JVM Defaults: Safe Settings or Silent Performance Thieves?
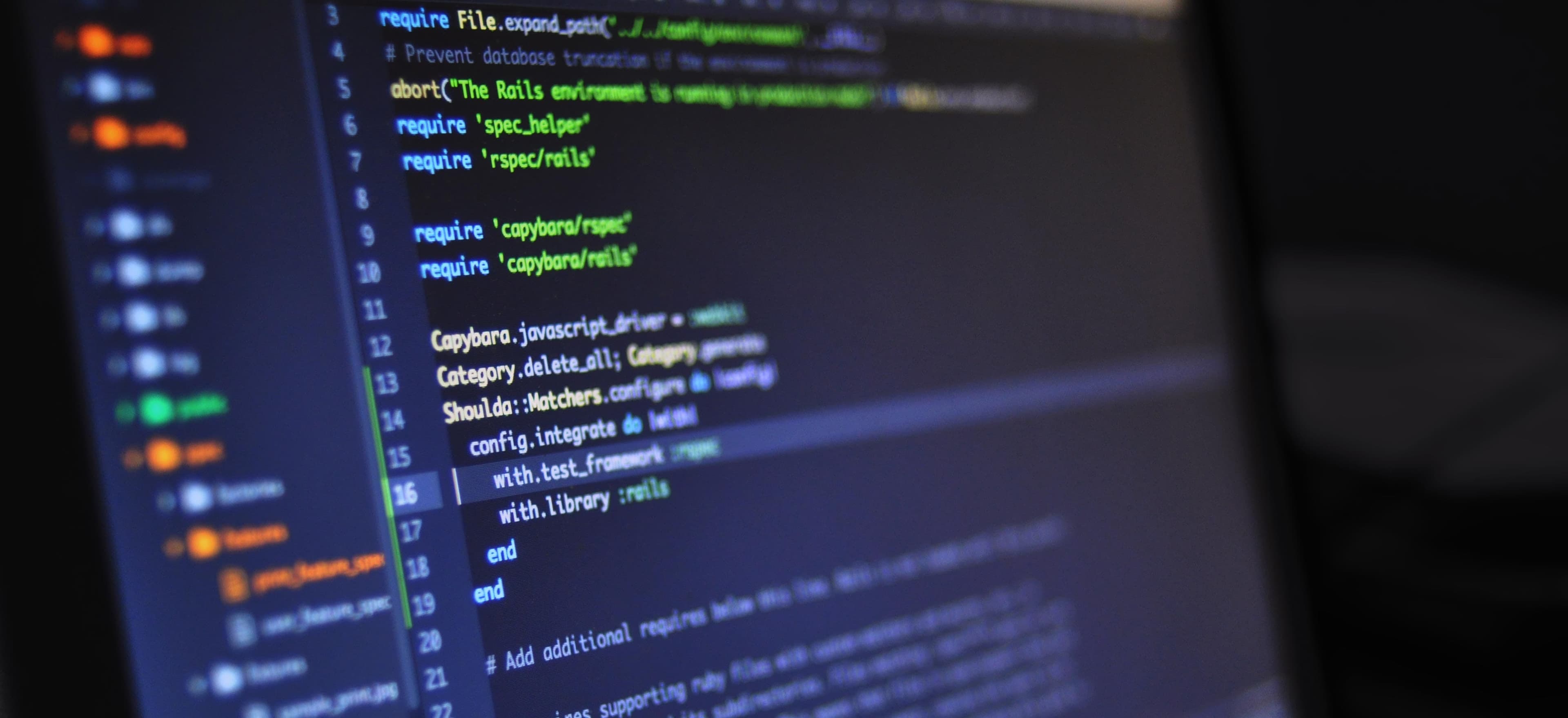
- Published on
JVM Defaults: Safe Settings or Silent Performance Thieves?
When it comes to developing Java applications, understanding the defaults of the Java Virtual Machine (JVM) is essential. The JVM comprises various parameters and settings that can significantly impact the performance, stability, and security of your Java applications. While the default settings are designed to cater to a broad range of applications, they may not always be optimal for specific use cases. In this article, we'll delve into the defaults of the JVM, their implications, and how to optimize them for improved performance.
Default JVM Settings
The JVM comes with a set of default parameters that govern its behavior. These defaults are chosen to provide a balance between performance, compatibility, and resource consumption across different hardware and software environments. However, these settings may not be the most suitable for all applications. Let's explore some of the most crucial default settings and their implications.
Heap Memory Settings
By default, the JVM assigns a relatively conservative amount of memory to the heap, which is the storage for Java objects. This default setting aims to prevent applications from consuming excessive system memory, which could lead to resource contention and potential performance degradation. However, for memory-intensive applications, this default setting might result in frequent garbage collection events and diminished performance.
Garbage Collection Settings
The JVM's default garbage collection settings are designed to provide a balanced approach to reclaiming memory while minimizing pause times. However, these settings might not be optimal for all applications. For instance, applications with stringent performance requirements or large heap sizes may benefit from fine-tuning the garbage collection settings to reduce pause times and overall overhead.
Thread Stack Size
The default thread stack size in the JVM is typically conservative to ensure that a large number of threads can coexist without exhausting the available memory. While this default is suitable for many applications, certain types of applications, such as those involving heavy concurrency or deep call stack requirements, may benefit from increasing the thread stack size to prevent stack overflow errors and improve performance.
Just-In-Time (JIT) Compilation
The JVM employs JIT compilation to optimize performance by dynamically compiling bytecode to native machine code. The default JIT compilation settings are often versatile, aiming to provide a reasonable balance between startup time and long-term performance. However, for long-running server applications, adjusting the JIT compilation settings can result in improved overall performance.
Optimizing JVM Settings
Heap Memory Adjustment
For memory-intensive applications, adjusting the heap memory settings is often crucial. By increasing the heap size, applications can accommodate more objects without triggering frequent garbage collection cycles. However, it's essential to perform thorough testing to avoid excessive memory allocation, which could lead to out-of-memory errors and adversely impact the system's stability.
// Example of increasing heap memory using the -Xmx flag
java -Xmx4g -jar myapplication.jar
In this example, the -Xmx4g
flag sets the maximum heap size to 4 gigabytes, which can be adjusted based on the application's requirements and the available system resources.
Garbage Collection Tuning
Fine-tuning the garbage collection settings can significantly impact the application's responsiveness and throughput. For example, by configuring the garbage collector to use a specific algorithm or adjusting the collection intervals, it's possible to mitigate pause times and reduce the overall impact of garbage collection on application performance.
// Example of specifying the garbage collector using the -XX:+UseG1GC flag
java -XX:+UseG1GC -jar myapplication.jar
In this example, the -XX:+UseG1GC
flag enables the Garbage-First (G1) garbage collector, which is known for its low-latency and high-throughput characteristics, making it suitable for memory-intensive applications.
Thread Stack Size Adjustment
Applications with specific thread utilization patterns or deep call stacks can benefit from adjusting the default thread stack size. By increasing the thread stack size, stack overflow errors can be mitigated, and performance can be improved in scenarios where deep recursion or extensive thread utilization is prevalent.
// Example of increasing thread stack size using the -Xss flag
java -Xss2m -jar myapplication.jar
In this example, the -Xss2m
flag sets the thread stack size to 2 megabytes, providing a larger stack space for each thread to accommodate deeper call stacks.
JIT Compilation Customization
For long-running server applications, customizing the JIT compilation settings can yield performance improvements over time. By adjusting the threshold for JIT compilation, modifying the inlining policies, or enabling specific optimizations, it's possible to tailor the JIT compiler's behavior to match the application's usage patterns and performance requirements.
// Example of customizing JIT compilation using the -XX:CompileThreshold flag
java -XX:CompileThreshold=1000 -jar myapplication.jar
In this example, the -XX:CompileThreshold=1000
flag sets the threshold for the number of method invocations before JIT compilation kicks in, allowing for earlier optimization of frequently executed code paths.
Key Takeaways
Understanding and optimizing the JVM defaults is crucial for achieving optimal performance and resource utilization in Java applications. While the defaults are designed to cater to a broad range of use cases, they may not always align with the specific requirements of your application. By fine-tuning parameters such as heap memory allocation, garbage collection behavior, thread stack sizes, and JIT compilation settings, it's possible to tailor the JVM to suit the precise needs of your application, ultimately leading to improved performance, stability, and responsiveness.
By carefully adjusting these settings based on your application's characteristics and usage patterns, you can unleash the full potential of your Java applications, ensuring that the JVM works for you, not against you.
Remember, the JVM defaults are just the starting point—optimizing them is where the true performance gains await.
Interested in more Java performance tuning techniques? Check out this comprehensive guide for additional insights.
Checkout our other articles