Streamline Integration Testing with the Docker Maven Plugin!
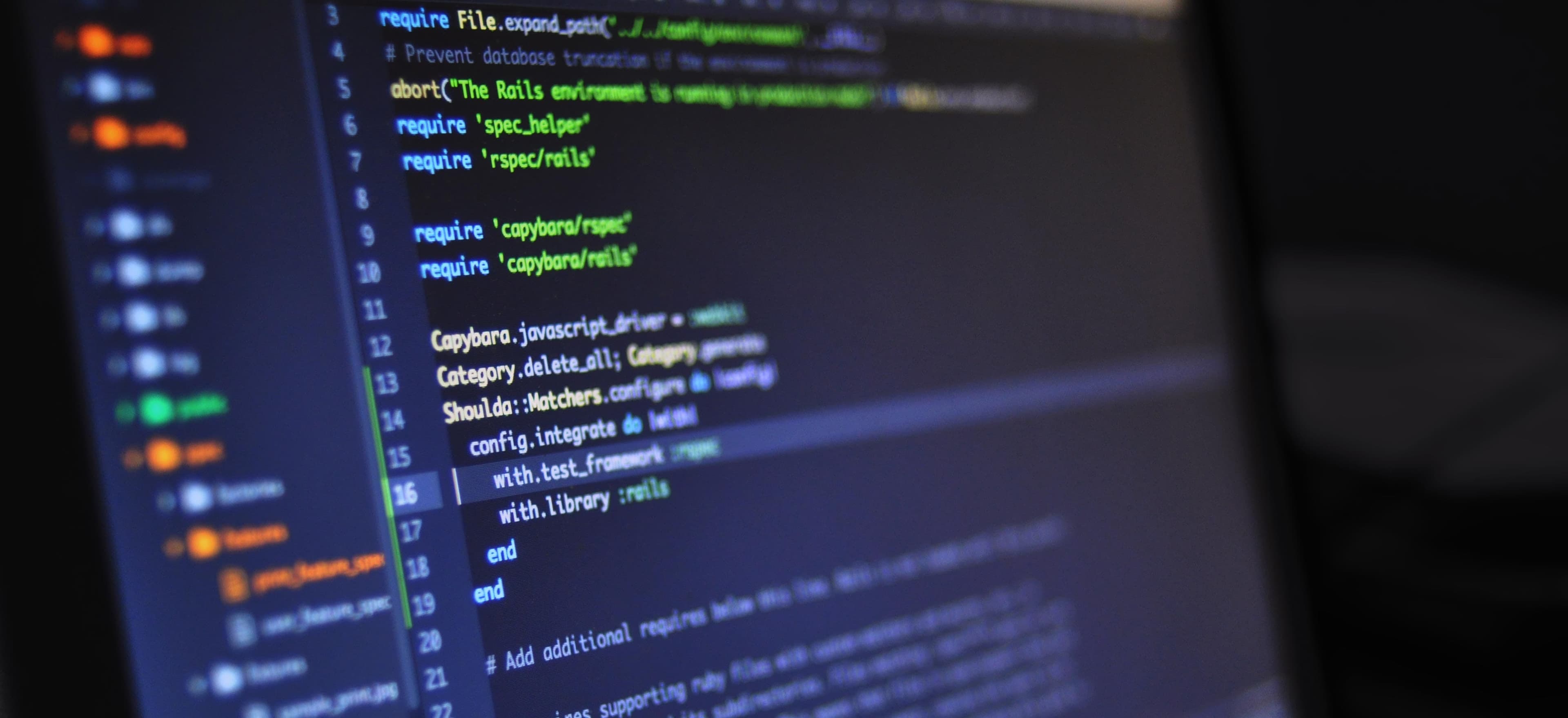
- Published on
Streamline Integration Testing with the Docker Maven Plugin
Integration testing can be a cumbersome process, often requiring complex setup and configuration. However, by leveraging the Docker Maven Plugin, you can streamline the integration testing process for your Java applications. This plugin allows you to build and manage Docker images as part of your Maven build process, making it easier to create disposable environments for testing.
In this blog post, we will explore how to use the Docker Maven Plugin to simplify integration testing for your Java applications. We will cover the basic setup, building Docker images, and running integration tests against these images. Let's dive in!
What is the Docker Maven Plugin?
The Docker Maven Plugin is a plugin for Maven that allows you to build and manage Docker images as part of your build process. It provides goals for building images, pushing them to a registry, and running containers. This plugin integrates seamlessly with your Maven project, allowing you to define your Docker image configuration directly within your pom.xml
file.
Setting Up the Docker Maven Plugin
To use the Docker Maven Plugin in your project, you need to include the plugin configuration in your pom.xml
file. Here's an example configuration that sets up the plugin:
<build>
<plugins>
<plugin>
<groupId>io.fabric8</groupId>
<artifactId>docker-maven-plugin</artifactId>
<version>0.35.0</version>
<configuration>
<!-- Docker image configuration goes here -->
</configuration>
</plugin>
</plugins>
</build>
In the <configuration>
section, you can define various aspects of your Docker image, such as the base image, exposed ports, environment variables, and more. This configuration will be used by the Docker Maven Plugin to build your image during the Maven build process.
Building Docker Images
Once you have set up the Docker Maven Plugin in your project, you can use it to build Docker images. To do this, you can execute the docker:build
goal from the command line:
mvn docker:build
This will trigger the Docker Maven Plugin to build an image based on the configuration specified in your pom.xml
file. The plugin will use the Docker daemon on your machine to build the image, leveraging the Dockerfile and context provided in the configuration.
Why Use Docker Maven Plugin for Building Images?
Using the Docker Maven Plugin to build images provides several benefits. Firstly, it allows you to define your image configuration directly within your Maven project, making it easier to maintain and version control. Additionally, it integrates seamlessly with the Maven build lifecycle, ensuring that your Docker image is built as part of your standard build process.
Another advantage is that it provides a consistent and reproducible way to build images across different environments. This can be especially useful in a CI/CD pipeline, where you want to ensure that your integration testing environment is consistent regardless of the underlying infrastructure.
Running Integration Tests with Dockerized Environments
One of the key advantages of using the Docker Maven Plugin is the ability to run integration tests against Dockerized environments. By leveraging Docker containers for testing, you can ensure that your tests are running against a consistent and isolated environment, reducing the likelihood of interference from external factors.
To run integration tests against a Dockerized environment, you can use the docker:start
and docker:stop
goals provided by the Docker Maven Plugin. These goals allow you to start and stop Docker containers as part of your Maven build process. Here's an example configuration for running integration tests:
<plugin>
<groupId>io.fabric8</groupId>
<artifactId>docker-maven-plugin</artifactId>
<version>0.35.0</version>
<executions>
<execution>
<id>start-container</id>
<phase>pre-integration-test</phase>
<goals>
<goal>start</goal>
</goals>
</execution>
<execution>
<id>stop-container</id>
<phase>post-integration-test</phase>
<goals>
<goal>stop</goal>
</goals>
</execution>
</executions>
<configuration>
<!-- Docker container configuration goes here -->
</configuration>
</plugin>
By defining the start
goal in the pre-integration-test
phase and the stop
goal in the post-integration-test
phase, you can ensure that the Docker container is started before running integration tests and stopped afterwards. This helps to maintain a clean and isolated environment for your tests.
Why Use Docker Maven Plugin for Integration Testing?
Integrating Docker containers into your integration testing process offers several advantages. Firstly, it provides a consistent and reproducible testing environment, ensuring that your tests yield predictable results across different environments. This can be especially beneficial when collaborating with a distributed team, as everyone can use the same containerized environment for testing.
Additionally, running integration tests against Dockerized environments can help uncover issues related to environment-specific configurations, dependencies, and networking. By encapsulating your test environment in a Docker container, you can identify and address these issues more effectively.
The Closing Argument
The Docker Maven Plugin is a powerful tool for streamlining integration testing in Java applications. By leveraging this plugin, you can easily build and manage Docker images as part of your Maven build process, and run integration tests against Dockerized environments with ease. This approach not only simplifies the setup and configuration of integration testing but also provides a consistent and reproducible environment for testing.
In conclusion, integrating the Docker Maven Plugin into your project can enhance the reliability and efficiency of your integration testing process. By embracing containerization for testing, you can ensure that your tests are running in consistent, isolated environments, leading to more reliable and maintainable test suites.
If you're interested in diving deeper into Docker Maven Plugin, you can explore the official documentation for comprehensive information and advanced usage. Additionally, the official Docker documentation is a valuable resource for understanding Docker concepts and best practices.
Try incorporating the Docker Maven Plugin into your Java project and experience the simplicity and power it brings to integration testing!
Checkout our other articles