Revitalizing Legacy Code: Default Methods Unleashed!
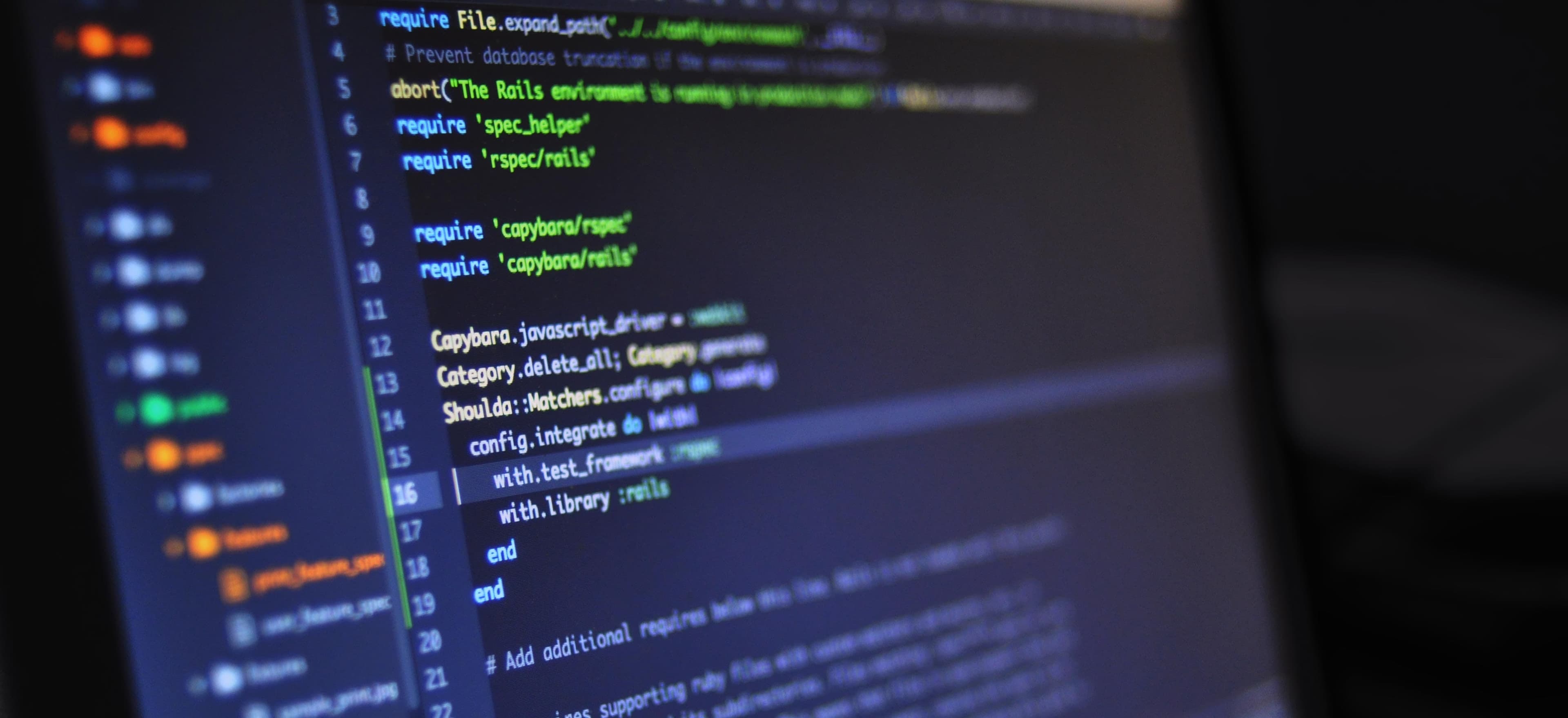
- Published on
Revitalizing Legacy Code: Default Methods Unleashed!
As a Java developer, working with legacy code can be both challenging and rewarding. While the thought of refactoring or updating legacy code may seem daunting, it's important to embrace modern Java features to improve code readability, maintainability, and performance. In this article, we'll explore how default methods in Java interfaces can breathe new life into legacy code, making it more robust and adaptable to evolving requirements.
The Challenge of Legacy Code
Legacy code often carries the weight of history and accumulated technical debt. It may lack the flexibility to accommodate new features and changes, leading to complex workarounds and patches that further complicate the codebase. Introducing new functionality without disrupting existing code requires a careful approach that leverages Java's evolving features.
Enter Default Methods
One of the significant enhancements introduced in Java 8 was the addition of default methods in interfaces. Default methods enable developers to add new methods to interfaces without breaking existing implementations. This feature is a game-changer when it comes to updating legacy code, as it allows us to augment interfaces with new methods while maintaining backward compatibility with classes that implement those interfaces.
Refactoring Legacy Code with Default Methods
Let's consider a hypothetical scenario where we have a legacy Calculator
interface with a calculate
method. To enhance the functionality of the Calculator
interface without impacting existing implementations, we can introduce a default method description
that provides additional context for the calculator operation.
public interface Calculator {
int calculate(int a, int b);
default String description() {
return "This interface provides basic arithmetic operations";
}
}
By adding the description
default method, we ensure that all existing implementations of Calculator
remain unaffected, while new implementations can benefit from the additional descriptive capability.
Why Use Default Methods?
Default methods serve as a powerful tool for injecting new behavior into existing interfaces. They enable us to extend interfaces without forcing all implementing classes to provide concrete implementations for the new methods. This is particularly valuable when dealing with legacy code, as it allows gradual enhancement of interfaces without immediately breaking existing code.
Embracing Backward Compatibility
Java's commitment to backward compatibility is a crucial factor in the widespread adoption of default methods. Existing codebases can incrementally integrate new interface methods without the need to modify every implementing class. This approach eases the burden of updating legacy code, as it aligns with the principles of gradual refinement and non-disruptive evolution.
Pitfalls to Avoid
While default methods offer a flexible approach to enhancing interfaces, it's essential to use them judiciously. Overuse of default methods can lead to bloated interfaces with scattered behavior, potentially muddying the design and conceptual clarity of the codebase. It's important to strike a balance between extending interfaces sensibly and preserving their cohesion and purpose.
Compatibility with Libraries and Frameworks
When introducing default methods into an existing interface, it's crucial to consider compatibility with external libraries and frameworks. While most modern libraries and frameworks handle default methods seamlessly, it's prudent to verify compatibility, especially when integrating with older components or third-party dependencies.
Default Methods and Multiple Inheritance
One of the long-standing limitations of Java interfaces was the inability to contain method implementations, leading to the "diamond problem" when implementing multiple interfaces with conflicting method signatures. Default methods mitigate this issue by providing a mechanism for interfaces to incorporate method implementations without introducing ambiguity or conflicts.
Migration Strategy for Legacy Codebases
Migrating legacy code to leverage default methods involves a systematic approach that aligns with the overall code maintenance and evolution strategy. It's crucial to identify opportunities where default methods can streamline existing interfaces without disrupting the codebase. Thorough testing and validation are essential to ensure that the introduction of default methods does not introduce unforeseen issues in legacy components.
To Wrap Things Up
Default methods in Java interfaces offer a compelling approach to enhancing legacy code without sacrificing backward compatibility. By judiciously introducing default methods, developers can augment interfaces with new functionality while preserving the stability and integrity of existing code. Embracing modern Java features empowers us to revitalize legacy codebases, making them more adaptable, maintainable, and aligned with contemporary development practices.
In conclusion, default methods are much more than just a convenient addition to Java interfaces – they are a powerful tool for modernizing legacy code and ensuring its relevance in evolving software environments. As we navigate the landscape of legacy systems and evolving requirements, default methods stand out as a versatile and pragmatic solution for breathing new life into aging codebases.
So, the next time you're faced with the challenge of revitalizing legacy code, remember the untapped potential of default methods – they just might be the key to unlocking a new era of flexibility and extensibility in your codebase.
Happy coding!