Overcome Common Selenium Java Hurdles for Local Website Testing
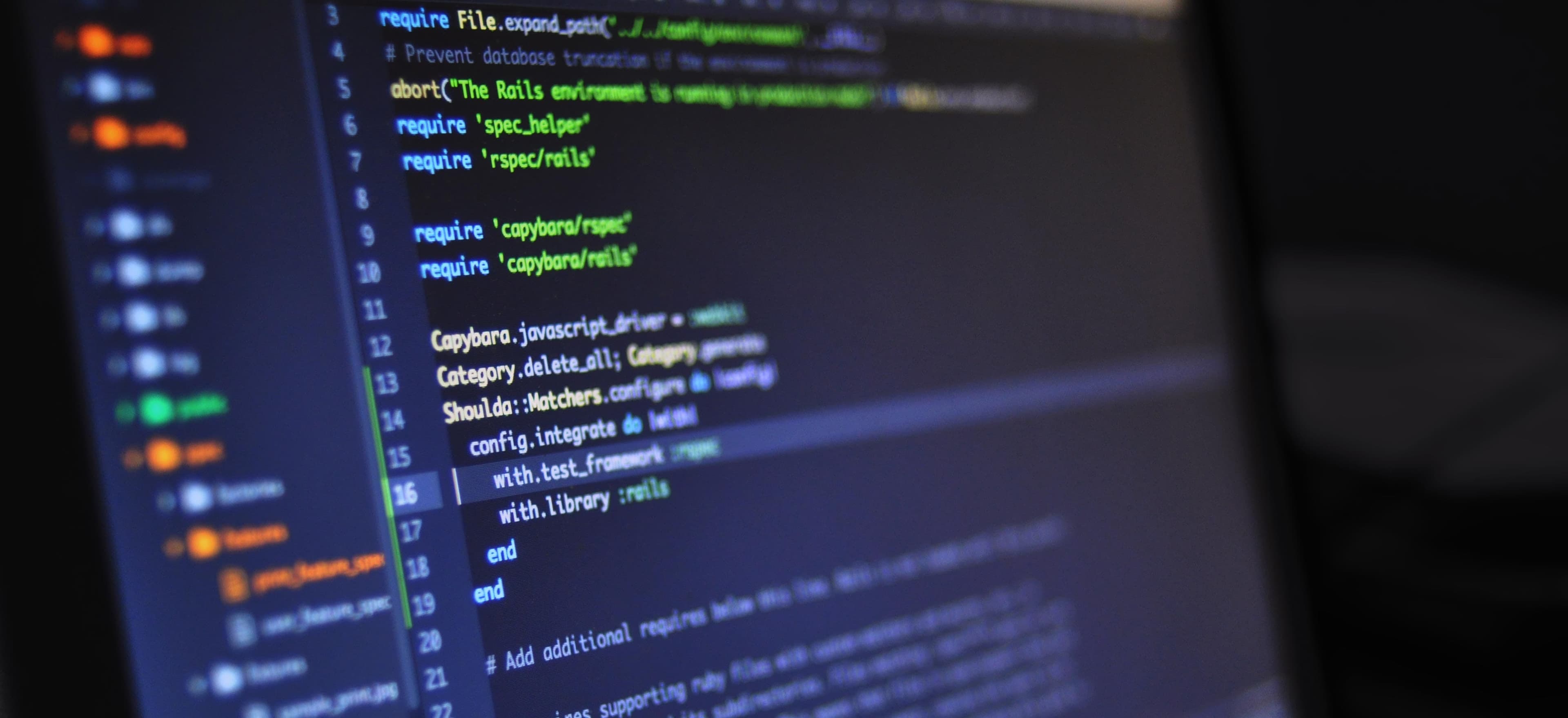
- Published on
Overcoming Common Selenium Java Hurdles for Local Website Testing
Testing is an essential part of the software development process, ensuring that the code meets requirements and functions as intended. For web applications, Selenium is a popular tool for automating browsers to carry out testing. Java, being a versatile and powerful language, is a natural fit for Selenium due to its robustness. However, using Selenium with Java for local website testing can present various challenges. In this article, we will explore some common hurdles encountered when using Selenium with Java for local website testing and how to overcome them.
Setting Up Selenium WebDriver
When beginning with Selenium testing in Java, the first challenge often involves setting up the Selenium WebDriver. The WebDriver is a vital component of Selenium that enables the interaction with web browsers. To overcome this hurdle, ensure that you have the correct Selenium WebDriver dependencies in your Java project. Here's an example using Maven, a popular Java build tool:
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version>
</dependency>
</dependencies>
Additionally, you need to download the browser-specific drivers such as ChromeDriver or GeckoDriver and set their paths in the system properties. This allows Selenium to interact with the respective browsers during testing.
Dealing with Asynchronous Behavior
Web applications frequently include asynchronous behavior through AJAX requests or timeouts. Handling these asynchronous operations is crucial for reliable testing. In Selenium with Java, the WebDriverWait
class can be utilized to wait for certain conditions to be met before proceeding further. For instance, waiting for an element to be clickable can be achieved as follows:
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("someElement")));
element.click();
By using WebDriverWait
, you can tackle the asynchronous nature of web applications and ensure that your tests remain resilient in the face of dynamic content loading.
Managing Dynamic Elements
Dynamic elements on web pages, such as those generated by JavaScript, often pose a challenge for Selenium testing. These elements may not be immediately available when the page loads, causing test failures. One approach to handle dynamic elements is by using explicit waits in Selenium with Java. By explicitly waiting for the element to be present in the DOM, you can avoid NoSuchElementException
errors.
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.presenceOfElementLocated(By.id("dynamicElement")));
Utilizing explicit waits ensures that your tests can effectively deal with dynamically generated elements, leading to more stable and reliable test automation.
Handling Authentication Pop-ups
Websites with authentication mechanisms often present a challenge for Selenium testing, particularly when dealing with authentication pop-ups. Using Selenium with Java, you can handle basic authentication pop-ups through the Alert
interface and passing the credentials directly in the URL. However, for more complex authentication scenarios, you may need to resort to browser automation tools like AutoIT or Robot class to handle operating system level authentication dialogs.
Dealing with Cross-Browser Testing
Ensuring that your web application functions correctly across different browsers is crucial. Selenium with Java makes cross-browser testing feasible, enabling you to validate your application's behavior on various browser platforms. By leveraging Selenium's WebDriver
interface in Java, you can write tests that are agnostic to the browser being used, thus facilitating cross-browser testing.
WebDriver driver = new ChromeDriver(); // For Chrome
WebDriver driver = new FirefoxDriver(); // For Firefox
By simply changing the instantiation of the WebDriver
object, you can switch the browser for testing, thus achieving cross-browser compatibility validation efficiently.
Testing on Mobile Devices
With the increasing prevalence of mobile web usage, it's essential to test your web application on mobile devices. Selenium with Java provides the capability to simulate mobile browsing through the use of emulators and simulators. By employing tools like Appium, you can extend your Selenium tests to cover mobile platforms as well, ensuring a comprehensive test coverage across different device categories.
The Last Word
Testing local websites using Selenium with Java comes with its set of challenges, ranging from setting up the appropriate dependencies to handling dynamic elements and diverse browser environments. By employing the strategies discussed in this article, you can overcome these hurdles and streamline your testing process, ultimately leading to more robust and reliable web applications. With the powerful combination of Selenium and Java, you can achieve comprehensive test automation for your web projects, delivering high-quality software with confidence.
In conclusion, mastering Selenium with Java for local website testing involves a combination of technical expertise, strategic test design, and thorough understanding of the web application's behavior. As you navigate the landscape of Selenium testing in Java, the ability to overcome common hurdles will undoubtedly elevate the effectiveness of your testing efforts, contributing to the overall success of your software projects.
Checkout our other articles