Mastering Stateless Sessions in Spring for Multi-Tenant Apps
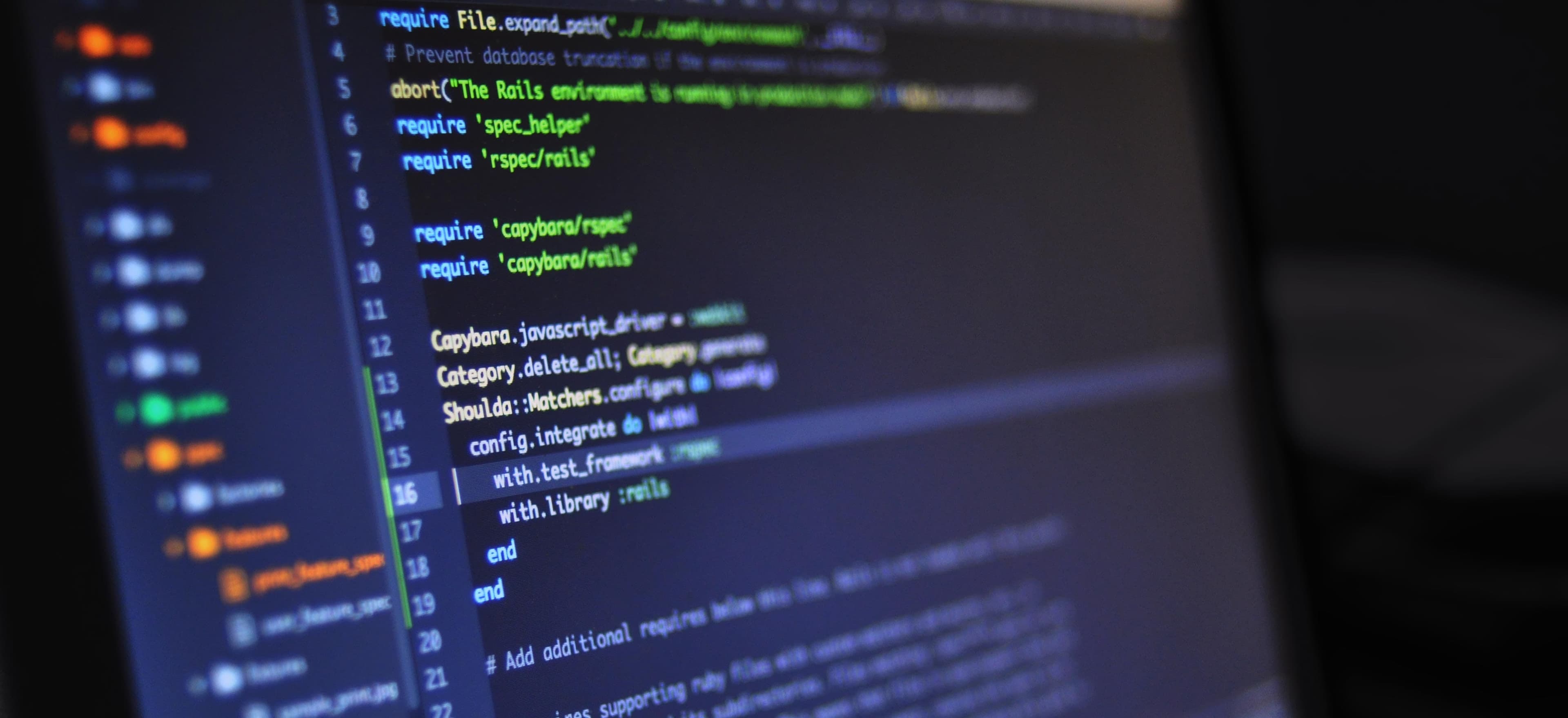
- Published on
Mastering Stateless Sessions in Spring for Multi-Tenant Apps
In a multi-tenant application, a single instance of the software serves multiple customers, each being a tenant. In such scenarios, efficient handling of user sessions becomes crucial, especially ensuring that user sessions are stateless. This approach allows for better scalability, high availability, and improved performance. In this blog post, we will delve into the concept of stateless sessions in Spring and explore how to master them for multi-tenant applications.
Understanding the Importance of Stateless Sessions in Multi-Tenant Apps
In a multi-tenant environment, traditional session management with server-side session storage introduces challenges related to scalability and performance. With stateful sessions, the server needs to maintain user session data, leading to potential bottlenecks and increased resource utilization, especially when serving multiple tenants concurrently.
Stateless sessions, on the other hand, eliminate the need for server-side session storage, as each request from the client contains all the necessary information for the server to process it. This approach aligns perfectly with the principles of microservices and cloud-native architectures, allowing for easier horizontal scaling and efficient resource utilization.
Implementing Stateless Sessions with Spring
Utilizing JWT for Stateless Authentication
JSON Web Token (JWT) is a popular choice for implementing stateless authentication in Spring applications. JWTs are compact, self-contained tokens that can carry information about the user, along with additional metadata that can be validated and trusted. This makes JWT a suitable candidate for achieving stateless sessions in multi-tenant apps.
Let's take a look at an example of how JWT can be used for stateless authentication in Spring:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers("/api/public/**").permitAll()
.anyRequest().authenticated()
.and()
.addFilter(new JwtAuthenticationFilter(authenticationManager()))
.addFilter(new JwtAuthorizationFilter(authenticationManager()));
}
@Bean
public JwtConfig jwtConfig() {
return new JwtConfig();
}
}
In this configuration, we are utilizing Spring Security to define the security constraints, allowing public access to certain API endpoints while requiring authentication for others. The JwtAuthenticationFilter
and JwtAuthorizationFilter
are responsible for processing JWTs and validating the user's identity for each request.
Leveraging ThreadLocal for Tenant Context
In a multi-tenant environment, it's crucial to maintain the context of the tenant associated with each user's request. One way to achieve this is by leveraging ThreadLocal to store the tenant information and propagate it throughout the request processing pipeline.
Let's explore how ThreadLocal can be used to manage the tenant context in a multi-tenant Spring application:
public class TenantContext {
private static final ThreadLocal<String> currentTenant = new ThreadLocal<>();
public static void setTenantId(String tenantId) {
currentTenant.set(tenantId);
}
public static String getTenantId() {
return currentTenant.get();
}
public static void clear() {
currentTenant.remove();
}
}
In this example, the TenantContext
class provides static methods to set and get the current tenant ID as a ThreadLocal variable. Before processing each request, the tenant ID can be extracted from the user's credentials and set in the TenantContext
. Throughout the request processing, any service or repository classes can retrieve the current tenant ID from the TenantContext
, ensuring that tenant-specific data is accessed within the context of the authenticated user.
Best Practices for Managing Stateless Sessions in Multi-Tenant Apps
As you dive deeper into handling stateless sessions in multi-tenant applications, keep these best practices in mind to ensure a robust and secure implementation:
1. Centralized Token Management
Maintain a centralized approach to token management, including issuing, validation, and revocation. This allows for consistent handling of JWTs across all tenant-specific instances of the application.
2. Secure Token Generation
Ensure that JWTs are generated securely, with appropriate cryptographic algorithms and key management practices. The integrity and confidentiality of the token payload are paramount for maintaining the stateless nature of the sessions.
3. Tenant Isolation
Enforce strict isolation of tenant data and resources to prevent any accidental or unauthorized leakage between tenants. Each user's request should strictly adhere to the context of their associated tenant without any cross-tenant information exposure.
4. Scalability Considerations
Design your stateless session management strategy with scalability in mind. As the application scales to serve a larger number of tenants, the session handling mechanism should gracefully accommodate the increased load without compromising performance.
Closing the Chapter
Mastering stateless sessions in Spring for multi-tenant applications requires a solid understanding of stateless authentication mechanisms like JWT and the effective management of tenant context throughout the request processing pipeline. By implementing best practices and leveraging the capabilities of Spring Security and ThreadLocal, you can achieve a robust and scalable solution for managing stateless sessions in a multi-tenant environment.
In the rapidly evolving landscape of cloud-native architectures and microservices, the ability to handle stateless sessions in a multi-tenant setting is a valuable skill for any Java developer. Embracing these concepts and practices will not only enhance the performance and scalability of your applications but also contribute to a more secure and efficient user experience.
For further reading on stateless session management and multi-tenant architecture, check out the official Spring Security documentation and multi-tenancy best practices.
Start mastering stateless sessions in Spring today, and elevate your ability to build robust, scalable, and secure multi-tenant applications!
Checkout our other articles