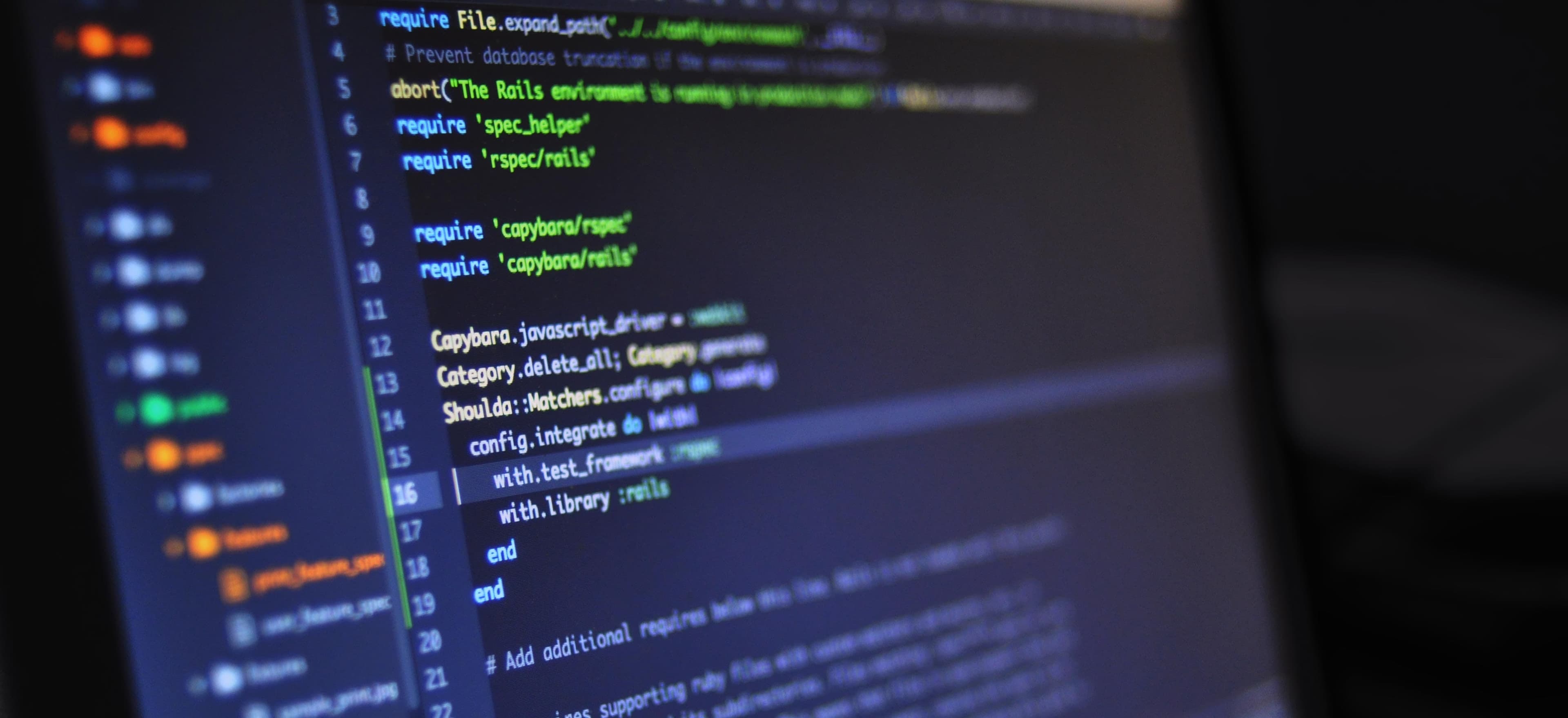
- Published on
Lock Down Your GWT Apps: Integrating Spring Security
When it comes to developing modern web applications in Java, Google Web Toolkit (GWT) remains a formidable choice. Its ability to write front-end code in Java and compile it to highly optimized JavaScript sets it apart. However, securing GWT applications requires a comprehensive approach. In this article, we will explore how to integrate Spring Security into GWT applications to implement robust security measures.
What is Spring Security?
Spring Security is a powerful and highly customizable authentication and access control framework for Java applications. It provides comprehensive security services for Java EE-based enterprise software applications. Spring Security is capable of protecting both the web tier and the service tier of a Spring application, ensuring that security concerns are not fragmented.
Why Integrate Spring Security with GWT?
Integrating Spring Security with GWT applications brings a multitude of benefits. First and foremost, it allows for robust authentication and authorization mechanisms, enabling developers to control access to various parts of the application based on roles and permissions. Additionally, Spring Security provides features such as session management, CSRF protection, and various means of ensuring secure communication.
Setting Up a GWT Project
Before diving into the integration of Spring Security, let's quickly create a simple GWT project. We'll use the GWT Maven archetype to set up the project structure.
<dependency>
<groupId>com.google.gwt</groupId>
<artifactId>gwt-user</artifactId>
<version>2.8.2</version>
</dependency>
<dependency>
<groupId>com.google.gwt</groupId>
<artifactId>gwt-dev</artifactId>
<version>2.8.2</version>
<scope>provided</scope>
</dependency>
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>gwt-maven-plugin</artifactId>
<version>2.8.2</version>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>test</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
This basic setup gives you the foundation to start building your GWT application.
Integrating Spring Security
Now, let's explore how to integrate Spring Security into our GWT application. We will start by configuring Spring Security in our application and then proceed to secure specific parts of the application.
Configuring Spring Security
To integrate Spring Security, we need to create a configuration class that extends WebSecurityConfigurerAdapter
. This class allows us to override and customize security configurations.
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
}
In the above configuration, we allow access to URLs starting with /public/
without authentication and require authentication for all other URLs. We also configure a custom login page and permit access to the logout functionality.
Securing GWT Specific Endpoints
GWT applications often have specific endpoints that need to be secured. To achieve this, we can use method-level security in combination with Spring Security.
@Service
public class GWTService {
@PreAuthorize("hasRole('ROLE_USER')")
public String sensitiveOperation() {
// Perform sensitive operation
return "Sensitive Data";
}
}
In this example, the sensitiveOperation
method is annotated with @PreAuthorize
to ensure that only users with the ROLE_USER
authority can access it.
Closing the Chapter
Integrating Spring Security with GWT applications provides a robust security layer, ensuring that sensitive parts of the application are protected. By configuring security at both the URL level and method level, developers can enforce access control and prevent unauthorized access. Additionally, Spring Security's comprehensive features such as CSRF protection and session management contribute to a more secure application overall.
In conclusion, by integrating Spring Security with your GWT application, you can achieve a high level of security without sacrificing the seamless user experience that GWT provides. This combination empowers developers to build secure, modern web applications with ease.
For more in-depth information on Spring Security, check out the official Spring Security documentation. If you're interested in diving deeper into GWT, refer to the GWT documentation.
Secure your GWT applications with Spring Security and empower your users with the confidence that their data and interactions are protected. Happy coding!
Checkout our other articles