Redis Libraries Showdown: Top Picks for Efficient Coding
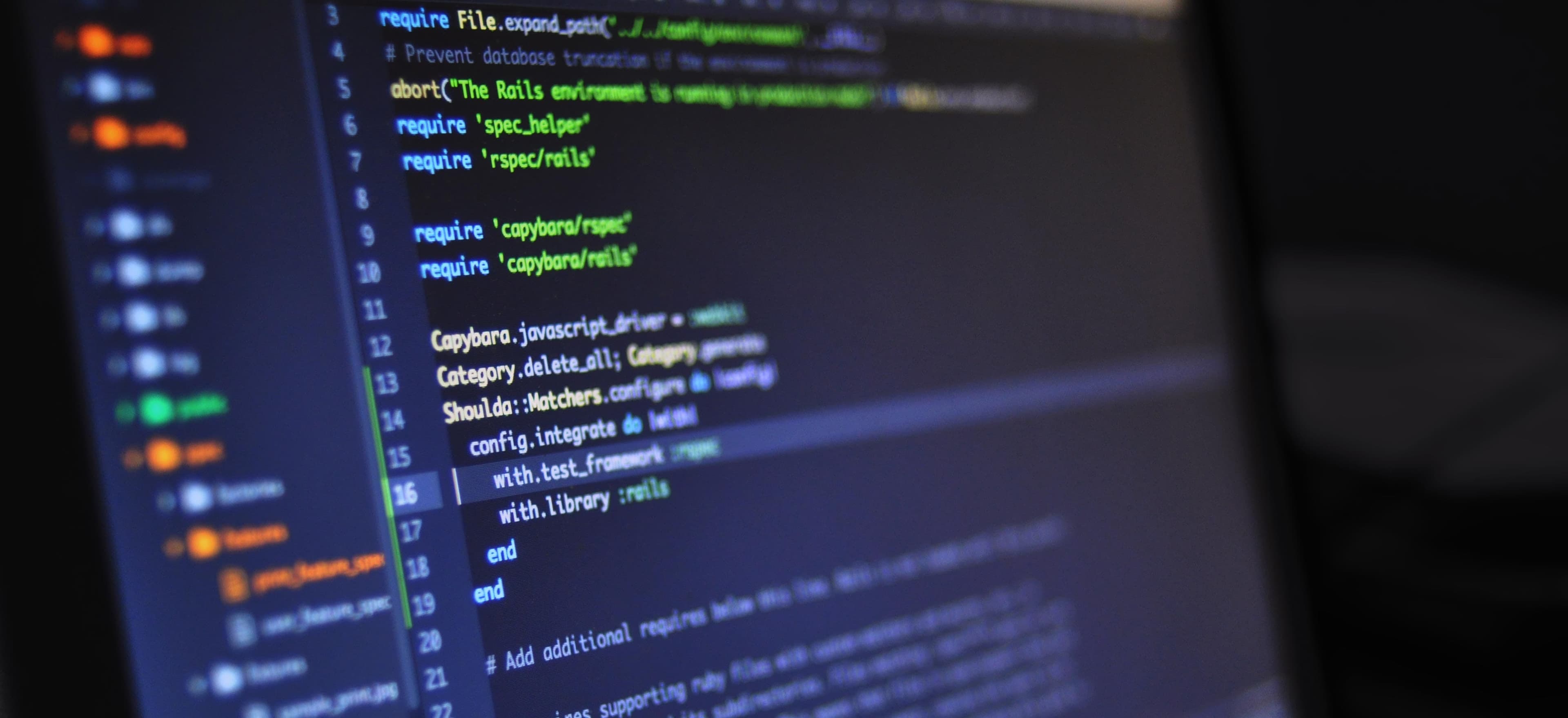
- Published on
Redis Libraries Showdown: Top Picks for Efficient Coding
When it comes to building efficient and scalable Java applications, integrating a reliable and performant data store is paramount. Redis, with its lightning-fast in-memory data storage and versatile data structures, has become a popular choice for developers looking to optimize their applications. In this article, we'll delve into some of the top Redis libraries for Java that can supercharge your application's performance while streamlining your coding process.
Jedis: The Traditional Workhorse
Jedis is a mature and widely-used Java client library for Redis, offering a straightforward and easy-to-use interface for interacting with Redis servers. It provides a low-level, close-to-the-metal interaction with Redis, giving developers direct access to the Redis commands and data types.
Jedis jedis = new Jedis("localhost");
jedis.set("key", "value");
String value = jedis.get("key");
The above code snippet demonstrates the simplicity and familiarity Jedis brings to the table. It allows you to quickly establish a connection with a Redis server and perform basic operations such as setting and getting key-value pairs.
While Jedis provides extensive control and flexibility, it's worth noting that it operates in a synchronous, blocking manner, which might not be suitable for all use cases, particularly those requiring non-blocking I/O.
Lettuce: Embracing Asynchronous Operations
In the world of reactive and non-blocking programming, Lettuce has emerged as a popular choice for integrating Redis with Java applications. Lettuce is an asynchronous Redis client that leverages the power of reactive programming and supports the reactive streams API.
RedisClient client = RedisClient.create("redis://localhost");
StatefulRedisConnection<String, String> connection = client.connect();
RedisCommands<String, String> syncCommands = connection.sync();
syncCommands.set("key", "value");
String value = syncCommands.get("key");
The above code showcases Lettuce's asynchronous nature, where the connection, commands, and operations are performed in a non-blocking manner, making it suitable for high-throughput and responsiveness-critical applications.
Lettuce also integrates seamlessly with popular reactive frameworks such as Project Reactor and RxJava, empowering developers to build robust and efficient reactive applications with Redis as the data store.
Redisson: Feature-Rich and Distributed
Redisson takes a different approach by providing a feature-rich, distributed, and high-performance alternative to traditional Java Redis clients. It abstracts the low-level details of Redis connections and data structures, offering a more developer-friendly and feature-packed API.
Config config = new Config();
config.useSingleServer().setAddress("redis://127.0.0.1:6379");
RedissonClient client = Redisson.create(config);
RMap<String, String> map = client.getMap("myMap");
map.put("key", "value");
String value = map.get("key");
With Redisson, developers can leverage advanced features such as distributed locks, distributed objects, and reactive execution models, all while benefitting from a simple and expressive API.
Choosing the Right Library for Your Project
When it comes to selecting the most suitable Redis library for your Java project, several factors come into play. Consider the nature of your application, its performance requirements, and your familiarity with reactive programming paradigms.
-
If you require utmost control over Redis interactions and are comfortable with a synchronous approach, Jedis might align well with your coding style and requirements.
-
For developers venturing into the realm of reactive and non-blocking programming, seeking high-throughput and responsiveness, Lettuce could be the perfect fit, enabling you to embrace a more modern approach to Redis integration.
-
In scenarios where distributed and feature-rich Redis capabilities are paramount, and a developer-friendly API is desired, Redisson presents a compelling choice.
It's important to evaluate the trade-offs and nuances of each library in the context of your specific project needs, and to consider future scalability and maintainability aspects when making the decision. Additionally, exploring the integration of these libraries within your existing or preferred reactive frameworks can also play a pivotal role in the library selection process.
To Wrap Things Up
Redis libraries play a pivotal role in shaping the efficiency, performance, and maintainability of Java applications leveraging Redis as a data store. Whether it's the classic approach of Jedis, the asynchronous prowess of Lettuce, or the feature-rich capabilities of Redisson, each library brings its own set of strengths to the table.
By understanding the unique features and design philosophies behind these Redis libraries, developers can make informed decisions and effectively optimize their coding process, ultimately leading to more efficient and scalable Java applications.
In conclusion, the right Redis library for your project depends on your specific use case, coding style, and the desired feature set. With the plethora of options available, it's essential to weigh the strengths and trade-offs of each library to make an informed choice that aligns with your application's performance and scalability objectives.
To explore more about Java and Redis integration, check out the Redis documentation on Java client libraries and gain deeper insights into the best practices and considerations for leveraging Redis in Java applications.
Checkout our other articles