Avoid Common Java 8 Base64 Encoding/Decoding Pitfalls
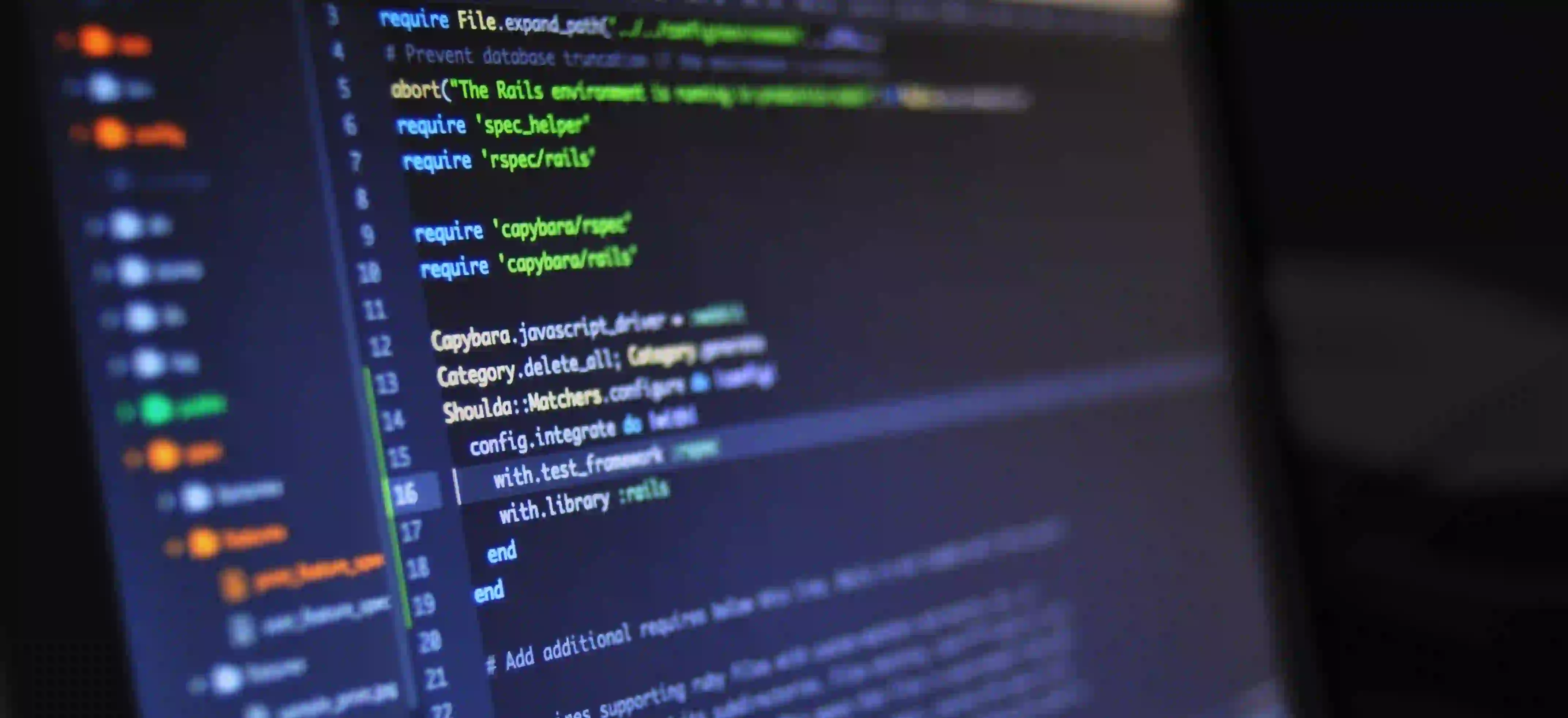
Avoid Common Java 8 Base64 Encoding/Decoding Pitfalls
Base64 encoding and decoding are common tasks in software development, especially when working with data serialization, authentication mechanisms, and data transmission over the network. In Java, the introduction of the java.util.Base64 class in Java 8 simplified these operations. However, developers often encounter pitfalls and mistakes when using it. In this post, we'll discuss common pitfalls and best practices to avoid them.
Pitfall 1: Incorrect Encoding or Decoding
One of the common mistakes is incorrectly encoding or decoding the data. Let's consider encoding a byte array to Base64.
import java.util.Base64;
public class Base64Example {
public static void main(String[] args) {
String original = "Hello, Base64 Encoding!";
byte[] bytes = original.getBytes();
String encodedString = Base64.getEncoder().encodeToString(bytes);
System.out.println("Encoded: " + encodedString);
byte[] decodedBytes = Base64.getDecoder().decode(encodedString);
String decodedString = new String(decodedBytes);
System.out.println("Decoded: " + decodedString);
}
}
In the example above, we encode a string to Base64 and then decode it back. If we mistakenly use a different decoder to decode the encoded string, or apply incorrect configurations during encoding, it can lead to unexpected results.
Best Practice: Always use the corresponding encoder to encode data and decoder to decode data. Ensure that the same decoder configuration is used to decode the encoded string.
Pitfall 2: Mishandling Unsupported Characters
Base64 encoding produces a string comprising a limited set of characters, namely A-Z, a-z, 0-9, +, and /. Mishandling these characters can lead to issues during transmission or storage.
import java.util.Base64;
public class Base64Example {
public static void main(String[] args) {
String original = "Hello, Base64 Encoding! #$%^";
byte[] bytes = original.getBytes();
String encodedString = Base64.getEncoder().encodeToString(bytes);
System.out.println("Encoded: " + encodedString);
}
}
If the original string contains unsupported characters like #$%^, the encoding process will produce an invalid Base64 string containing unsupported characters.
Best Practice: Always ensure that the input data to be encoded is compatible with Base64 encoding. Sanitize the input data if necessary to remove any unsupported characters.
Pitfall 3: Handling Large Data
Base64 encoding is known to increase the size of the data by approximately 33%. When dealing with large data, this increase in size can lead to memory issues, especially if the encoded data needs to be held in memory.
import java.util.Base64;
import java.nio.file.Files;
import java.nio.file.Path;
import java.io.IOException;
public class Base64Example {
public static void main(String[] args) {
try {
Path filePath = Path.of("largeFile.zip");
byte[] fileData = Files.readAllBytes(filePath);
String encodedString = Base64.getEncoder().encodeToString(fileData);
System.out.println("Encoded: " + encodedString);
} catch (IOException e) {
// Handle exception
}
}
}
In the above example, if the largeFile.zip is significantly large, the attempt to encode it to Base64 and hold it in memory as a string might result in OutOfMemoryError.
Best Practice: When dealing with large data, prefer using streaming processing to avoid holding the entire encoded data in memory at once.
Pitfall 4: Misunderstanding Configurations
The java.util.Base64 class provides the flexibility to configure the encoding and decoding behavior. When misunderstandings arise in configuring the encoder or decoder, it can lead to unexpected outcomes.
import java.util.Base64;
public class Base64Example {
public static void main(String[] args) {
String original = "Hello, Base64 Encoding!";
byte[] bytes = original.getBytes();
String encodedString = Base64.getEncoder().withoutPadding().encodeToString(bytes);
System.out.println("Encoded: " + encodedString);
byte[] decodedBytes = Base64.getDecoder().decode(encodedString);
String decodedString = new String(decodedBytes);
System.out.println("Decoded: " + decodedString);
}
}
In the above example, by using withoutPadding()
during encoding, the configuration is not preserved during decoding, leading to unexpected results.
Best Practice: Always ensure that the configuration used during encoding is preserved and correctly used during decoding.
Lessons Learned
Base64 encoding and decoding are essential operations in Java development, and avoiding pitfalls during these processes is crucial. By understanding the common pitfalls and following best practices, developers can effectively use the java.util.Base64 class, ensuring robust and reliable encoding and decoding of data.
Remember to continuously refer to the Java documentation for further details and guidance.
By adopting these practices, you can safeguard your applications from common Base64 encoding and decoding pitfalls. Happy coding!