Common Pitfalls When Starting with Spring Data Solr
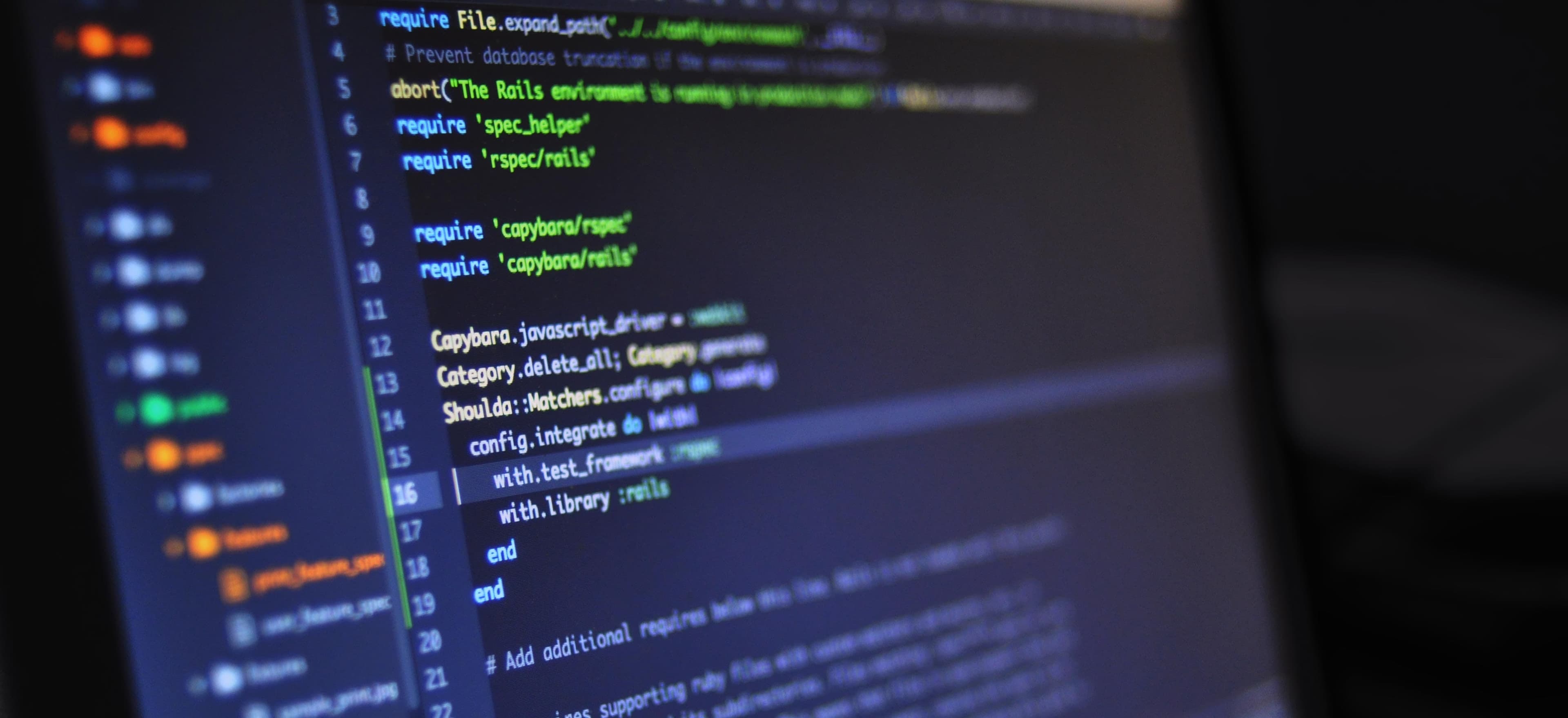
- Published on
Common Pitfalls When Starting with Spring Data Solr
Spring Data Solr is a powerful framework for working with Apache Solr, an open-source search platform. Integration of Solr with Spring allows developers to build robust applications that can handle large datasets with advanced search capabilities. However, newcomers to Spring Data Solr may encounter various pitfalls. In this blog post, we will explore common mistakes developers make when starting with Spring Data Solr and how to avoid them.
Understanding Spring Data Solr
Before diving into the pitfalls, let’s quickly recap what Spring Data Solr is. Spring Data Solr simplifies Solr integration by providing a repository abstraction tailored to Solr. It can help you perform CRUD (Create, Read, Update, Delete) operations easily, leverage repository functionalities, and engage with Solr’s querying capabilities.
Why Choose Spring Data Solr?
- Ease of Use: With Spring’s familiar programming model and syntax, you can quickly build dynamic applications.
- Powerful Features: It provides features like pagination, full-text search, and faceting out of the box.
- Community and Documentation: There is extensive support available from Spring’s community, and the documentation is comprehensive.
With that context, let's look at some common pitfalls.
1. Misunderstanding the Core Concepts
What to Avoid
A fundamental pitfall beginners make is misinterpreting the core concepts of Solr itself, such as documents, fields, and collections. A document in Solr is a set of fields, and collections allow you to store multiple documents.
Why Understanding Matters
Understanding these concepts is essential for successful data modeling and querying. For instance, not defining fields correctly could lead to data that is not searchable or performs poorly.
Example Code
Here’s a simple example of defining a document in Spring Data Solr:
import org.springframework.data.annotation.Id;
import org.springframework.data.solr.core.mapping.SolrDocument;
@SolrDocument(collection = "my_collection")
public class MySolrDocument {
@Id
private String id;
private String title;
private String description;
// Getters and setters
}
This code snippet defines a Solr document class. The @SolrDocument
annotation specifies which collection this document belongs to, and the @Id
annotation indicates that the 'id' field is the unique identifier.
2. Ignoring Configuration Nuances
What to Avoid
Not properly configuring your Solr server can lead to various runtime issues. Forgetting to set up the correct URL or enabling the required features in application.properties
could result in connectivity failures.
Why Configuration Matters
If the configuration is incorrect, your application won't be able to connect to Solr, thereby hindering search functionalities.
Example Code
Here's how you might set up the configuration in your application.properties
file:
spring.data.solr.host=http://localhost:8983/solr
spring.data.solr.repositories.enabled=true
This snippet configures the host where your Solr instance is running and enables Spring Data Solr repositories.
3. Not Utilizing Paging and Sorting
What to Avoid
Many developers fail to employ paging and sorting when querying data, which can lead to performance issues and user experience problems.
Why Paging and Sorting Matter
When dealing with large datasets, loading all results at once can inundate users and slow down the application. Implementing paging and sorting can significantly enhance performance and user satisfaction.
Example Code
Utilizing paging and sorting in your repository interface:
import org.springframework.data.domain.Pageable;
import org.springframework.data.solr.repository.SolrCrudRepository;
public interface MySolrDocumentRepository extends SolrCrudRepository<MySolrDocument, String> {
List<MySolrDocument> findByTitle(String title, Pageable pageable);
}
In this example, the findByTitle
method leverages Spring Data Solr's repository capabilities to return results in a paginated manner.
4. Overlooking Query Optimization
What to Avoid
Neglecting to optimize queries can result in slow response times, especially as data volumes grow. Developers might not utilize filter queries that could enhance performance.
Why Optimization Matters
Optimizing queries can drastically reduce execution time and improve user experience. Utilizing features like caching, filter queries, and appropriate field types makes a significant difference.
Example Code
For example, using filter queries can optimize your search:
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.solr.core.query.FilterQuery;
import org.springframework.data.solr.core.query.SimpleFilterQuery;
public Page<MySolrDocument> searchWithFilters(String title, String category) {
FilterQuery filterQuery = new SimpleFilterQuery();
filterQuery.addCriteria(new Criteria("category").is(category));
return mySolrDocumentRepository.findByTitle(title, PageRequest.of(0, 10));
}
The above code combines a regular search with a filter, providing both relevant results and optimized performance.
5. Not Handling Exceptions Gracefully
What to Avoid
Failing to manage exceptions properly can lead to poor user experiences. Beginners often neglect to catch specific exceptions, leading to general error messages that don't provide helpful context to users.
Why Exception Handling Matters
Good exception handling aids debugging and helps build a robust application. It ensures that errors are logged properly and can be presented to users in a friendly manner.
Example Code
Here is how you might handle exceptions for your repository:
import org.springframework.dao.DataAccessException;
import org.springframework.stereotype.Service;
@Service
public class MySolrService {
public List<MySolrDocument> findDocuments(String title) {
try {
return mySolrDocumentRepository.findByTitle(title);
} catch (DataAccessException e) {
// Log the error and return an empty list or an informative message
System.err.println("Error fetching documents: " + e.getMessage());
return Collections.emptyList();
}
}
}
This code catches database access exceptions and allows the application to continue functioning while logging the error for further investigation.
6. Skipping Testing and Validation
What to Avoid
Another frequent pitfall is forgoing testing, especially integration testing. Many developers are excited to push their applications into production without verifying their functionality.
Why Testing Matters
Thorough testing (unit and integration) ensures that components behave as expected. It helps catch integration issues between Spring and Solr before they impact users.
Example Code
You might use Spring's testing support as follows:
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.data.solr.DataSolrTest;
import org.springframework.test.context.junit.jupiter.SpringExtension;
import org.junit.jupiter.api.extension.ExtendWith;
@ExtendWith(SpringExtension.class)
@DataSolrTest(MySolrDocumentRepository.class)
public class MySolrDocumentRepositoryTest {
@Autowired
private MySolrDocumentRepository repository;
@Test
public void testRepository() {
// Test your repository methods here
// e.g., assertEquals(expectedResult, repository.findByTitle("example title"));
}
}
This snippet demonstrates how to set up a simple test for your Solr repository using Spring's testing framework.
The Bottom Line
Spring Data Solr offers fantastic capabilities for developers building applications around Apache Solr, but it is not without its challenges. By avoiding the common pitfalls outlined above—understanding core concepts, focusing on proper configurations, utilizingpaging and sorting, optimizing queries, handling exceptions gracefully, and embracing thorough testing—you can greatly streamline your development process.
As you embark on your journey with Spring Data Solr, consider referring to the official documentation for an in-depth understanding. You may also find the Spring Data Release Notes helpful for exploring new features and updates.
By arming yourself with knowledge and best practices, you can unleash the full potential of Spring Data Solr in your projects. Happy coding!
Checkout our other articles