Unlock Agile Success: Tackling CI/CD Integration Challenges
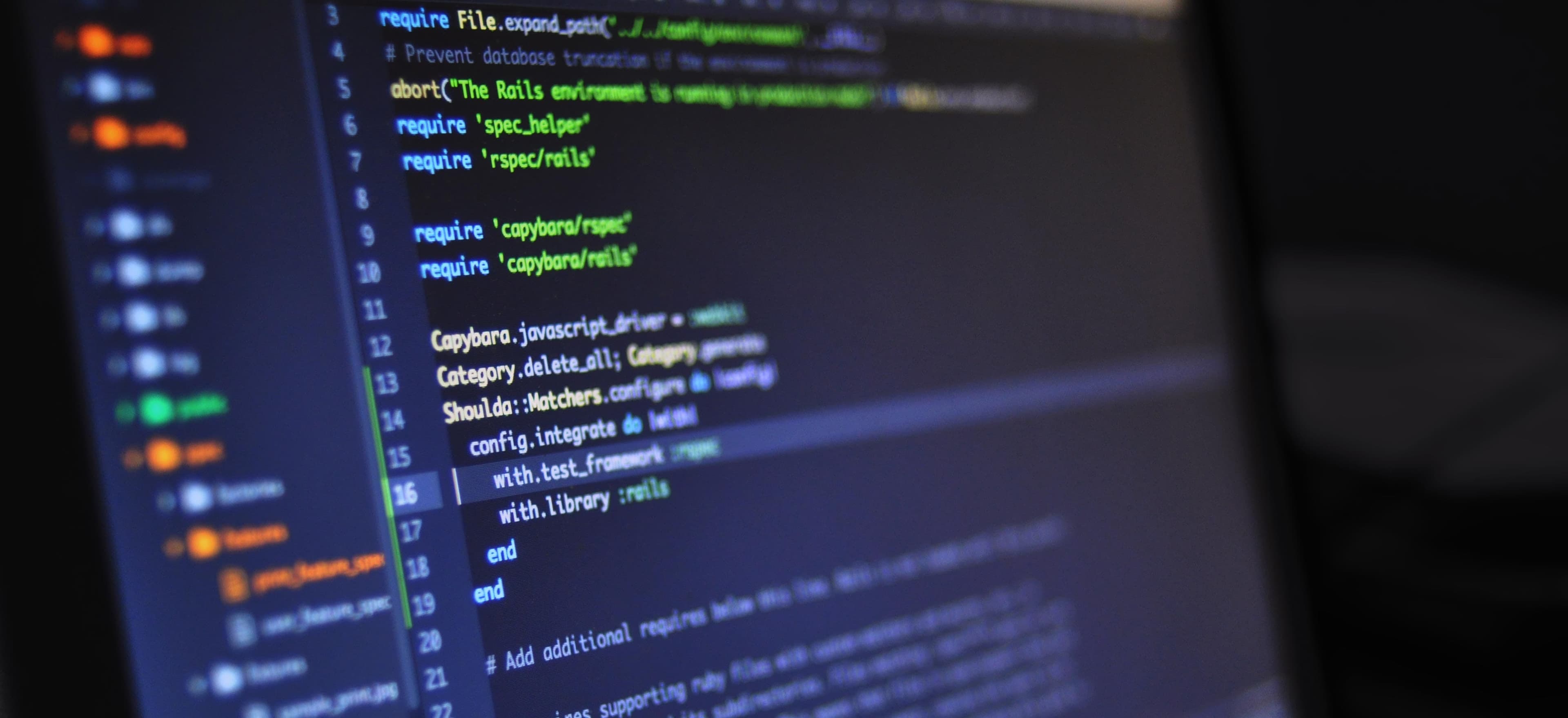
- Published on
Tackling CI/CD Integration Challenges for Agile Success
In the realm of Agile software development, Continuous Integration (CI) and Continuous Deployment (CD) are integral components for achieving success. By automating the building, testing, and deployment processes, CI/CD pipelines ensure a streamlined and efficient delivery of software. However, integrating CI/CD into the Agile workflow comes with its own set of challenges. In this post, we'll explore some of these challenges and discuss strategies to overcome them.
Challenge 1: Ensuring Consistent Build Environments
One of the fundamental requirements of CI is the ability to ensure a consistent build environment. This consistency is crucial for guaranteeing that the code will behave the same way across different stages of the pipeline. To address this challenge, containerization technologies such as Docker can be leveraged. By encapsulating the build environment within a Docker container, developers can maintain consistency across various stages of the CI/CD pipeline.
// Utilizing Docker for consistent build environments
FROM openjdk:11
WORKDIR /app
COPY . /app
RUN javac Main.java
CMD ["java", "Main"]
The above Dockerfile snippet demonstrates how a Java application can be containerized, allowing for uniformity in the build environment.
Challenge 2: Managing Dependencies and Version Control
In a typical Agile project, managing dependencies and ensuring version control across different stages of the CI/CD pipeline can be complex. Dependency management tools like Apache Maven prove to be immensely beneficial in this scenario. Maven helps in managing project dependencies and facilitates uniformity in the build process, ensuring that the same set of dependencies and versions are used throughout the pipeline.
<!-- Declaring dependencies in pom.xml -->
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
By defining dependencies in a pom.xml
file, Maven simplifies the process of managing dependencies and version control.
Challenge 3: Implementing Automated Testing
Automated testing is a cornerstone of the CI/CD process, and ensuring its seamless integration into the Agile workflow is crucial. Utilizing frameworks like JUnit for unit testing and Selenium for end-to-end testing is essential for achieving comprehensive test coverage. Additionally, implementing test automation tools like Jenkins allows for the execution of automated tests as part of the CI/CD pipeline.
// Example of JUnit test case
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class SampleTest {
@Test
public void testAddition() {
assertEquals(4, 2 + 2);
}
}
The above JUnit test case ensures that the addition functionality meets the expected outcome, thus validating the code's integrity.
Challenge 4: Enabling Continuous Deployment
Continuous Deployment involves automating the deployment process to deliver software changes to production. While this facilitates rapid and frequent releases, it also presents the challenge of ensuring a robust deployment pipeline. Tools like Jenkins, in combination with deployment automation scripts, enable seamless deployment to various environments, while also allowing for manual interventions when necessary.
// Example of Jenkins Pipeline for Continuous Deployment
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Deploy') {
steps {
sh 'sh deploy.sh'
}
}
}
}
The Jenkins Pipeline script depicted above orchestrates the build and deployment stages, ensuring a continuous deployment process.
Lessons Learned
In the Agile software development landscape, addressing the challenges of integrating CI/CD into the workflow is pivotal for achieving rapid and reliable software delivery. By leveraging containerization, dependency management tools, automated testing frameworks, and deployment automation, teams can surmount these challenges and unlock Agile success.
To delve deeper into the concepts discussed, explore more about building Java applications with Docker, and best practices for using Jenkins Pipelines.
By embracing these strategies and technologies, Agile teams can fortify their CI/CD pipelines, ensuring a seamless integration that propels them towards Agile success.