Decoding JVM Internals: The Power of Reflection Unveiled
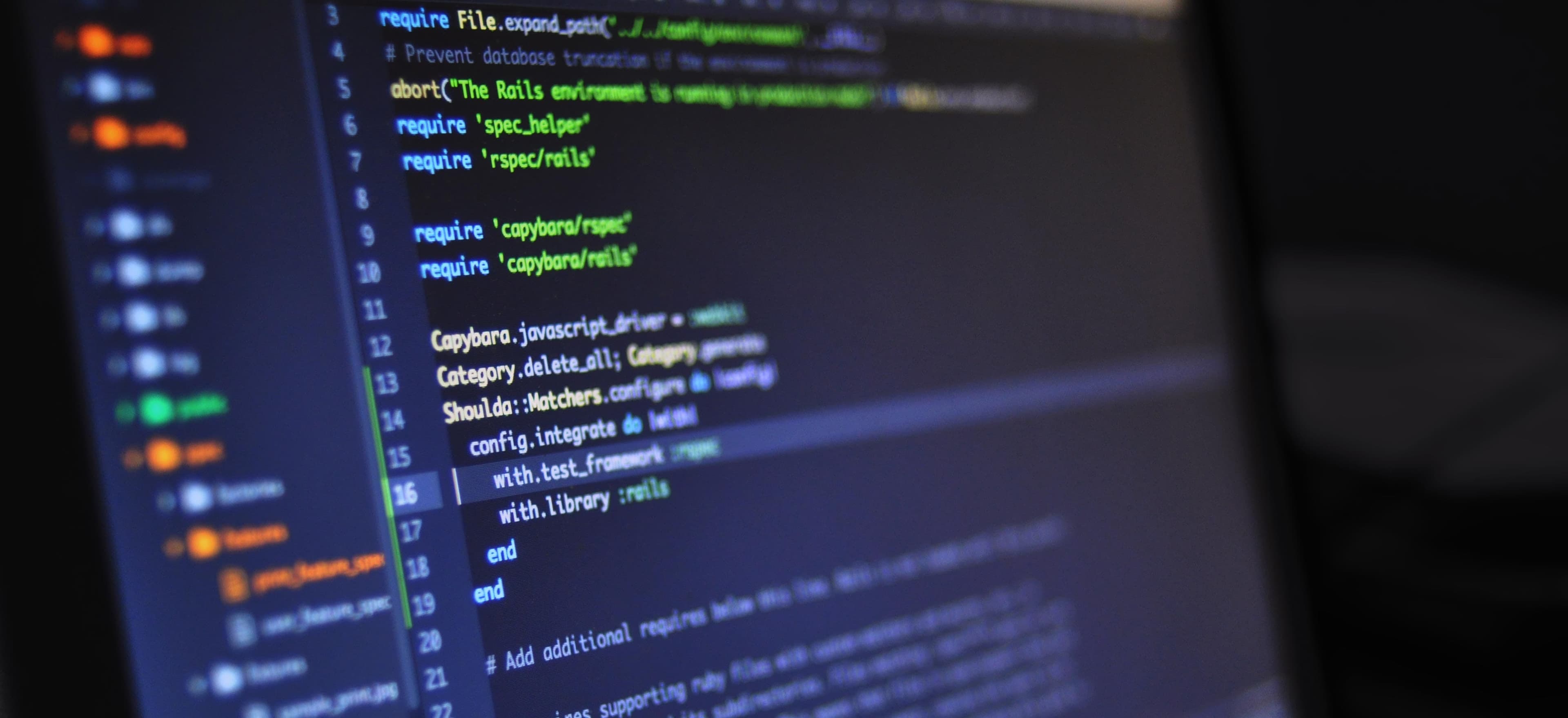
- Published on
Decoding JVM Internals: The Power of Reflection Unveiled
Have you ever wondered about the magic behind Java's ability to introspect and manipulate classes and objects at runtime? The answer lies in a powerful mechanism known as Reflection. In this post, we'll dive deep into the world of Java Reflection and explore its inner workings, use cases, and best practices. So, fasten your seatbelts as we embark on a journey to unveil the mysteries of Java Reflection.
What is Reflection in Java?
Reflection is a feature in Java that allows an application to inspect and modify its own structure at runtime. This includes the ability to examine classes, interfaces, fields, and methods, as well as invoke methods and access fields dynamically. Reflection provides a way to manipulate classes and objects that was not possible through normal static code.
Why Use Reflection?
Reflection opens up a world of possibilities for developers. It enables frameworks like Spring, Hibernate, and JUnit to perform tasks such as dependency injection, mapping objects to database records, and running test cases without needing to know the exact details of the underlying classes.
Reflection can also be useful for building tools like debuggers and profilers, generating code dynamically, and implementing certain design patterns such as the Factory pattern. Although reflection should be used judiciously due to its performance overhead and the potential for breaking encapsulation, it remains a valuable tool in a Java developer's arsenal.
Let's now delve into some fundamental concepts of Reflection and learn how to harness its power.
Getting Class Information
The java.lang.Class
class is the entry point for Reflection. It provides methods to retrieve information about the class, such as its name, modifiers, fields, methods, constructors, and more.
Example 1: Retrieving Class Information
public class ReflectionExample {
public static void main(String[] args) {
Class<Person> personClass = Person.class;
// Getting class name
System.out.println("Class name: " + personClass.getName());
// Getting class modifiers
int modifiers = personClass.getModifiers();
System.out.println("Modifiers: " + Modifier.toString(modifiers));
// Getting fields
Field[] fields = personClass.getDeclaredFields();
System.out.println("Fields:");
for (Field field : fields) {
System.out.println(field.getName());
}
// Getting methods
Method[] methods = personClass.getDeclaredMethods();
System.out.println("Methods:");
for (Method method : methods) {
System.out.println(method.getName());
}
}
}
class Person {
private String name;
public void sayHello() {
System.out.println("Hello!");
}
}
In this example, we obtain the class name, modifiers, fields, and methods using Reflection. This allows us to dynamically inspect the structure of the Person
class at runtime.
Accessing and Modifying Fields
Reflection enables us to access and modify the fields of a class, even if they are private. This can be especially handy when integrating with legacy or third-party code that does not provide public accessors or mutators for its fields.
Example 2: Accessing and Modifying Fields
public class ReflectionExample {
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
Person person = new Person();
Class<? extends Person> personClass = person.getClass();
// Accessing a private field
Field nameField = personClass.getDeclaredField("name");
nameField.setAccessible(true);
String nameValue = (String) nameField.get(person);
System.out.println("Original name: " + nameValue);
// Modifying the field
nameField.set(person, "Alice");
System.out.println("Modified name: " + person.getName());
}
}
class Person {
private String name;
public String getName() {
return name;
}
}
In this example, we use Reflection to access and modify the private name
field of the Person
class. By setting the field to be accessible, we can read and modify its value even though it is private.
Invoking Methods Dynamically
Reflection empowers us to invoke methods on objects dynamically, without knowing their exact signature at compile time. This can be beneficial when designing frameworks that need to call arbitrary user-defined methods, or when implementing scripting languages on top of Java.
Example 3: Invoking Methods Dynamically
public class ReflectionExample {
public static void main(String[] args) throws NoSuchMethodException, InvocationTargetException, IllegalAccessException {
Person person = new Person();
Class<? extends Person> personClass = person.getClass();
// Invoking a method
Method sayHelloMethod = personClass.getDeclaredMethod("sayHello");
sayHelloMethod.invoke(person);
}
}
class Person {
public void sayHello() {
System.out.println("Hello!");
}
}
In this example, we use Reflection to invoke the sayHello
method on the Person
object. This allows us to call the method dynamically, without knowing its details at compile time.
Creating Objects Dynamically
Reflection enables us to create instances of classes dynamically, which can be useful in scenarios where the exact class to be instantiated is determined at runtime. Frameworks like Hibernate use this feature to create entity instances based on metadata obtained from the database.
Example 4: Creating Objects Dynamically
public class ReflectionExample {
public static void main(String[] args) throws ClassNotFoundException, IllegalAccessException, InstantiationException {
Class<?> personClass = Class.forName("Person");
Person person = (Person) personClass.newInstance();
}
}
class Person {
// ...
}
In this example, we use Reflection to dynamically create an instance of the Person
class. This gives us the flexibility to instantiate classes based on their names obtained from external sources.
Performance Considerations
While Reflection is powerful, it comes with a performance overhead. Operations performed through Reflection are slower than their equivalent non-reflective counterparts. Additionally, the compiler cannot perform certain checks on reflective code, which can lead to runtime errors if used incorrectly.
Therefore, it is important to use Reflection judiciously and consider alternative approaches where performance is critical. Where possible, caching reflective objects and results can help mitigate some of the performance costs associated with Reflection.
My Closing Thoughts on the Matter
In this post, we've scratched the surface of Java Reflection and explored its potential to inspect and manipulate classes and objects at runtime. We've seen how Reflection allows us to access class information, modify fields, invoke methods dynamically, and create objects on the fly. While Reflection is a powerful tool, it should be used responsibly and sparingly due to its performance implications and potential for breaking encapsulation.
By gaining a deeper understanding of Java Reflection, you'll be better equipped to leverage its capabilities and wield its power effectively in your Java projects. So, make sure to keep this valuable tool in your arsenal and unleash its potential when the situation calls for it.
Now that we've unveiled the mysteries of Reflection, it's time to roll up our sleeves and explore its applications in real-world scenarios. Keep experimenting, and may the power of Reflection propel your Java journey to new heights!