Mastering MVVM Binding Challenges in ZK Framework
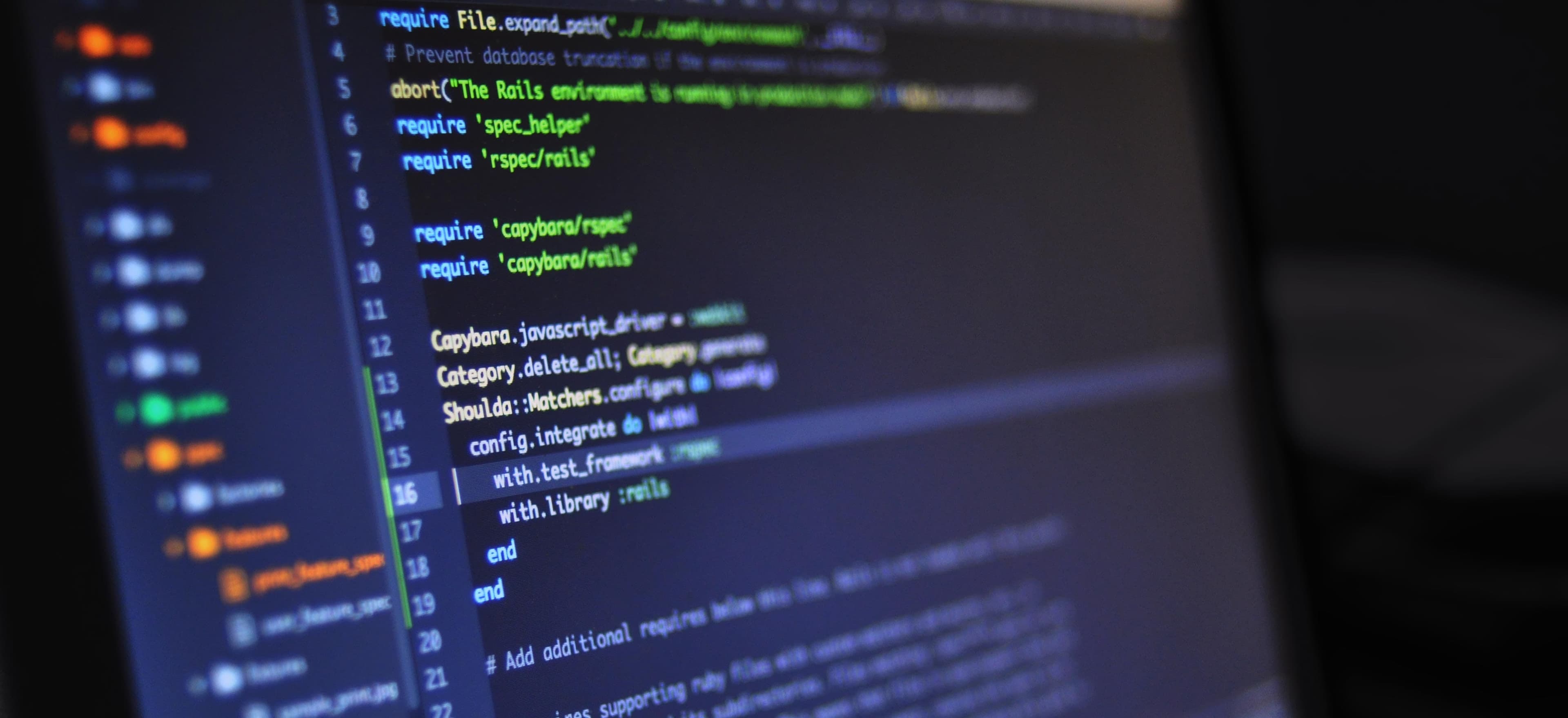
- Published on
Mastering MVVM Binding Challenges in ZK Framework
In the world of Java web development, the Model-View-ViewModel (MVVM) architecture has gained significant traction due to its ability to separate concerns and streamline the development process. When it comes to implementing MVVM in Java, ZK Framework stands out as a powerful tool for building enterprise web applications. However, like any framework, ZK presents its own set of challenges when it comes to MVVM binding. In this post, we will take a deep dive into some of these challenges and discuss strategies for mastering MVVM binding in ZK Framework.
Understanding MVVM and ZK Framework
Before delving into the challenges and solutions, it's important to have a solid grasp of MVVM and ZK Framework.
MVVM is an architectural pattern that facilitates a clear separation between the graphical user interface and the application logic. It consists of three key components:
- Model: Represents the data and business logic
- View: Represents the UI elements
- ViewModel: Acts as an intermediary between the Model and the View, exposing data and commands to the View
ZK Framework is a Java-based open-source web framework that allows developers to build highly interactive and responsive web applications. It provides a rich set of components and a powerful event-driven programming model, making it an ideal choice for enterprise-grade applications.
MVVM Binding Challenges in ZK
While MVVM offers numerous benefits, implementing MVVM binding in ZK Framework comes with its own set of challenges. Let's explore some of the common hurdles developers may encounter:
1. Property Binding
One of the fundamental aspects of MVVM is binding properties between the View and the ViewModel. In ZK, achieving seamless property binding can be challenging, especially when dealing with complex UI components and nested property structures.
2. Command Binding
Binding commands from the View to the ViewModel is crucial for handling user interactions and triggering application logic. ZK's event-driven model adds a layer of complexity to command binding, requiring careful attention to ensure proper communication between the View and the ViewModel.
3. Two-Way Data Binding
In MVVM, two-way data binding allows changes in the View to be automatically reflected in the ViewModel, and vice versa. ZK's rich and dynamic components make implementing two-way data binding a non-trivial task, particularly when dealing with input elements and live data synchronization.
4. Validation Binding
Validating user input and propagating validation feedback to the UI is an essential aspect of modern web applications. Integrating validation logic into the MVVM pattern in ZK requires a thorough understanding of the framework's validation mechanisms and their alignment with ViewModel properties.
Strategies for Mastering MVVM Binding in ZK
Now that we've identified the challenges, let's discuss strategies for mastering MVVM binding in ZK Framework. These approaches can help developers overcome the hurdles and leverage the full potential of MVVM in their web applications.
1. Leverage Data Binding Annotations
ZK Framework provides powerful data binding annotations that can simplify the process of binding properties and commands between the View and the ViewModel. Annotations such as @bind
and @command
play a key role in establishing the communication channels and reducing boilerplate code.
@bind(vm="myViewModel", save= "onSave")
private Textbox nameTextbox;
In this example, the @bind
annotation links the nameTextbox
component to the myViewModel
ViewModel, with the onSave
method triggered on save.
2. Utilize ZK Bind Composer
The ZK Bind Composer is a valuable tool for managing MVVM bindings in ZK applications. By extending the SelectorComposer
class and leveraging its lifecycle methods, developers can orchestrate the binding process, handle events, and manage data synchronization between the View and the ViewModel.
public class MyBindComposer extends SelectorComposer<Component> {
@Wire
private Textbox nameTextbox;
@Init
public void init() {
// Initialize ViewModel and bind properties
}
@Command
public void onSave() {
// Handle save command
}
}
By utilizing the ZK Bind Composer, developers can encapsulate MVVM binding logic in a structured and organized manner.
3. Implement Custom Converters and Validators
ZK Framework offers support for custom converters and validators, which can be seamlessly integrated into the MVVM binding process. By creating custom converter and validator classes and incorporating them into the binding annotations, developers can enforce data integrity and validation rules within the MVVM paradigm.
@Converter("customConverter")
public class CustomConverter implements FormatWidget{
// Implement conversion logic
}
By defining custom converters and validators, developers can tailor the data binding process to accommodate specific requirements and domain-specific validation rules.
Lessons Learned
Mastering MVVM binding challenges in ZK Framework is essential for building robust and maintainable web applications. By understanding the intricacies of MVVM binding in ZK and employing the strategies discussed in this post, developers can overcome the challenges and harness the power of MVVM to create sophisticated, responsive, and scalable web applications.
In conclusion, while MVVM binding in ZK Framework presents its own set of challenges, with the right knowledge and techniques, developers can navigate the complexities and unlock the full potential of MVVM in their web development endeavors.
In the end, it's about embracing the power of MVVM and leveraging the capabilities of ZK Framework to build extraordinary web applications that seamlessly integrate the Model, View, and ViewModel into a cohesive and efficient architecture.
By mastering MVVM binding challenges in ZK Framework, developers can elevate their web development skills and deliver exceptional user experiences through well-structured and maintainable codebases.
So, are you ready to take on the challenge of MVVM binding in ZK Framework and unlock a new level of web application development? The journey awaits, and the possibilities are limitless.
Happy coding!