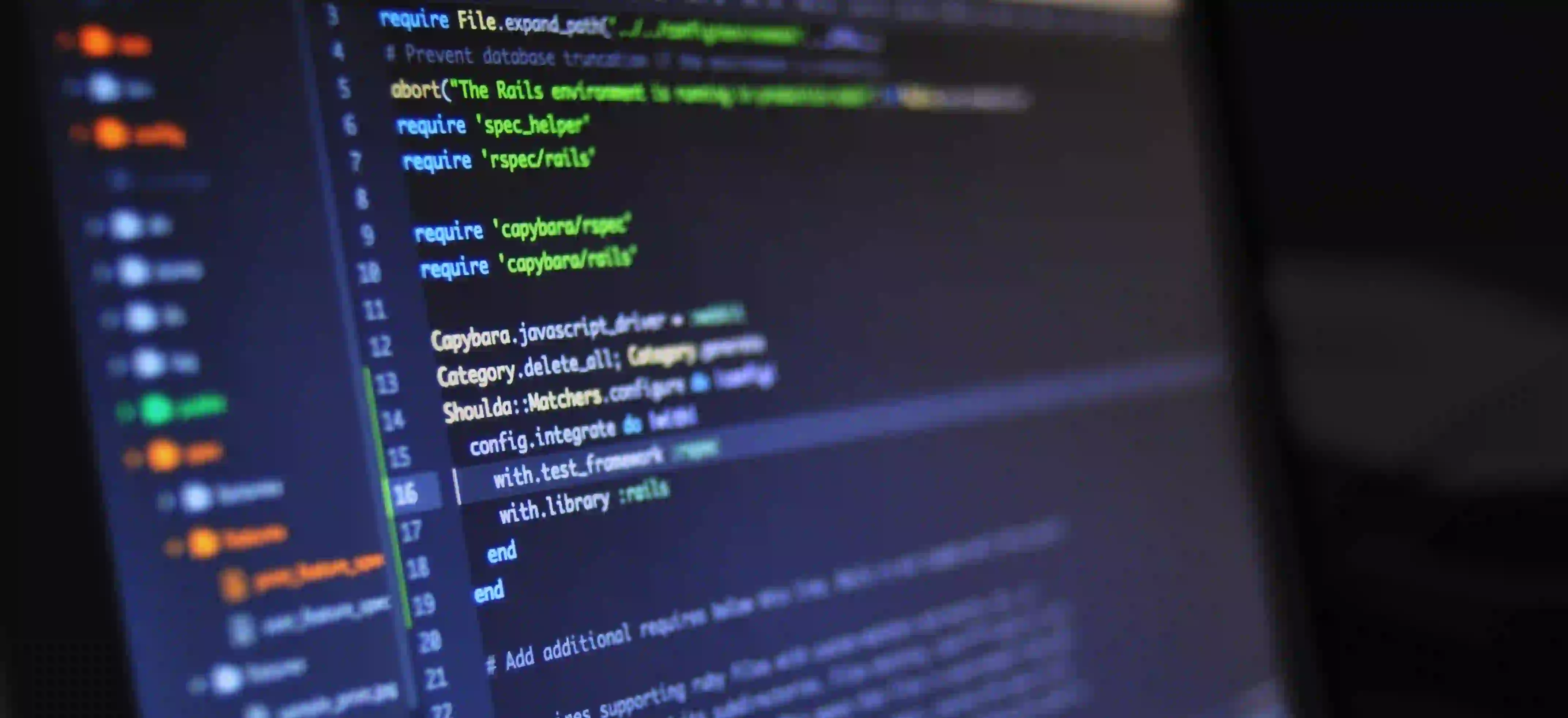
Avoid Deadlocks: Mastering Semaphore Locking Techniques
Deadlocks are a common problem in multithreaded programming, where two or more threads are blocked forever, waiting for each other. Java provides various synchronization mechanisms to handle concurrent access to shared resources, and one of them is semaphores.
In this article, we will explore how to use semaphores in Java to prevent deadlocks and manage access to critical sections of code in a multithreaded environment.
What is a Semaphore?
A semaphore is a synchronization construct that can be used to control access to a shared resource. Unlike other synchronization mechanisms like locks, semaphores can allow multiple threads to access the resource at the same time, up to a certain limit.
Semaphore in Java
In Java, the java.util.concurrent
package provides an implementation of semaphores. The Semaphore
class has two main methods: acquire()
and release()
.
acquire()
The acquire()
method is used to acquire a permit from the semaphore. If no permit is available, the calling thread will block until one is available.
release()
The release()
method is used to release a permit back to the semaphore. This increases the number of available permits.
Preventing Deadlocks with Semaphores
Now, let's look at how to use semaphores to prevent deadlocks in Java.
Example: Dining Philosophers Problem
The dining philosophers problem is a classic synchronization problem where a group of philosophers sit at a dining table and alternate between thinking and eating. There are only a limited number of forks available, and each philosopher requires two forks to eat.
Let's see how semaphores can be used to solve the dining philosophers problem and prevent deadlocks.
The Philosopher
Class
import java.util.concurrent.Semaphore;
public class Philosopher extends Thread {
private Semaphore leftFork, rightFork;
public Philosopher(Semaphore leftFork, Semaphore rightFork) {
this.leftFork = leftFork;
this.rightFork = rightFork;
}
public void run() {
while (true) {
think();
eat();
}
}
private void think() {
// Implement thinking behavior
}
private void eat() {
try {
leftFork.acquire();
rightFork.acquire();
// Implement eating behavior
leftFork.release();
rightFork.release();
}
catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
In the Philosopher
class, each philosopher is represented by a thread. The leftFork
and rightFork
are Semaphores representing the forks on the left and right of the philosopher, respectively.
The eat()
method demonstrates how semaphores are used to acquire and release permits for the forks, preventing deadlocks by ensuring that a philosopher can only eat when both required forks are available.
Why Use Semaphores?
-
Preventing Resource Exhaustion: Semaphores can be used to limit the number of concurrent threads accessing a resource, preventing resource exhaustion and improving system stability.
-
Flexibility: Unlike simple locks, semaphores can be initialized with a count, allowing a fixed number of threads to access a resource simultaneously.
-
Preventing Deadlocks: As demonstrated in the dining philosophers problem, semaphores can be used to prevent deadlock situations by carefully managing concurrent access to shared resources.
To Wrap Things Up
In this article, we have explored how semaphores can be used to prevent deadlocks and manage access to shared resources in a multithreaded Java environment. By understanding and utilizing semaphores effectively, developers can write more robust and stable concurrent programs.
Preventing deadlocks is crucial in multithreaded programming, and semaphores provide a powerful tool to achieve this. With careful design and implementation, semaphores can make concurrent programs more reliable and efficient.
So, the next time you are faced with a multithreaded programming challenge in Java, consider using semaphores to avoid deadlocks and ensure smooth execution of your concurrent code.
Happy coding!
Learn more about Java concurrency and semaphores here.
Note: When using semaphores, it's crucial to release acquired permits in a finally block to handle exceptions and prevent resource leaks.