Boost Performance: Going Off-Heap with Java ChronicleMap
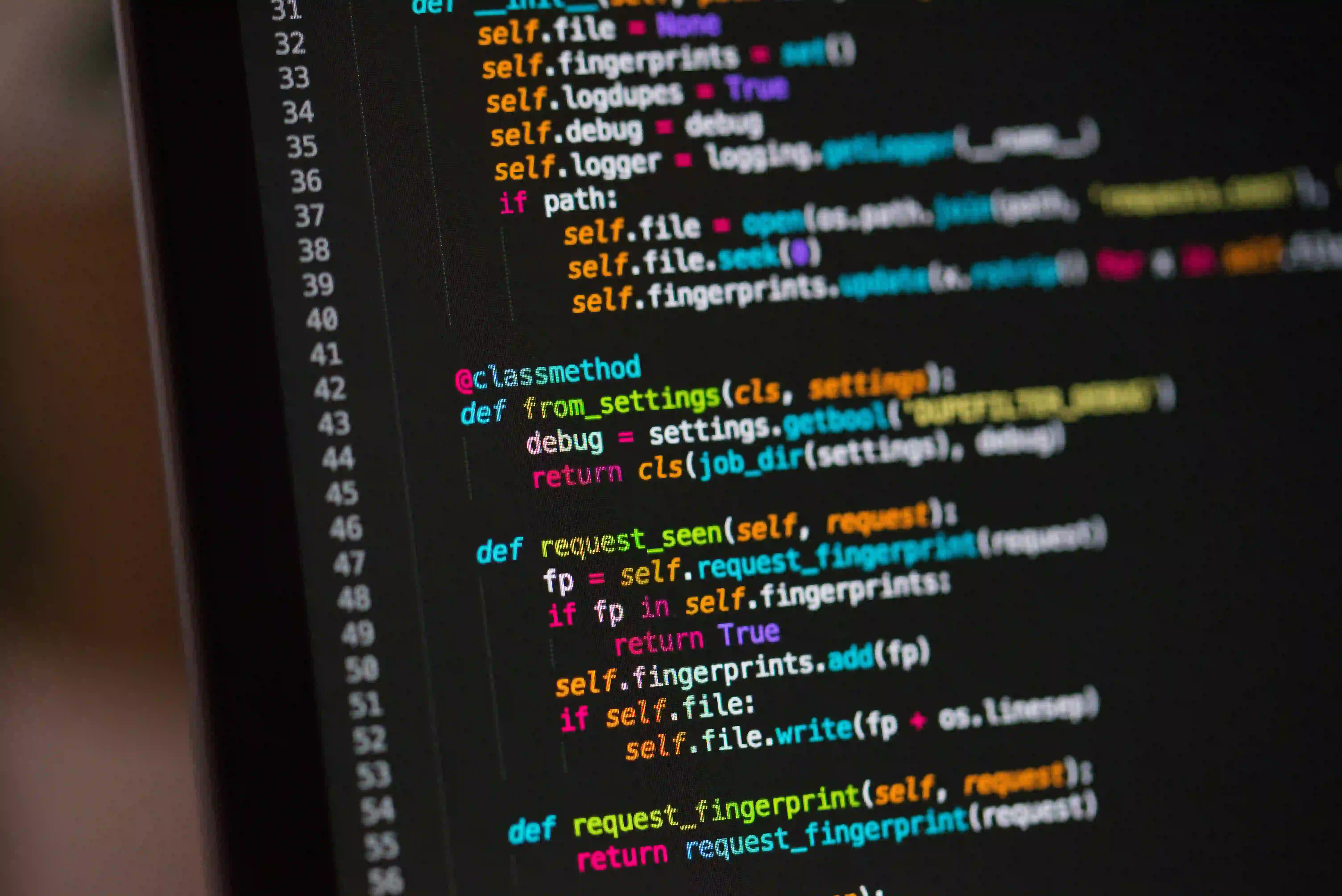
Boost Performance: Going Off-Heap with Java ChronicleMap
In the world of Java, performance optimizations are crucial, and one area of focus is memory management. Traditional Java data structures reside in the heap, which can result in performance bottlenecks due to garbage collection pauses. Off-heap memory, on the other hand, offers benefits such as consistent access times and reduced garbage collection overhead. In this blog post, we will explore the use of Java ChronicleMap to leverage off-heap memory for improved performance.
Understanding Off-Heap Memory
In Java, the heap is the memory where all objects are allocated. During garbage collection, the JVM pauses program execution to free up memory by reclaiming unreachable objects. This process, while necessary, can introduce latency in applications, particularly those that manage large amounts of data.
Off-heap memory, as the name suggests, refers to memory that is not managed by the JVM heap. By utilizing off-heap memory, we can bypass the limitations and overhead associated with garbage collection. This can lead to more predictable and consistent performance, especially for applications with stringent latency requirements.
Introducing Java ChronicleMap
Java ChronicleMap is a high-performance off-heap key-value store that is part of the Chronicle library. It provides a memory-mapped data structure, allowing direct memory access without incurring the overhead of garbage collection. This makes it an ideal choice for applications that demand low-latency data access with minimal pauses.
Setting Up ChronicleMap
Let's begin by including the ChronicleMap dependency in our project. We can achieve this by adding the following Maven dependency to our pom.xml
file:
<dependency>
<groupId>net.openhft</groupId>
<artifactId>chronicle-map</artifactId>
<version>3.19.39</version>
</dependency>
Once the dependency is added, we can start using ChronicleMap to harness the benefits of off-heap memory.
Creating a ChronicleMap Instance
To create a ChronicleMap instance, we need to decide on the data types for our keys and values. For example, if we want to create a map with String
keys and Integer
values, we can do so as follows:
ChronicleMap<String, Integer> offHeapMap = ChronicleMap
.of(String.class, Integer.class)
.averageValueSize(4) // Set the average size of the values
.entries(10_000) // Set the maximum number of entries
.createPersistedTo(new File("offheap.dat")); // Persist the map to a file
In this example, we create a ChronicleMap instance that maps String
keys to Integer
values. We specify the average value size and the maximum number of entries, and persist the map to a file (offheap.dat
). This enables the map to be reloaded from the file without needing to rebuild it, offering persistence across application restarts.
Usage of ChronicleMap
With our ChronicleMap instance created, we can now use it to store and retrieve key-value pairs. The usage is akin to a regular Map
instance in Java:
offHeapMap.put("key1", 100);
int value = offHeapMap.get("key1");
By storing data off-heap, we can potentially reduce memory pressure on the JVM heap, leading to more efficient garbage collection and overall improved performance.
Benefits of Off-Heap Memory with ChronicleMap
Reduced Garbage Collection Overhead
By utilizing off-heap memory with ChronicleMap, we can significantly reduce the impact of garbage collection on our application. This is especially beneficial for long-running services or applications that handle large datasets, as it minimizes pauses caused by garbage collection cycles.
Predictable Latency
Since off-heap memory does not suffer from the non-deterministic nature of garbage collection pauses, applications leveraging ChronicleMap can achieve more predictable and consistent latency. This is essential for real-time and low-latency use cases where response times must be tightly controlled.
Enhanced Scalability
Off-heap memory allows applications to scale more effectively, particularly when dealing with large volumes of data. By minimizing the impact of garbage collection, ChronicleMap can support higher throughput and better utilization of system resources, leading to improved scalability.
Additional Considerations
Memory Management
While off-heap memory offers performance benefits, it comes with the responsibility of explicitly managing memory. Developers must ensure proper allocation and deallocation of memory, as well as handle potential memory leaks. It's crucial to carefully manage off-heap memory to avoid adverse effects on system stability and performance.
Persistence and Durability
ChronicleMap supports persistence to files, enabling data to survive application restarts. However, developers must consider the trade-offs between durability and performance, as persistent operations can introduce additional latency. Careful consideration should be given to balancing persistence requirements with performance needs.
Object Serialization
When working with off-heap memory, developers should be mindful of object serialization and deserialization. Efficient serialization mechanisms are essential for achieving optimal performance, as they directly impact the latency and throughput of data operations.
The Closing Argument
In the quest for optimal performance, leveraging off-heap memory with Java ChronicleMap can be a game-changer for applications that demand low-latency, high-throughput data access. By bypassing the limitations of garbage collection and providing consistent access times, ChronicleMap offers a compelling solution for performance-critical use cases.
As with any optimization, careful consideration and benchmarking should be employed to assess the impact and benefits of off-heap memory. While off-heap memory can offer significant performance improvements, it requires diligence in memory management and a thorough understanding of the trade-offs involved.
In conclusion, Java ChronicleMap provides a powerful tool for harnessing the benefits of off-heap memory, paving the way for enhanced performance and scalability in Java applications.
By incorporating off-heap memory management techniques like ChronicleMap, Java developers can achieve significant performance boosts and set their applications on the path to success.
For more in-depth understanding, you can refer to the official documentation and performance benchmarks of Java ChronicleMap.