Overcoming Slow Performance in Java Queue Operations
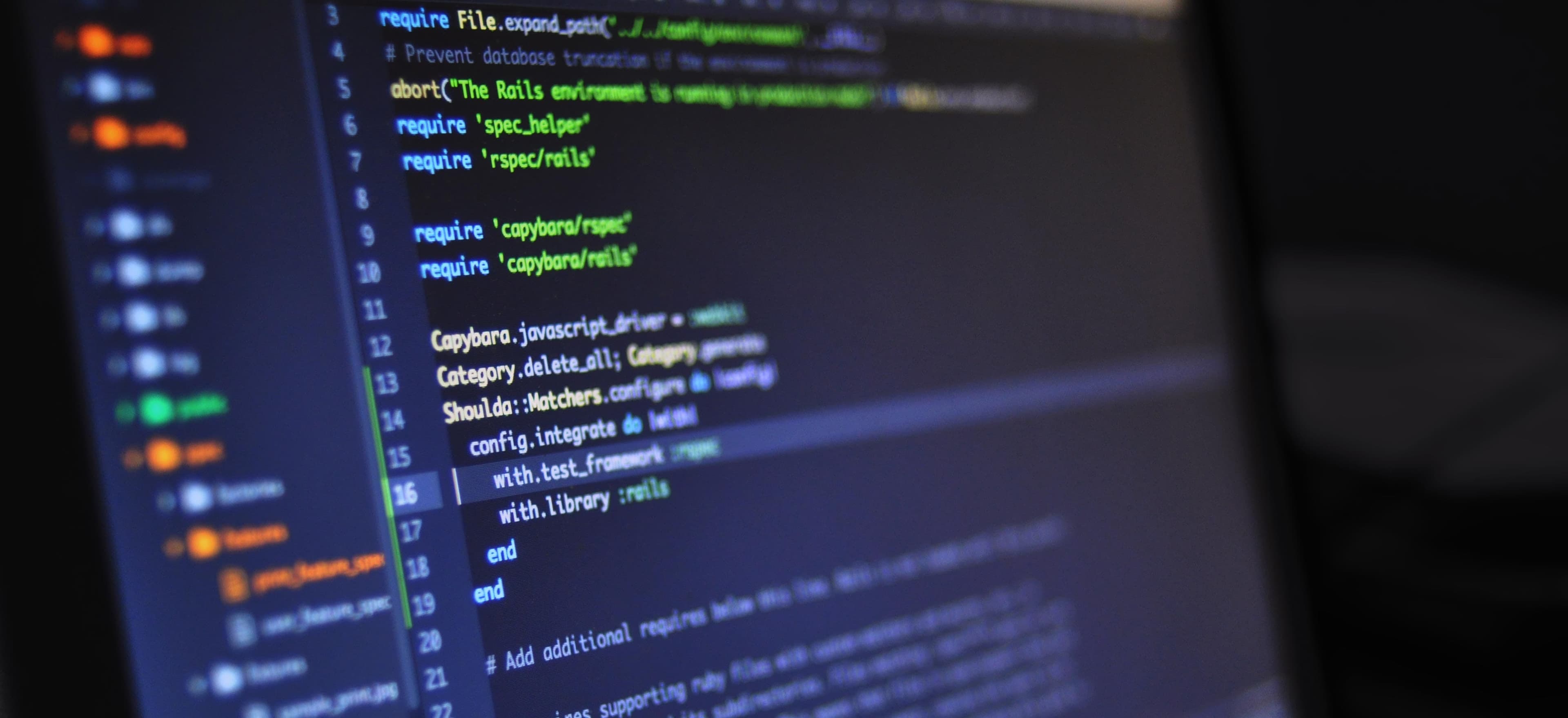
- Published on
Overcoming Slow Performance in Java Queue Operations
When it comes to Java programming, one of the fundamental data structures used is the queue. A queue is a collection used to hold elements prior to processing, with the FIFO (First In, First Out) principle governing its operations. While queues are powerful in managing data, inefficient implementation can lead to performance issues. In this blog post, we'll explore the reasons behind slow performance in Java queue operations and delve into strategies to overcome them.
Understanding the Slow Performance Issues
1. Inadequate Data Structures
The Java Collections Framework provides various queue implementations such as LinkedList, ArrayDeque, and PriorityQueue. Each of these implementations has different characteristics that can impact performance. For instance, using LinkedList as a queue may result in slower performance for operations that require accessing elements in the middle of the queue due to its sequential traversal nature.
2. Large Data Sets
When dealing with large data sets, the performance of queue operations can deteriorate. As elements are continuously added and removed from the queue, inefficiencies in the implementation can significantly impact the overall performance.
3. Inefficient Algorithm Design
The design of the algorithm utilizing the queue can also contribute to slow performance. Inefficient use of queue operations, such as unnecessary iterations or redundant element comparisons, can lead to performance bottlenecks.
Strategies to Improve Performance
1. Choose the Right Queue Implementation
Selecting the appropriate queue implementation based on the specific requirements of the application is crucial. For example, if the operation involves frequent additions and removals of elements, ArrayDeque often offers better performance than LinkedList due to its array-based implementation.
Queue<Integer> queue = new ArrayDeque<>();
2. Capacity Planning for Queues
In scenarios where the size of the queue is known beforehand, specifying the initial capacity can prevent frequent resizing of the underlying data structure, thus improving performance. This can be achieved using the appropriate constructor or method, such as the ArrayDeque(int initialCapacity)
constructor.
Queue<Integer> queue = new ArrayDeque<>(initialCapacity);
3. Efficient Algorithm Design
Optimizing the algorithm using the queue can significantly enhance performance. For instance, employing efficient data structures in conjunction with the queue, such as HashSet or HashMap for constant time lookups, can streamline operations and mitigate performance issues.
Queue<Integer> queue = new LinkedList<>();
Set<Integer> set = new HashSet<>();
// Efficient algorithm design
while (!queue.isEmpty()) {
int element = queue.poll();
if (!set.contains(element)) {
set.add(element);
// Process the element
}
}
4. Threading and Concurrency Considerations
In multi-threaded applications, usage of thread-safe queue implementations, such as ConcurrentLinkedQueue, can prevent contention and ensure efficient performance by allowing multiple threads to access the queue concurrently without the need for external synchronization.
Queue<Integer> concurrentQueue = new ConcurrentLinkedQueue<>();
5. Profiling and Performance Tuning
Utilizing profiling tools such as YourKit, JProfiler, or VisualVM can help identify performance bottlenecks in queue operations. By pinpointing areas of inefficiency, developers can fine-tune their code and data structures for optimal performance.
To Wrap Things Up
In conclusion, while Java queue operations are fundamental to many applications, inefficient implementation can lead to slow performance. By understanding the underlying causes of slow performance and adopting appropriate strategies such as choosing the right queue implementation, efficient algorithm design, capacity planning, and leveraging profiling tools, developers can significantly improve the performance of queue operations in their Java applications. With these strategies in place, developers can ensure that their queue operations perform optimally, even when dealing with large data sets and demanding workloads.
By addressing these performance issues and implementing best practices, developers can build efficient and responsive applications that leverage the power of Java's queue data structures effectively.
Take your Java queue operations to the next level by optimizing performance and ensuring efficient data processing.
Remember, understanding the 'why' behind the code and the performance strategies is crucial to writing efficient and effective Java programs.
Checkout our other articles