Visualizing World Rain Patterns: HTML5 Canvas & Open Data
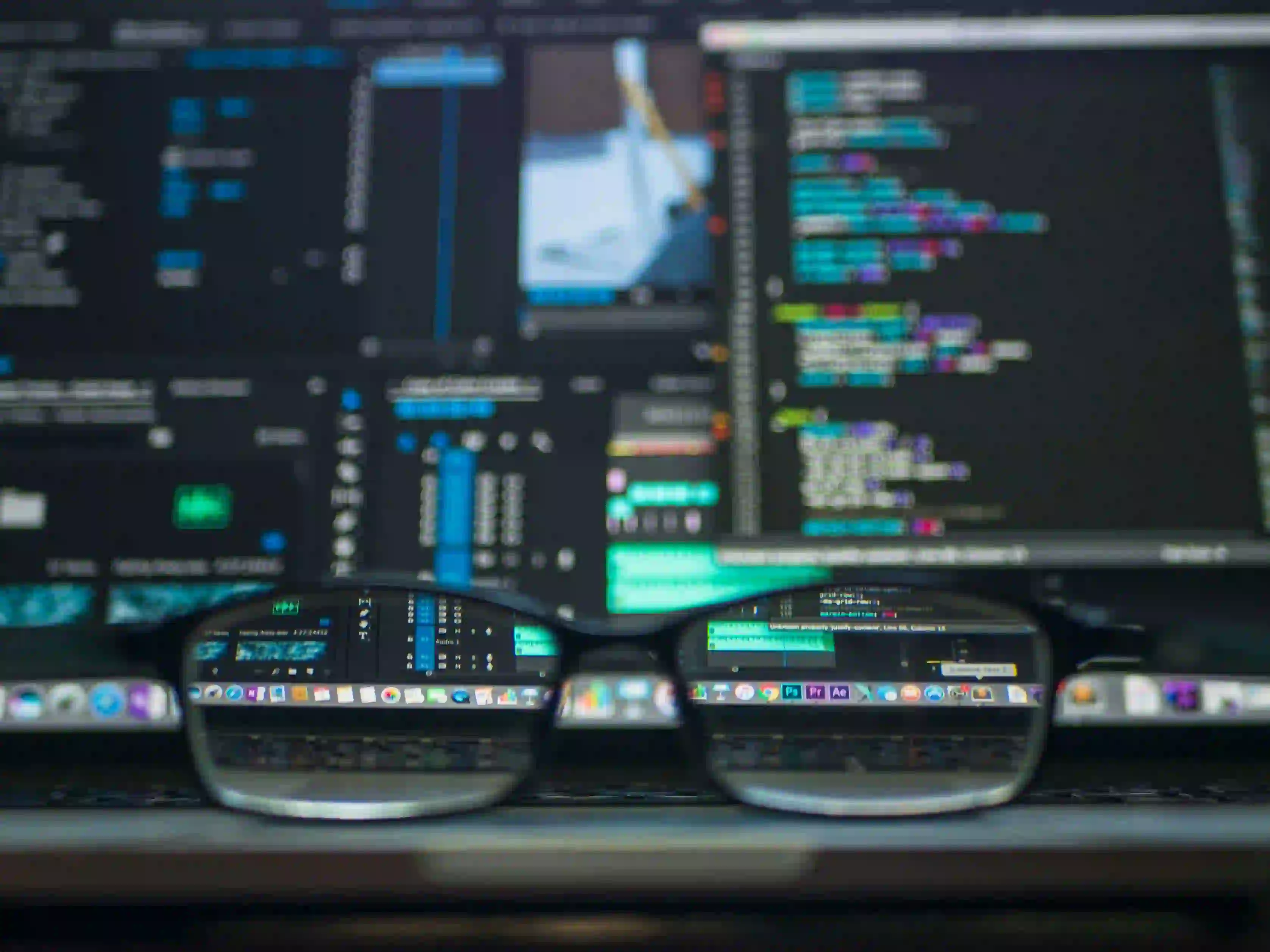
Visualizing World Rain Patterns with HTML5 Canvas & Open Data
Have you ever wondered about the global distribution of rainfall patterns? Rainfall is a critical component of the Earth's climate system, shaping ecosystems and influencing agricultural productivity. In this tutorial, we will leverage HTML5 Canvas and open data to create an interactive visualization of world rain patterns.
The Opening Bytes to HTML5 Canvas
HTML5 Canvas provides a powerful and flexible way to render graphics and visualizations within web browsers. Its ability to dynamically draw shapes, text, and images makes it an ideal tool for creating interactive visualizations.
Open Data for Rain Patterns
To create our visualization, we will utilize open data sources such as the Global Precipitation Climatology Centre (GPCC) dataset. This dataset provides global monthly precipitation data at a resolution of 1° x 1° latitude/longitude grid. The GPCC dataset is available for free and can be accessed through the CDO API or downloaded directly.
Setting Up the HTML and JavaScript Environment
Let's start by setting up the HTML structure and including the necessary JavaScript libraries. We will use Bootstrap for responsive layout and d3.js for data manipulation and scaling.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>World Rain Patterns Visualization</title>
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" rel="stylesheet">
<style>
/* Add custom CSS styles here */
</style>
</head>
<body>
<div class="container">
<h1 class="text-center my-4">World Rain Patterns</h1>
<div id="visualization"></div>
</div>
<script src="https://d3js.org/d3.v6.min.js"></script>
<script src="app.js"></script>
</body>
</html>
In this HTML structure, we include the Bootstrap CSS for responsive layout, create a container for our visualization, and include the d3.js library along with our custom JavaScript file app.js
.
Creating the Visualization with HTML5 Canvas and d3.js
Now, let's jump into the app.js
file and begin creating our rain pattern visualization.
We will start by defining the dimensions of the canvas and setting up the necessary variables.
// Define the canvas dimensions
const width = 800;
const height = 400;
// Append the canvas to the visualization div
const canvas = d3.select('#visualization')
.append('canvas')
.attr('width', width)
.attr('height', height);
// Get the 2D context for the canvas
const context = canvas.node().getContext('2d');
In the above code, we use d3.js to append a canvas
element to the visualization
div and obtain the 2D drawing context for the canvas.
Next, we'll fetch the GPCC rainfall data and use it to render the visualization on the canvas.
// Fetch the GPCC rainfall data (example using fetch API)
fetch('https://api.example.com/gpcc-data')
.then(response => response.json())
.then(data => {
// Process and render the data on the canvas
renderRainPatterns(data);
});
// Function to render the rain patterns on the canvas
function renderRainPatterns(data) {
// Code for rendering rain patterns goes here
// Use the GPCC data to draw the rain patterns on the canvas
}
In the renderRainPatterns
function, we will utilize the GPCC rainfall data to draw the world rain patterns on the canvas. This involves mapping the data to the canvas coordinates and using appropriate visual encoding to represent the rainfall intensity.
Mapping Data to Canvas Coordinates
Mapping the GPCC rainfall data to canvas coordinates involves scaling the latitude and longitude values to fit the canvas dimensions. This also requires handling the translation of spherical coordinates to flat Cartesian coordinates.
// Define the projection function to map lat/long to x/y coordinates
function project(x, y) {
const lambda = x * Math.PI / 180;
const phi = y * Math.PI / 180;
const xCoord = (lambda + Math.PI) * (width / (2 * Math.PI));
const yCoord = (Math.PI - Math.log(Math.tan(Math.PI / 4 + phi / 2))) * (height / (2 * Math.PI));
return [xCoord, yCoord];
}
In the project
function, we convert the latitude and longitude values to Cartesian coordinates using a projection formula. This enables us to accurately position the rain patterns on the canvas.
Drawing Rain Patterns on the Canvas
After mapping the data to canvas coordinates, we can now draw the rain patterns based on the rainfall intensity. We'll use color encoding to represent varying levels of rainfall.
// Function to render the rain patterns on the canvas
function renderRainPatterns(data) {
data.forEach(d => {
const [x, y] = project(d.longitude, d.latitude);
const intensity = d.rainfallIntensity;
// Set the fill color based on rainfall intensity
if (intensity < 50) {
context.fillStyle = 'lightblue';
} else if (intensity < 100) {
context.fillStyle = 'blue';
} else {
context.fillStyle = 'darkblue';
}
// Draw a circle at the projected coordinates
context.beginPath();
context.arc(x, y, 5, 0, 2 * Math.PI);
context.fill();
});
}
In the renderRainPatterns
function, we iterate through the GPCC data, project the coordinates, and draw circular shapes on the canvas. We dynamically set the fill color based on the rainfall intensity, providing a visual representation of rain patterns across the world.
Creating Interactive Features
To enhance the visualization, we can add interactive features such as tooltips to display detailed information when the user interacts with the rain patterns.
// Function to handle mouse hover for tooltip display
canvas.on('mousemove', function(event) {
const [x, y] = d3.pointer(event);
// Code to display tooltip based on canvas coordinates
});
The mouse hover event allows us to track the user's interaction with the rain patterns and display relevant information using tooltips.
Closing Remarks
In this tutorial, we've explored how to utilize HTML5 Canvas and open data to create an interactive visualization of world rain patterns. By leveraging d3.js for data manipulation and canvas drawing, we've successfully visualized global rainfall patterns in a dynamic and engaging manner.
Visualizing rain patterns is just one application of HTML5 Canvas and open data. You can further extend this approach to visualize various geospatial datasets and create compelling interactive visualizations for diverse domains.
Start exploring rain patterns and unleash the potential of HTML5 Canvas for visual storytelling!