Master Java 8: Simplify Getting Current Date and Time
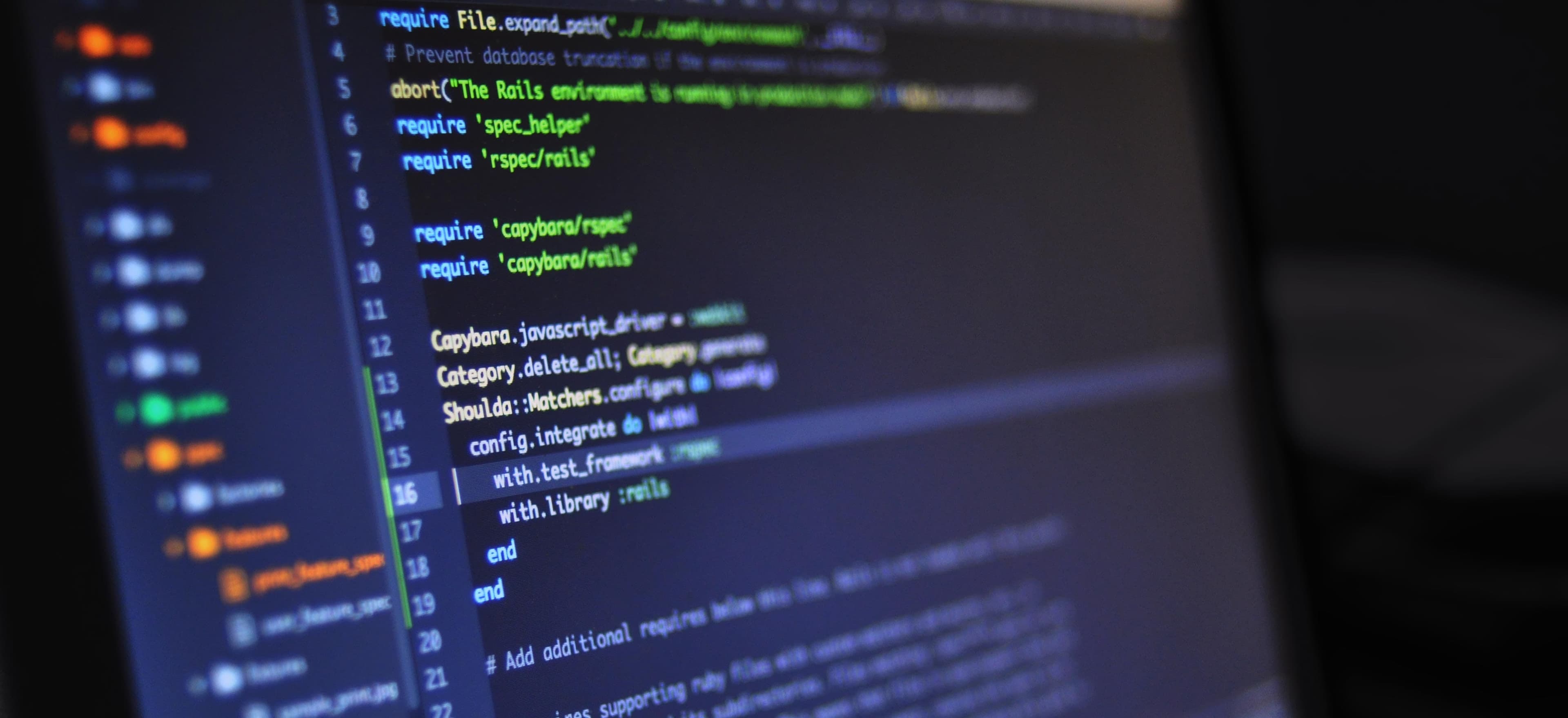
- Published on
Master Java 8: Simplify Getting Current Date and Time
When it comes to working with dates and times in Java, Java 8 introduced the java.time
package, which provides a more comprehensive and flexible API for date and time manipulation. In this blog post, we will explore how Java 8's date and time API simplifies the process of obtaining the current date and time.
Obtaining the Current Date and Time
In traditional versions of Java, obtaining the current date and time involved using java.util.Date
and java.util.Calendar
, which were not the most intuitive and often led to cumbersome code. With Java 8, however, the java.time
package makes this process much more straightforward.
To obtain the current date, Java 8 provides the LocalDate
class. It represents a date without time-zone information. Let's take a look at how we can obtain the current date using LocalDate
:
import java.time.LocalDate;
public class CurrentDateExample {
public static void main(String[] args) {
LocalDate currentDate = LocalDate.now();
System.out.println("Current Date: " + currentDate);
}
}
In the above example, LocalDate.now()
returns the current date, simplifying the process of obtaining the date without the need for additional conversions or complex logic.
Similarly, for obtaining the current time, Java 8 offers the LocalTime
class, which represents a time without time-zone information. Here's how we can obtain the current time:
import java.time.LocalTime;
public class CurrentTimeExample {
public static void main(String[] args) {
LocalTime currentTime = LocalTime.now();
System.out.println("Current Time: " + currentTime);
}
}
Using LocalTime.now()
simplifies the task of obtaining the current time, eliminating the need for manual manipulation of date and time components.
Obtaining the Current Date and Time Together
In addition to obtaining the current date and time separately, Java 8 provides the LocalDateTime
class, which represents a date and time without time-zone information. This simplifies the process of obtaining the current date and time together. Let's take a look at an example:
import java.time.LocalDateTime;
public class CurrentDateTimeExample {
public static void main(String[] args) {
LocalDateTime currentDateTime = LocalDateTime.now();
System.out.println("Current Date and Time: " + currentDateTime);
}
}
By using LocalDateTime.now()
, we can effortlessly obtain the current date and time in a single step, streamlining the process and improving code readability.
Additional Flexibility with Time Zones
Java 8's date and time API also provides the ZonedDateTime
class, which represents a date and time with a time-zone. This is incredibly useful when dealing with applications or systems that operate across different time zones. Let's consider an example:
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class ZonedDateTimeExample {
public static void main(String[] args) {
ZoneId zoneId = ZoneId.of("America/New_York");
ZonedDateTime currentDateTimeInNY = ZonedDateTime.now(zoneId);
System.out.println("Current Date and Time in New York: " + currentDateTimeInNY);
}
}
In the above example, the ZoneId.of("America/New_York")
allows us to specify the time zone, and ZonedDateTime.now(zoneId)
returns the current date and time for that particular time zone. This demonstrates the flexibility and convenience provided by Java 8's date and time API when working with time zones.
Key Takeaways
In summary, Java 8's date and time API simplifies the task of obtaining the current date and time by providing intuitive and flexible classes such as LocalDate
, LocalTime
, LocalDateTime
, and ZonedDateTime
. These classes streamline the process, making the code more readable and maintainable. By leveraging Java 8's date and time API, developers can handle date and time operations with ease and precision.
By mastering these concepts, you can streamline your code, simplify maintenance, and ensure your applications operate reliably across different time zones. So, embrace the power of Java 8's date and time API and simplify your date and time manipulation tasks today!
Continue to explore the Java 8 date and time API to unleash its full potential, and stay tuned for more insightful Java-related content to enhance your programming prowess.
Happy coding!