Avoiding Overuse of Spring's @Primary: Best Practices
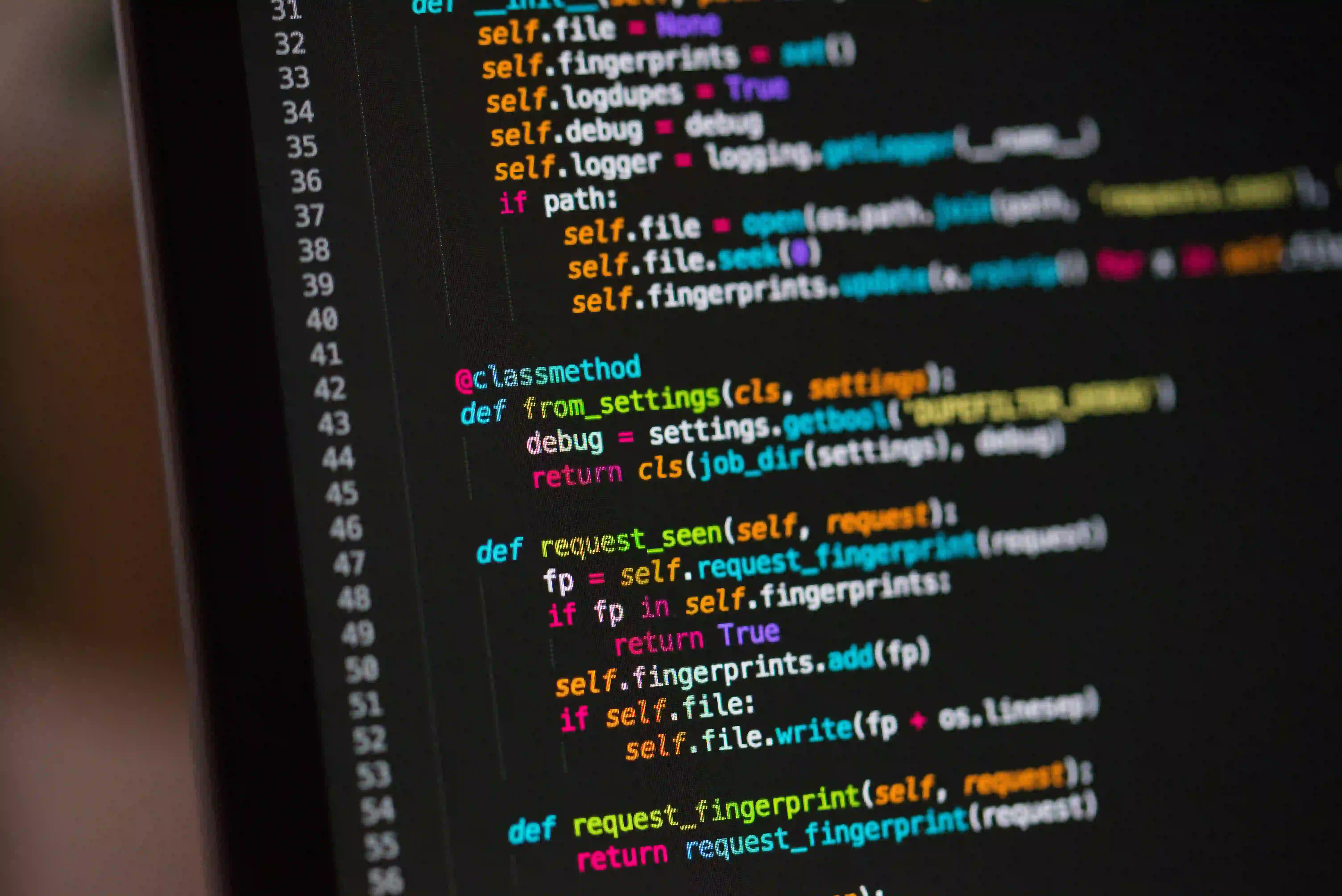
Avoiding Overuse of Spring's @Primary: Best Practices
When developing a complex Java application with Spring, you'll often encounter scenarios where multiple beans of the same type need to be defined. You might be tempted to use Spring's @Primary
annotation to designate a primary bean for injection, but overusing this feature can lead to maintenance issues and confusion down the road. In this blog post, we'll explore the best practices for using @Primary
in Spring and how to avoid its overuse.
Understanding @Primary
The @Primary
annotation in Spring is used to specify a primary bean when multiple beans of the same type are present. When autowiring beans of that type, the primary bean will be injected by default. While @Primary
can be a convenient way to establish a default bean, it should be used judiciously to avoid creating tangled dependencies and unclear code.
Best Practices for Using @Primary
1. Use @Primary Sparingly
While it's tempting to designate a primary bean for every bean type, it's important to use @Primary
sparingly. Overusing @Primary
can lead to ambiguity in the codebase, especially as the application grows. Instead, consider alternatives such as using @Qualifier
or refactoring the code to reduce the need for multiple beans of the same type.
2. Choose @Primary for Default Implementations
Reserve the use of @Primary
for defining a default implementation of a bean type. This can be particularly useful when you want to provide a fallback option for autowiring without explicitly specifying the bean to use.
3. Leverage @Qualifier for Specific Cases
In scenarios where you need to wire a specific bean of a type, prefer using @Qualifier
over @Primary
. @Qualifier
allows you to specify the bean to be injected based on the qualifier value, providing more explicit control and reducing ambiguity in the code.
4. Document @Primary Usage
When you do use @Primary
, make sure to document the reasons why a certain bean has been designated as the primary one. This can help future developers understand the intent behind the primary bean and minimize confusion.
5. Consider Refactoring for Clarity
If you find yourself relying heavily on @Primary
for multiple beans of the same type, take a step back and consider refactoring the code. Introducing clearer distinctions between bean types or consolidating functionality into fewer beans can reduce the need for @Primary
and improve code maintainability.
Example: Using @Primary Sparingly
Let's take a look at an example to illustrate the impact of using @Primary
sparingly. Suppose we have a NotificationService
interface with two different implementations: EmailNotificationService
and SlackNotificationService
. We want to designate EmailNotificationService
as the primary bean for autowiring.
public interface NotificationService {
void sendNotification(String message);
}
@Component
@Primary
public class EmailNotificationService implements NotificationService {
// Implementation for sending email notifications
}
@Component
public class SlackNotificationService implements NotificationService {
// Implementation for sending Slack notifications
}
In this example, we've used @Primary
to designate EmailNotificationService
as the primary bean. This approach is appropriate because we are providing a default implementation for the NotificationService
interface.
A Final Look
In conclusion, while @Primary
can be a useful tool for defining primary beans in Spring, it should be used judiciously to avoid creating a web of tangled dependencies. By using @Primary
sparingly, choosing it for default implementations, leveraging @Qualifier
for specificity, documenting usage, and considering refactoring for clarity, you can effectively manage the use of @Primary
in your Spring application.
By following these best practices, you can maintain a clean and understandable codebase while leveraging the power of Spring's bean injection mechanism.
Remember, the goal is not to eliminate the use of @Primary
altogether but rather to use it in a strategic and intentional manner to enhance the clarity and maintainability of your Spring application.
For further reading on this topic, you can refer to the Spring Framework documentation on @Primary
and bean autowiring.
Implementing these best practices will undoubtedly contribute to the overall robustness and cleanliness of your codebase, while still leveraging the power and flexibility of Spring's dependency injection.
So, keep coding and keep your Spring applications clean and well-architected!