Solving Java's Diamond Problem with Delegation
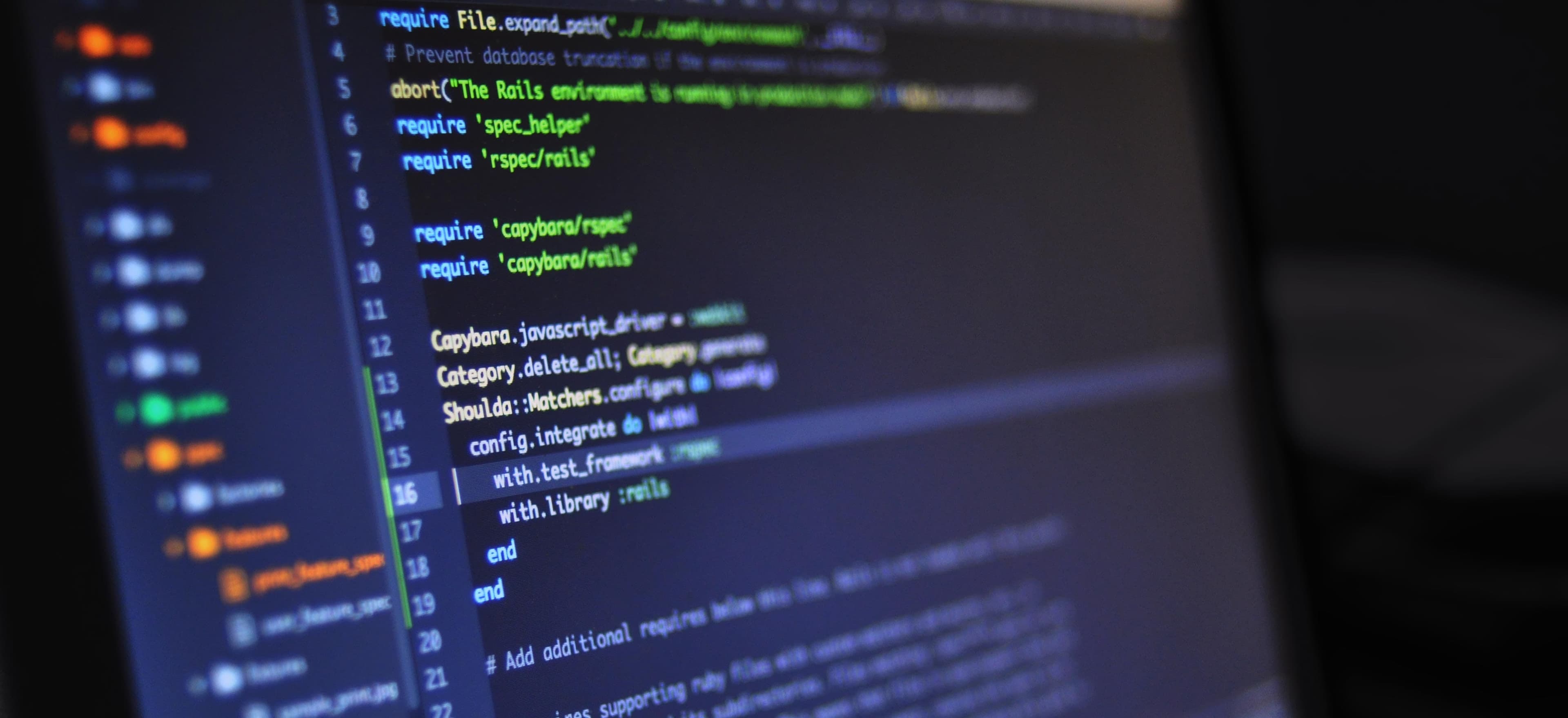
- Published on
Solving Java's Diamond Problem with Delegation
The "diamond problem" is a well-known issue in object-oriented programming languages with multiple inheritance, where a base class is inherited by two distinct subclasses, which then both inherit from a shared superclass. Java elegantly avoids this problem through its use of single inheritance, but there are instances where the diamond problem can still arise through interface inheritance.
In Java, a class can implement multiple interfaces, some of which may have methods with the same signature. This can lead to a scenario analogous to the diamond problem. Fortunately, Java offers the means to resolve this through a concept known as "delegation."
Understanding the Diamond Problem
Consider the following scenario: We have an interface A
with a method void doSomething()
, and two interfaces B
and C
, both of which extend A
. Additionally, we have a class D
which implements both B
and C
. If B
and C
both have a default implementation of doSomething()
, a conflict arises for class D
.
The Traditional Approach: Default Methods
Prior to Java 8, there was no elegant solution to this problem. With the introduction of default methods in interfaces, Java attempted to address this issue by allowing interfaces to provide a default implementation for methods. However, this solution could not resolve the conflict between multiple default implementations inherited through different paths.
This is where delegation comes to the rescue.
The Delegation Approach
Delegation is a design pattern where an object handles a request by delegating it to a helper object. In the context of Java, we can use delegation to explicitly choose which default method implementation to use. One common way to do this is to create a separate class that implements the conflicting method, and then let the implementing class delegate the method call to an instance of that class.
interface A {
default void doSomething() {
System.out.println("Default implementation from A");
}
}
interface B extends A {
default void doSomething() {
System.out.println("Default implementation from B");
}
}
interface C extends A {
default void doSomething() {
System.out.println("Default implementation from C");
}
}
class Delegator implements B, C {
private A delegate = new A() {
public void doSomething() {
B.super.doSomething(); // Explicitly choose B's implementation
}
};
@Override
public void doSomething() {
delegate.doSomething();
}
}
In the above example, the Delegator
class implements both interfaces B
and C
, but the conflict is resolved by explicitly choosing the implementation from B
using delegation. This allows for a clear resolution of the diamond problem scenario.
The 'Why' Behind Delegation
The use of delegation in this context solves the diamond problem by providing a clear and explicit way to select which interface's method implementation to use. By delegating the method call to a specific implementation, we eliminate the ambiguity and conflict that arises from multiple inheritance of default methods in Java interfaces.
Delegation is also beneficial from a design perspective, as it allows for better separation of concerns and maintainability in the codebase. It provides a clear delineation of responsibilities between classes and interfaces, making the code more readable and easier to maintain.
The Last Word
In conclusion, while Java's single inheritance model prevents the traditional diamond problem, similar issues can still arise through interface inheritance. By leveraging the concept of delegation, we can elegantly resolve conflicts that stem from multiple inheritance of default methods in interfaces.
Delegation provides a clear and explicit way to select the desired method implementation, effectively mitigating the diamond problem in Java. This approach not only resolves the conflict but also promotes clean, maintainable, and readable code.
So, the next time you encounter the diamond problem in Java, consider using the power of delegation to pave a clear path forward.
For further reading on Java's interface inheritance and delegation, check out the official Java documentation on interfaces and delegation.