Troubleshooting Java-Cassandra Connection Woes: A Guide
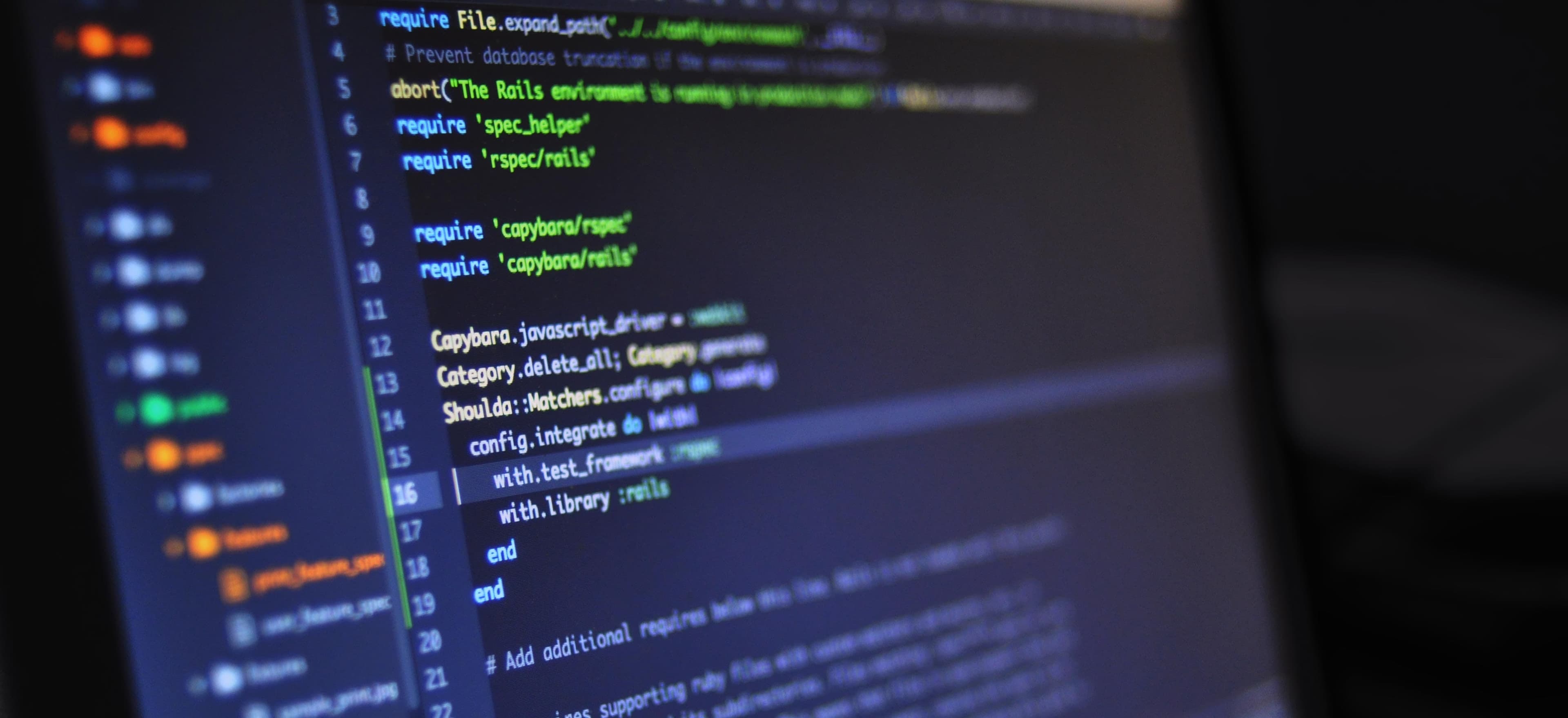
- Published on
Troubleshooting Java-Cassandra Connection Woes: A Guide
Are you encountering issues while trying to establish a connection between your Java application and Cassandra database? You're not alone. Setting up the connection between Java and Cassandra can occasionally be a challenging task. However, fear not, as this guide will walk you through some common problems and their solutions, ensuring a smooth and error-free connection. Let's dive in!
1. Check Dependency Management
Before delving into complex troubleshooting, let's start with the basics. Ensure that you have included the necessary dependencies in your Java project. For connecting to Cassandra, you will typically rely on the Datastax Java Driver. Here's a simplified example of how you would include the driver using Maven:
<dependencies>
<dependency>
<groupId>com.datastax.oss</groupId>
<artifactId>java-driver-core</artifactId>
<version>4.13.0</version>
</dependency>
</dependencies>
Remember to verify that your project includes the correct version of the Java driver. Sometimes, compatibility issues arise due to the usage of incompatible driver versions.
2. Connection Configuration
Once you have verified the presence of the necessary dependencies, the next step is to ensure that your connection configuration is accurate. One common pitfall is misconfiguring the contact points or using incorrect credentials. Double-check the host and port configuration, as well as any authentication details if required.
Here's an example of how you would set up a simple connection to a Cassandra cluster using the Datastax Java Driver:
CqlSession session = CqlSession.builder()
.addContactPoint(new InetSocketAddress("127.0.0.1", 9042))
.withLocalDatacenter("datacenter1")
.withAuthCredentials("username", "password")
.build();
One crucial aspect to note here is to replace "username" and "password" with your actual Cassandra database credentials. Also, ensure that the contact point and datacenter configuration match your Cassandra cluster setup.
3. Handling Connection Pooling
Efficient connection pooling is vital for maintaining a scalable and performant Java-Cassandra connection. Failing to configure an appropriate connection pool can result in performance bottlenecks and resource exhaustion.
The Datastax Java Driver provides a built-in connection pooling mechanism, which is efficiently managed by default. However, it's essential to understand and tune the connection pool settings based on your application's workload and Cassandra cluster configuration.
Here's an example of configuring the connection pool in the Datastax Java Driver:
CqlSession session = CqlSession.builder()
.withLocalDatacenter("datacenter1")
.addContactPoint(new InetSocketAddress("127.0.0.1", 9042))
.withAuthCredentials("username", "password")
.withKeyspace("your_keyspace")
.build();
Ensure that you replace "your_keyspace" with the actual keyspace you intend to work with. Additionally, review the connection pooling settings provided by the Java Driver documentation and adjust them according to your application's requirements.
4. Handling Timeouts and Retries
In a distributed environment such as Cassandra, network timeouts and query retries are common scenarios. Failing to handle these effectively can lead to unstable connections and unpredictable application behavior.
It's crucial to configure appropriate timeout settings and implement retry strategies in your Java application. The Datastax Java Driver offers comprehensive support for configuring timeouts and retries, allowing you to fine-tune these parameters based on your specific use case.
Let's look at an example of configuring timeouts and retries in the Datastax Java Driver:
CqlSession session = CqlSession.builder()
.withLocalDatacenter("datacenter1")
.addContactPoint(new InetSocketAddress("127.0.0.1", 9042))
.withAuthCredentials("username", "password")
.withKeyspace("your_keyspace")
.withSocketOptions(
new SocketOptions().setConnectTimeout(Duration.ofSeconds(5))
.setReadTimeout(Duration.ofSeconds(10)))
.build();
In this example, we've set the connect timeout to 5 seconds and the read timeout to 10 seconds. Adjust these values based on your network environment and Cassandra cluster responsiveness. Additionally, explore the retry policies offered by the Java Driver for handling query retries in case of failures.
Wrapping Up
In conclusion, establishing a robust and efficient connection between your Java application and Cassandra database requires careful consideration of dependency management, connection configuration, connection pooling, and handling timeouts and retries.
By following the guidelines and best practices outlined in this guide, you can troubleshoot and resolve common Java-Cassandra connection issues, ensuring a reliable and performant interaction between your Java application and Cassandra database.
Remember, as with any technology, continuous learning and exploration of best practices are crucial for mastering the art of Java-Cassandra connection management.
Now, armed with this knowledge, go forth and conquer the realm of Java-Cassandra connectivity with confidence!