Tiny Steps to Big Success: Incremental Feature Development
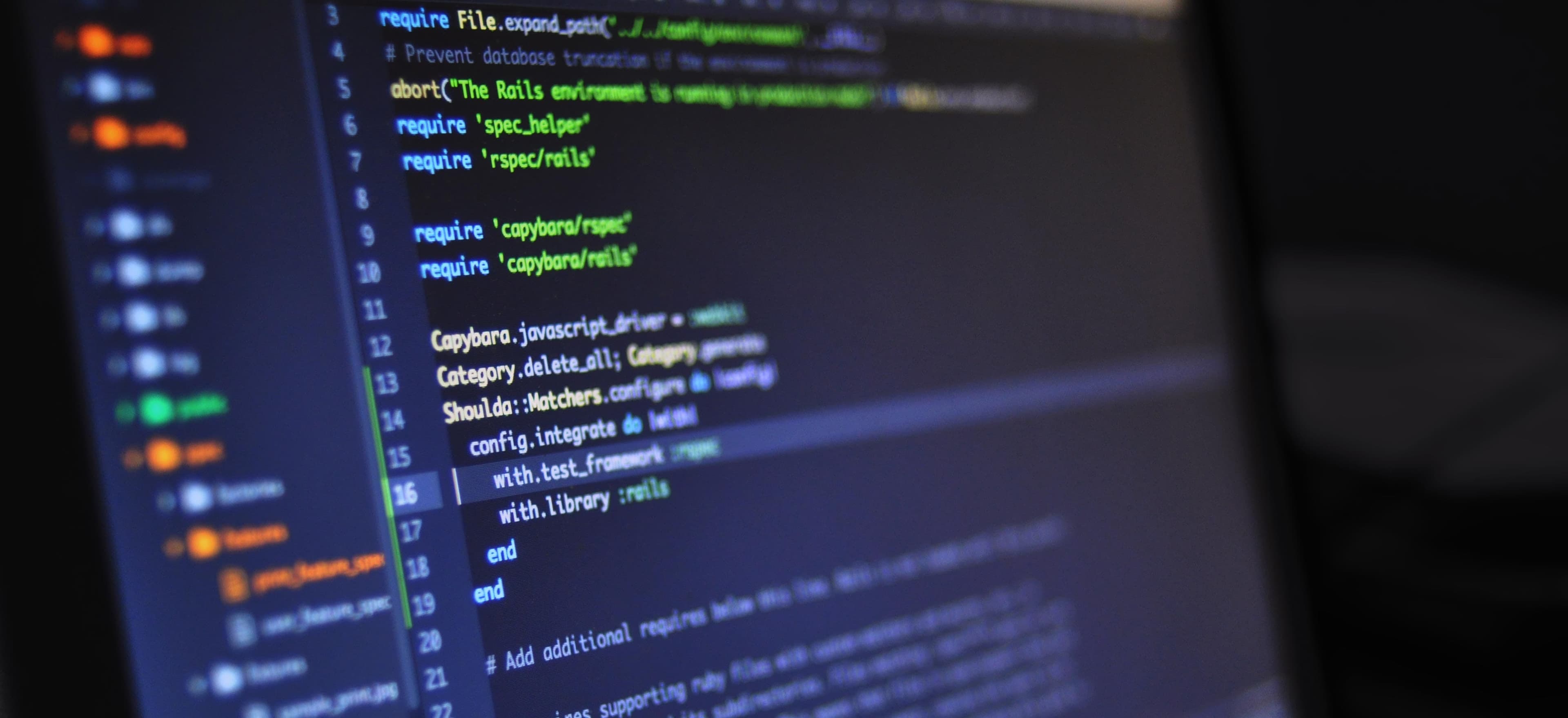
- Published on
Incremental Feature Development: The Power of Small Steps
In the fast-paced world of software development, the ability to efficiently deliver features is crucial. Incremental feature development, a concept deeply rooted in agile methodologies, offers a strategic approach to building and evolving software. By breaking down larger features into smaller, manageable tasks, teams can continuously deliver value to users while maintaining flexibility and adaptability. In this blog post, we'll explore the benefits of incremental feature development and how Java, with its robust ecosystem and powerful libraries, is well-suited for this approach.
Embracing Agility with Incremental Feature Development
Software development is an iterative process, and incremental feature development aligns seamlessly with this reality. Instead of attempting to deliver entire features in a single release, teams employing incremental development focus on delivering small, functional increments of a feature with each iteration. This approach not only allows for quicker user feedback but also mitigates the risks associated with long development cycles and potential derailments.
The Power of Small Steps
Breaking down features into smaller steps offers several tangible benefits. It allows for early testing and validation, enabling teams to make course corrections early in the development cycle. Furthermore, smaller increments make it easier to manage change, as adjustments can be incorporated without disrupting the entire feature implementation. This flexibility is particularly valuable in today's dynamic and competitive software landscape.
Java: The Ideal Companion for Incremental Feature Development
Java, with its robust tools, extensive libraries, and a vast developer community, is a natural fit for incremental feature development. Let's explore some key aspects that make Java an ideal choice for this approach.
Versatility of Java Libraries
Java boasts a rich set of libraries that cater to various aspects of software development. Whether it's building RESTful APIs with JAX-RS, managing dependencies with Maven or Gradle, or implementing unit tests with JUnit, Java's libraries empower developers to focus on incremental development without reinventing the wheel for every feature.
Strong Typing and Refactoring Support
Java's static typing and robust IDE support (like IntelliJ IDEA or Eclipse) provide a safety net for refactoring code during incremental development. The compiler's ability to catch type-related errors at compile-time, along with the refactoring tools, enables developers to confidently make changes and iterate on features without the fear of introducing unexpected issues.
// Example of Java's strong typing
public class IncrementalFeature {
private int featureStep;
public void setFeatureStep(int step) {
this.featureStep = step;
}
}
In the above code snippet, the strong typing of Java ensures that only integers can be assigned to featureStep
, preventing runtime type-related errors.
Ecosystem for Continuous Integration and Delivery (CI/CD)
Java's extensive ecosystem supports CI/CD practices, allowing teams to automate the build, test, and deployment processes. Tools like Jenkins, Bamboo, or GitLab CI seamlessly integrate with Java projects, enabling teams to continuously deliver incremental features with confidence.
Implementing Incremental Feature Development in Java
Now, let's delve into the practical aspects of implementing incremental feature development in Java.
Breaking Down Features into User Stories
In agile development, features are often expressed as user stories. These user stories can be further decomposed into smaller, actionable tasks or sub-stories. For instance, if the feature involves adding a user authentication mechanism, the incremental steps could include designing the user interface, implementing backend logic, and integrating with a security framework.
Utilizing Feature Branches in Version Control
Version control systems like Git support feature branching, allowing developers to work on specific increments of a feature in isolation. By creating feature branches and merging them back into the main codebase upon completion, teams can effectively manage the incremental development process without disrupting ongoing work.
# Create a new feature branch
git checkout -b feature/auth-increment-1
# Work on the feature increment
# Commit changes
git commit -m "Implement user authentication UI"
# Merge the feature branch back into main
git checkout main
git merge feature/auth-increment-1
Writing Test-Driven Code
Adopting test-driven development (TDD) practices in Java empowers teams to write tests for each incremental feature, ensuring that new functionality is thoroughly tested and validated. Tools like JUnit and Mockito facilitate the creation of unit tests and mock objects, enabling confident refactoring and continuous integration.
// Test-driven development with JUnit
public class IncrementalFeatureTest {
@Test
public void testFeatureIncrement() {
IncrementalFeature feature = new IncrementalFeature();
feature.setFeatureStep(1);
assertEquals(1, feature.getFeatureStep());
}
}
Continuous Integration and Deployment
Integrating an incremental feature development workflow with CI/CD pipelines ensures that each feature increment is automatically built, tested, and deployed. This streamlines the process of delivering features to users, maintaining a consistent cadence of value delivery.
Embracing Continuous Improvement
Incremental feature development in Java is not just a methodology; it's a mindset. By continually seeking feedback, reflecting on the development process, and embracing a culture of improvement, teams can elevate their ability to deliver impactful software in small, iterative steps.
Wrapping Up
Incremental feature development, when embraced with the right tools and mindset, empowers teams to navigate the complexities of software development with agility and resilience. Java, with its versatile libraries, strong typing, and CI/CD support, provides a conducive environment for thriving in an incremental development paradigm. By breaking down features into manageable increments, Java developers can deliver continuous value to users while adapting to evolving requirements with ease. Embracing the incremental approach is not just about taking small steps; it's about unlocking the potential for big successes in software development.