Unlocking Java 8: Mastering Lambdas for Better Code
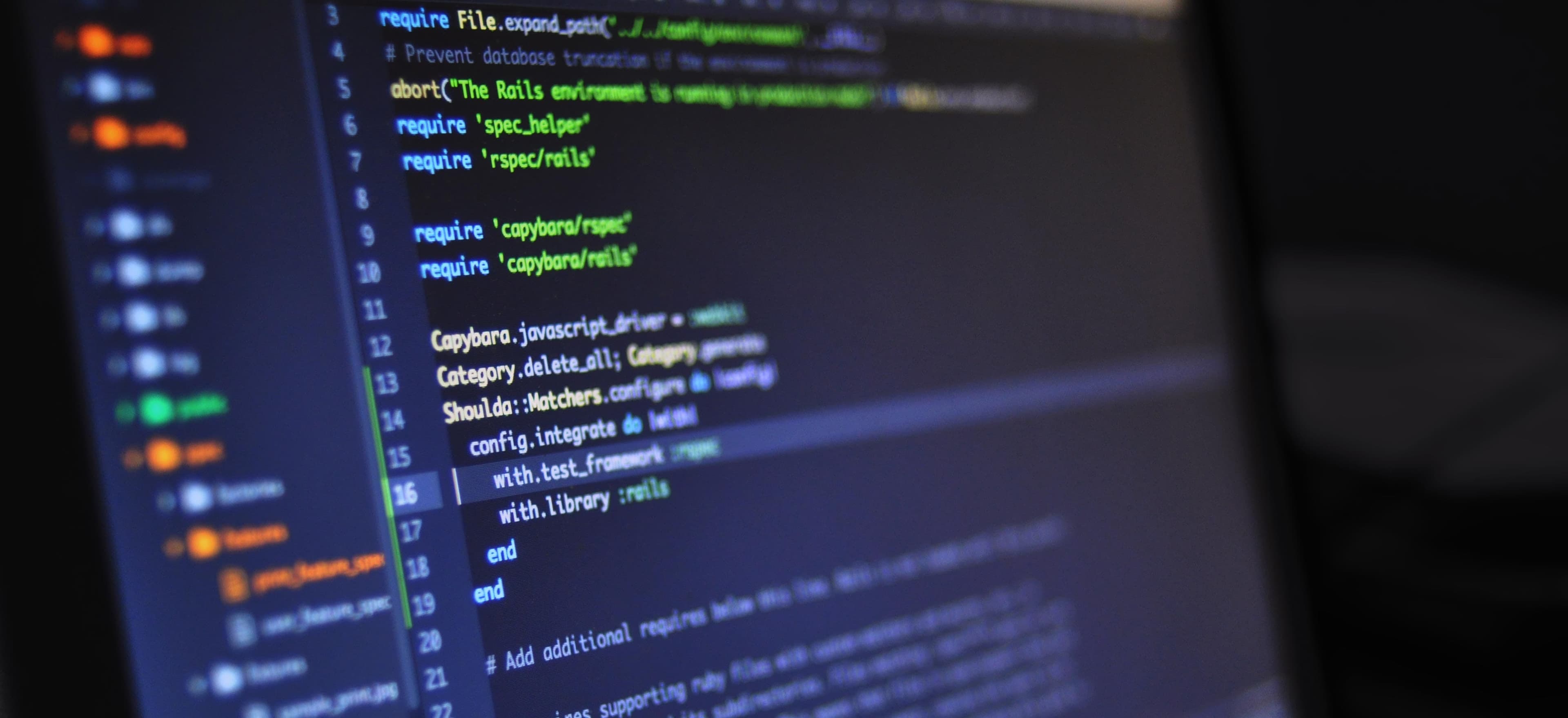
- Published on
Unlocking Java 8: Mastering Lambdas for Better Code
When it comes to writing clean, concise, and efficient code in Java, embracing the power of lambdas introduced in Java 8 is crucial. Lambdas are a core feature of functional programming that allows developers to write smaller, more readable code for tasks that can be represented as a single method interface. In this article, we will delve into the world of lambdas, exploring their syntax, benefits, and best practices for leveraging them to write better Java code.
Understanding Lambdas
At its core, a lambda expression is a block of code that represents an instance of a functional interface. A functional interface is an interface that contains exactly one abstract method. The syntax of a lambda expression consists of parameters, an arrow (->), and a body. Consider the following example:
Comparator<String> comparator = (String s1, String s2) -> s1.compareTo(s2);
In this example, we define a lambda expression that represents an instance of the Comparator
functional interface. The parameters (String s1, String s2)
are followed by the arrow ->
, and the body s1.compareTo(s2)
provides the implementation of the abstract method compare
.
Benefits of Using Lambdas
Concise and Readable Code
One of the key benefits of using lambdas is the ability to write more concise and readable code. Instead of defining an entire anonymous class or interface implementation, lambdas allow developers to express functionality directly at the point where it's needed. This results in code that is easier to understand and maintain.
Improved API Flexibility
Lambdas enable the use of functional programming techniques, which can lead to more flexible and composable APIs. By treating behavior as a first-class citizen, lambdas allow for the creation of higher-order functions and facilitate the passing of behavior as arguments to methods.
Enhanced Parallelism and Concurrency
With the advent of functional interfaces like java.util.function.Function
and java.util.function.Consumer
, lambdas play a crucial role in enhancing parallelism and concurrency in Java. They provide a more streamlined way to express operations that can be parallelized or executed asynchronously.
Practical Use Cases
Sorting with Lambdas
Lambdas are particularly useful when sorting collections. Traditionally, sorting involved implementing a custom Comparator
or Comparable
interface, which often led to verbose and redundant code. With lambdas, sorting becomes more intuitive and less cumbersome.
Consider the following example:
List<String> names = Arrays.asList("Alice", "Bob", "Carol", "David");
// Prior to Java 8
Collections.sort(names, new Comparator<String>() {
public int compare(String s1, String s2) {
return s1.compareTo(s2);
}
});
// Using lambdas in Java 8
Collections.sort(names, (s1, s2) -> s1.compareTo(s2));
The lambda expression simplifies the sorting process, making the code more succinct and easier to comprehend.
Event Handling
Lambdas are also valuable for event handling, especially in graphical user interface (GUI) applications. Instead of implementing lengthy and cumbersome anonymous classes for event listeners, lambdas provide a more elegant solution for defining event handling logic.
button.setOnAction(event -> {
System.out.println("Button clicked");
});
In this example, the lambda expression directly specifies the action to be taken when the button is clicked, offering a more streamlined and readable alternative to traditional event handling mechanisms.
Best Practices and Considerations
Maintain Readability
While lambdas can significantly improve code readability, it's important to ensure that the lambda expressions remain clear and focused. Avoid writing overly complex lambdas that obscure the intent of the code.
Be Mindful of Capturing Variables
When using lambdas, be mindful of capturing variables from the enclosing scope. Variables used within a lambda expression should either be effectively final or explicitly marked as final
to prevent unintended side effects.
Prefer Standard Functional Interfaces
Java 8 introduced a set of standard functional interfaces in the java.util.function
package, such as Predicate
, Function
, and Consumer
. Leveraging these interfaces promotes code consistency and makes it easier for other developers to understand and maintain the codebase.
Use Method References When Appropriate
In cases where a lambda expression simply calls an existing method, consider using method references for improved clarity. Method references provide a shorthand notation for invoking methods and can enhance code readability.
A Final Look
In the realm of modern Java development, mastering lambdas is essential for writing clean, expressive, and efficient code. By understanding the syntax, benefits, and best practices of lambdas, developers can unlock the full potential of functional programming in Java 8 and beyond. Embracing lambdas empowers developers to create code that is more maintainable, flexible, and scalable, ultimately leading to better software quality and developer productivity.
As you venture further into the world of Java 8 and lambdas, consider exploring additional resources such as Oracle's official documentation and the book "Java 8 in Action" by Raoul-Gabriel Urma, Mario Fusco, and Alan Mycroft to deepen your understanding and expertise in leveraging lambdas for better code.
Happy coding!