Local Containers for Cloud-Native Dev: Overcoming Challenges
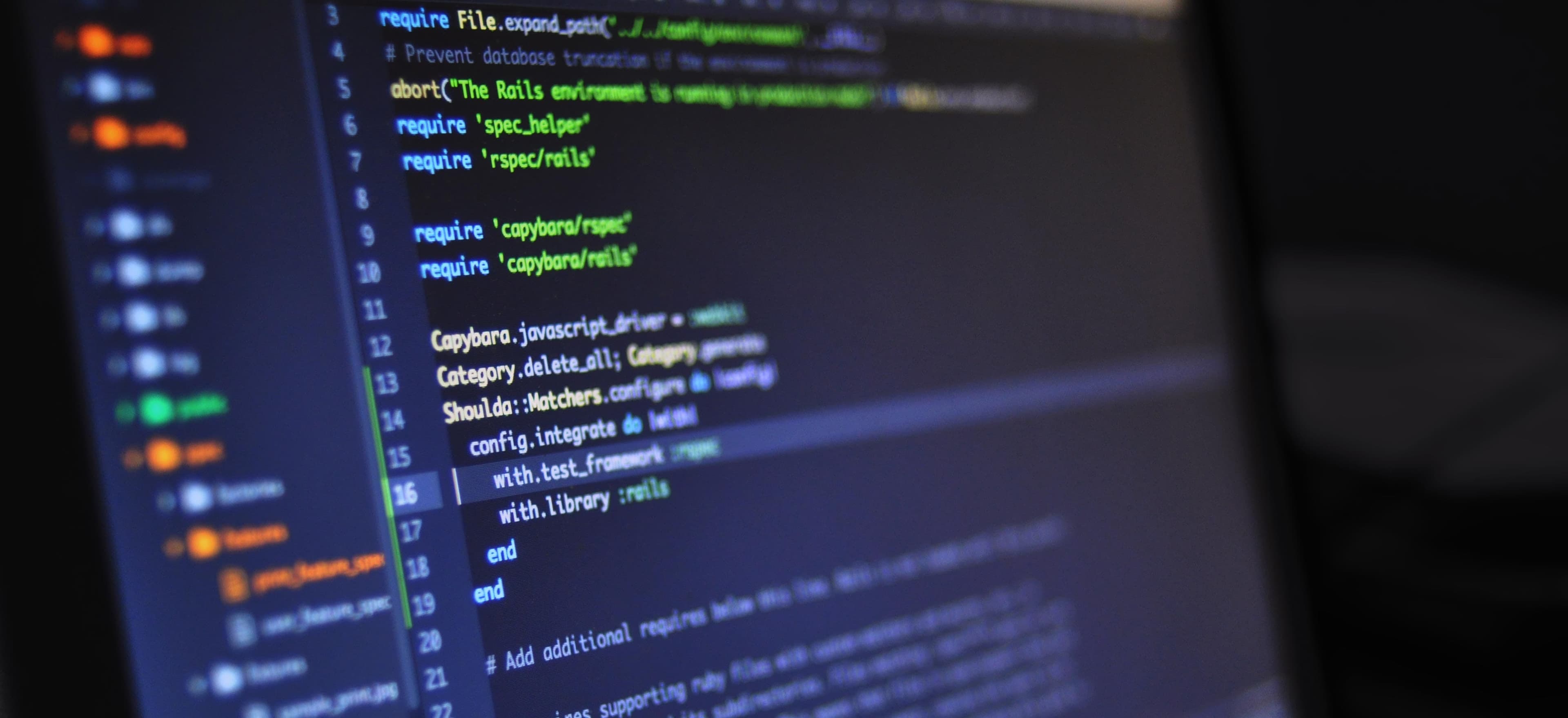
- Published on
How to Use Local Containers for Cloud-Native Java Development
Cloud-native development has become a standard approach for modern software engineering. With the rise in popularity of containerization, the development process is often integrated with containerization tools such as Docker. In this blog post, we will explore the benefits and challenges of using local containers for cloud-native Java development. We will also discuss best practices and tools to overcome these challenges.
Benefits of Local Containers for Cloud-Native Java Development
The use of local containers for cloud-native Java development offers several benefits, including:
-
Consistency: Containers provide a consistent environment across different stages of the development lifecycle, ensuring that the application behaves the same way in development, testing, and production environments.
-
Isolation: Containers encapsulate the application and its dependencies, preventing conflicts with other applications and system libraries.
-
Portability: Containers can be easily moved between different environments, enabling seamless deployment across various cloud platforms and on-premises infrastructure.
-
Scalability: Containers allow developers to easily scale the application horizontally by adding more container instances.
Challenges of Using Local Containers for Cloud-Native Java Development
While local containers offer many benefits, they also present several challenges, especially when developing Java applications:
-
Resource Consumption: Running multiple containers locally can consume a significant amount of system resources, impacting the performance of the development machine.
-
Integration with IDEs: Integrating containerized development with IDEs, such as IntelliJ IDEA or Eclipse, can be challenging and may require additional setup.
-
Networking: Managing networking between containers and the host machine can be complex, especially when dealing with microservices architectures.
-
Data Persistence: Handling data persistence and stateful services within local containers requires careful consideration and configuration.
Overcoming Challenges with Best Practices and Tools
Managing Resource Consumption
To address the issue of resource consumption, developers can utilize tools like Docker Compose to define multi-container applications. Docker Compose allows you to specify the resources allocated to each container, ensuring optimal resource utilization.
Integrating with IDEs
For seamless integration with IDEs, consider using the Jib plugin for Maven or Gradle. Jib allows you to build and package Java applications directly into container images without a Docker daemon or Dockerfile, streamlining the development workflow within the IDE.
Managing Networking
When it comes to networking, tools like Telepresence can be utilized to facilitate local development with remote Kubernetes clusters. Telepresence allows developers to run a single service locally while connecting to the rest of the services running in a remote Kubernetes cluster, simplifying the development and debugging of microservices.
Data Persistence
For managing data persistence within local containers, using Docker volumes is a recommended approach. Docker volumes provide a way to persist data generated by and used by Docker containers, ensuring that data is retained even if the container is stopped or removed.
Using Local Containers for Cloud-Native Java Development: Best Practices
Build Lightweight Java Images
When creating container images for Java applications, it's essential to optimize the image size to improve deployment speed and efficiency. Utilize multi-stage builds to separate the build environment from the runtime environment, ensuring that the final image contains only the necessary artifacts and dependencies.
Example of a multi-stage Dockerfile for a Java application:
# Build stage
FROM maven:3.6.3-jdk-11 AS build
COPY ./ /usr/src/app
WORKDIR /usr/src/app
RUN mvn clean package
# Runtime stage
FROM adoptopenjdk:11-jre-hotspot
COPY --from=build /usr/src/app/target/app.jar /app/
CMD ["java", "-jar", "/app/app.jar"]
In the above example, the build stage uses a Maven image to build the Java application, and the runtime stage uses a lightweight JDK image to run the built application.
Use Environment Variables for Configuration
When working with Java applications in containers, utilize environment variables for configuration. This makes the application more configurable and portable across different environments without modifying the application code or configuration files.
Example of using environment variables in a Dockerfile:
FROM adoptopenjdk:11-jre-hotspot
ENV APP_PORT 8080
ENV DB_HOST localhost
COPY ./target/app.jar /app/
CMD ["java", "-jar", "/app/app.jar"]
In the above example, the application's port and database host are configured using environment variables, allowing for easy customization.
Implement Health Checks and Graceful Shutdown
When running Java applications in containers, implement health checks to ensure the application is ready to receive traffic. Additionally, configure graceful shutdown to allow the application to complete ongoing operations before shutting down, ensuring a clean shutdown process.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.support.SpringBootServletInitializer;
import org.springframework.context.annotation.Bean;
import org.springframework.boot.actuate.health.Health;
import org.springframework.boot.actuate.health.HealthIndicator;
@SpringBootApplication
public class Application extends SpringBootServletInitializer {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
public HealthIndicator healthIndicator() {
return () -> Health.up().build();
}
}
In the above example, a health check endpoint is implemented using Spring Boot Actuator, which can be used to indicate the application's health to the container orchestrator.
Wrapping Up
In conclusion, leveraging local containers for cloud-native Java development brings numerous advantages, including consistency, isolation, portability, and scalability. While challenges such as resource consumption, IDE integration, networking, and data persistence exist, employing best practices and tools such as Docker Compose, Jib, Telepresence, and Docker volumes can help overcome these obstacles. Additionally, following best practices such as building lightweight Java images, using environment variables for configuration, and implementing health checks and graceful shutdowns can further enhance the development process.
By embracing best practices and utilizing the right tools, developers can harness the power of local containers to streamline and enhance their cloud-native Java development workflow, leading to more efficient, scalable, and portable applications.
For more in-depth insights into Java development, containerization, and cloud-native practices, be sure to check out our Java development guide and the Cloud-Native Computing Foundation for the latest industry standards and best practices.
Happy containerizing!
Checkout our other articles