101 Ways to Enhance Java: Insights from JavaOne 2012
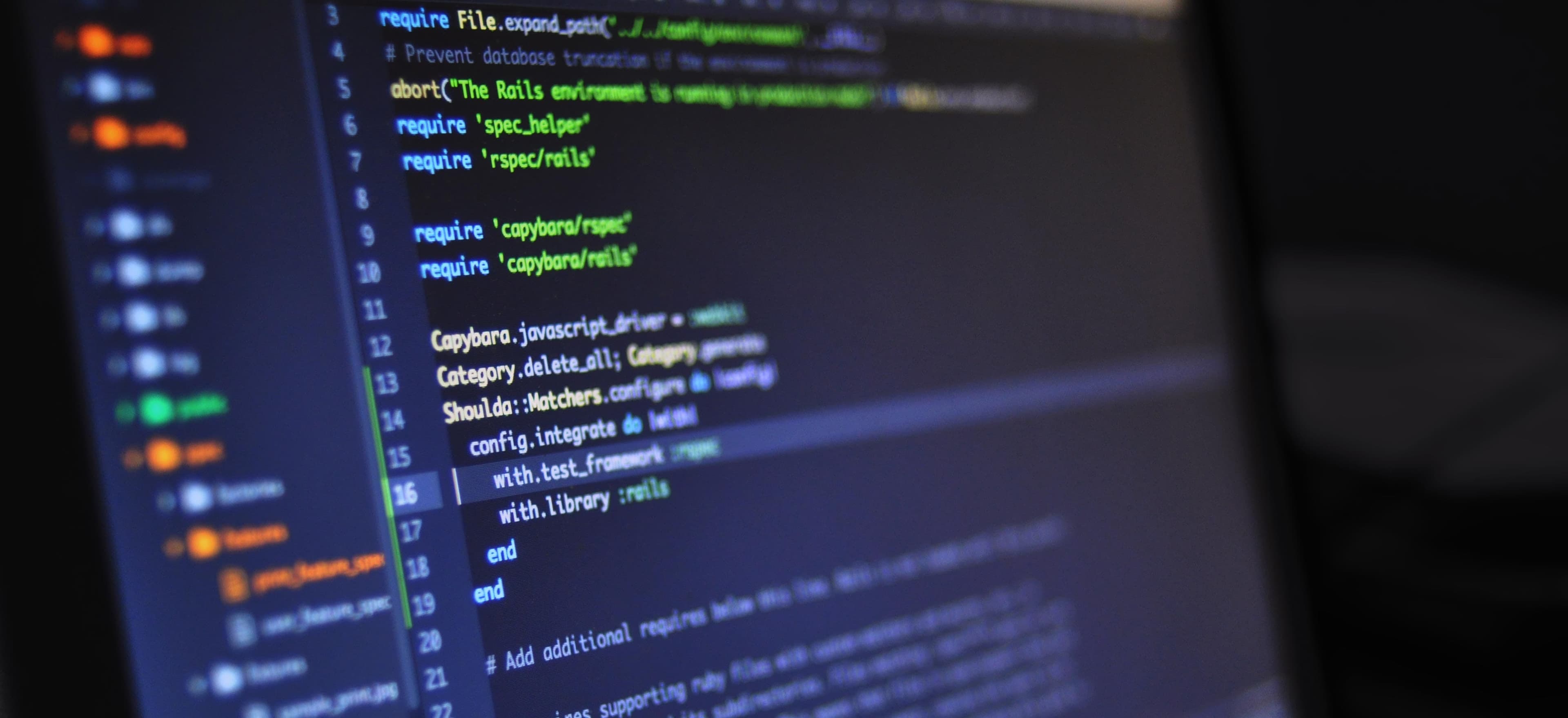
- Published on
101 Ways to Enhance Java: Insights from JavaOne 2012
Java has been a cornerstone of the programming world for decades, and it continues to evolve and improve. JavaOne 2012, one of the most prominent events in the Java community, showcased numerous ways to enhance Java and take advantage of its capabilities. This article captures key insights from the event, offering 101 actionable tips to boost your Java prowess.
1. Embrace Java 8 Lambdas
Java 8 introduced lambdas, which revolutionized the way we write code. Applying lambdas can make your code more concise and readable. Here's an example:
List<String> names = Arrays.asList("John", "Sarah", "Michael");
names.forEach(name -> System.out.println("Hello, " + name));
The use of lambdas here simplifies the iteration over the list, making the code more expressive.
2. Leverage Functional Interfaces
Functional interfaces are vital for working with lambdas. By embracing functional interfaces, you can succinctly express single abstract methods, making your code more flexible and reusable. For instance, the java.util.function
package provides a variety of predefined functional interfaces like Predicate
, Consumer
, and Supplier
that you can exploit in your everyday coding tasks.
3. Explore the Stream API
The Stream API, also introduced in Java 8, offers a fluent and declarative approach to processing collections. Utilizing streams enables you to write concise, elegant, and high-performing code for operations like filtering, mapping, and reducing.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream().reduce(0, Integer::sum);
System.out.println("Sum: " + sum);
By using streams, you can easily perform complex operations on collections in a more functional style.
4. Embrace Optional
To alleviate the pitfalls of dealing with null values, Java 8 introduced the Optional
class. Incorporating Optional
in your code promotes better handling of potentially absent values, reducing the likelihood of null pointer exceptions and making your code more robust.
Optional<String> name = Optional.ofNullable(getName());
name.ifPresent(System.out::println);
5. Use CompletableFuture for Asynchronous Operations
Asynchronous programming is crucial for building responsive and efficient applications. Java 8's CompletableFuture
provides a powerful way to work with asynchronous tasks. By employing CompletableFuture
, you can easily create, combine, and compose asynchronous operations, enabling you to write non-blocking code more effectively.
6. Embrace Modularization with Java Platform Module System (JPMS)
With the introduction of JPMS in Java 9, you can modularize your codebase, leading to increased maintainability and encapsulation. Modularization via JPMS allows for better organization of code, improved encapsulation, and minimized classpath issues.
7. Exploit the New HTTP Client
Java 11 introduced a new HTTP client, which offers a more modern and efficient way to perform HTTP requests and handle responses. By utilizing the new HTTP client, you can benefit from features such as non-blocking communication, support for WebSockets, and more flexible request building.
8. Enhance Productivity with JShell
Java 9 introduced JShell, a read-eval-print loop (REPL) tool that enables interactive and exploratory programming. Leveraging JShell facilitates rapid prototyping, testing small code snippets, and experimenting with language features without the need for writing full-fledged applications or classes.
9. Embrace Pattern Matching (JEP 305)
Pattern matching, a feature expected to be fully realized in future versions, allows concise and safe extraction of components from objects. With pattern matching, you can streamline code, enhance readability, and reduce the verbosity of certain operations.
10. Upgrade with Records (JEP 359)
Records, introduced as a preview feature in Java 14, provide a compact way to declare classes to model immutable data. By using records, you can concisely define data-centric classes with less boilerplate code, leading to enhanced code clarity and maintainability.
Final Thoughts
JavaOne 2012 offered a plethora of insights and strategies to enhance Java programming. By embracing the aforementioned tips and keeping abreast of the latest Java advancements, you can elevate your proficiency in Java development and contribute to the evolution of this ever-dynamic language.
Incorporating these enhancements can significantly augment your Java development skills, empowering you to build more robust, concise, and efficient applications. Stay tuned for more exciting updates and innovative features as Java continues to advance and flourish.
Remember, mastering Java is a continuous journey, and JavaOne 2012 was just the tip of the iceberg. Keep exploring, experimenting, and honing your Java skills to stay ahead in the ever-evolving world of programming.
Java, the Duke logo, and JavaOne are trademarks of Oracle Corporation.
Checkout our other articles