Preventing ConcurrentModificationException in Java Collections
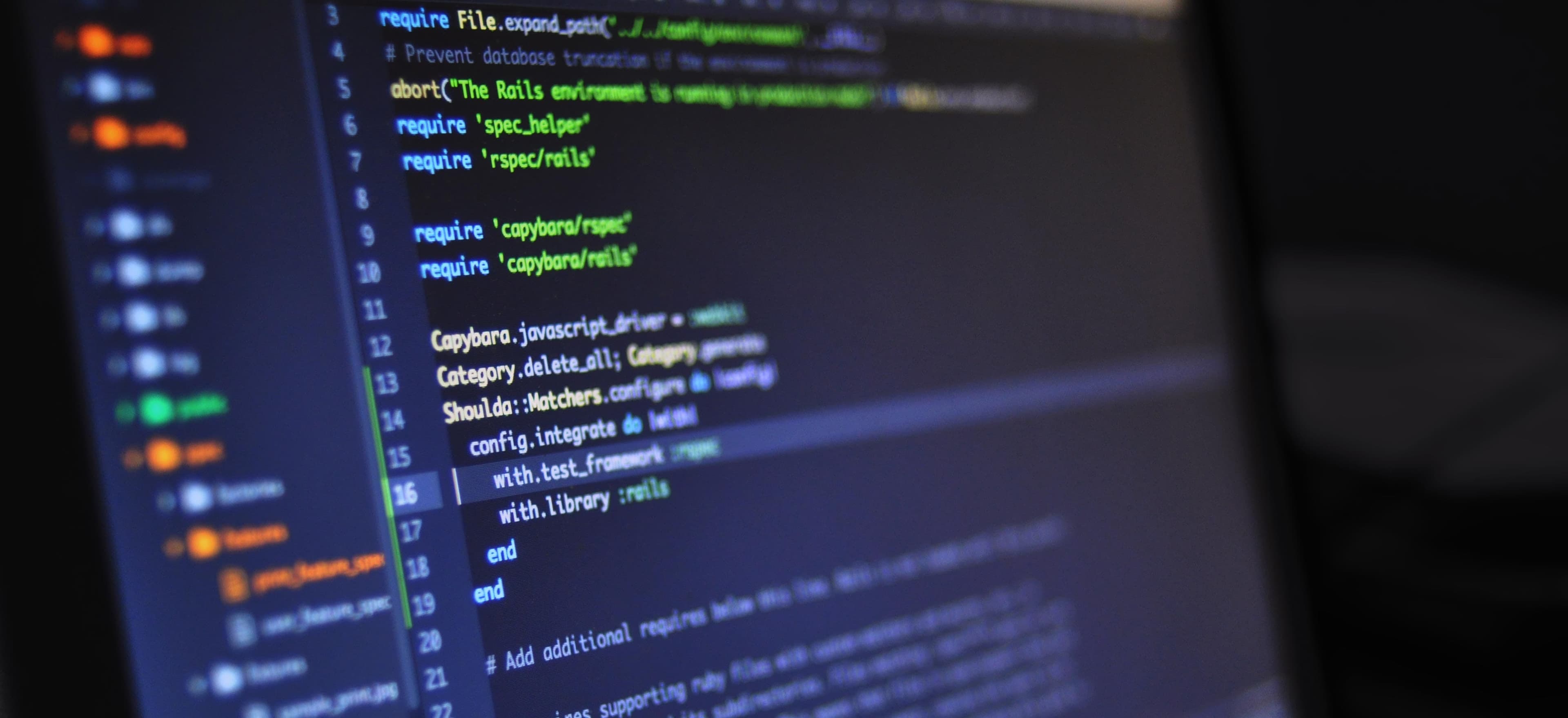
- Published on
Preventing ConcurrentModificationException in Java Collections
When working with Java collections, it's crucial to handle concurrent modifications to avoid the dreaded ConcurrentModificationException
. This exception occurs when a collection is modified while it's being iterated, leading to unpredictable behavior and potential data corruption. In this article, we'll explore the causes of this exception and discuss strategies to prevent it, ensuring the stability and consistency of your Java applications.
Understanding ConcurrentModificationException
The ConcurrentModificationException
is thrown to indicate that an operation being performed on a collection is not valid due to concurrent modification by another thread. This commonly happens during iteration, such as with enhanced for loops or explicit iterators, where a collection is modified while it's being traversed.
Root Causes of ConcurrentModificationException
The primary cause of this exception is the modification of a collection while it's being iterated. This can happen when:
- Adding or removing elements from the collection during iteration
- Changing the structure of the collection (e.g., adding or removing elements) without using proper synchronization
Preventing ConcurrentModificationException
1. Use Iterator's remove() Method
When iterating over a collection, use the iterator's remove()
method instead of the collection's remove()
method to avoid ConcurrentModificationException
. The iterator's remove()
method removes the last element that was returned by the next()
method during the iteration.
List<String> list = new ArrayList<>();
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String element = iterator.next();
if (condition) {
iterator.remove(); // Use iterator's remove method
}
}
Using the iterator's remove()
method ensures that the iteration and modification are synchronized, preventing concurrent modification issues.
2. Utilize Synchronized Collections
Java provides synchronized wrappers for various collection types, such as Collections.synchronizedList()
and Collections.synchronizedMap()
. These wrappers encapsulate the original collection and provide synchronized methods to ensure thread safety.
List<String> syncList = Collections.synchronizedList(new ArrayList<>());
By using synchronized collections, you can avoid concurrent modification issues caused by simultaneous access from multiple threads.
3. Employ Concurrent Collections
Java also offers specialized concurrent collections in the java.util.concurrent
package, such as ConcurrentHashMap
and CopyOnWriteArrayList
. These concurrent collections are designed to handle concurrent modifications gracefully.
ConcurrentMap<String, Integer> concurrentMap = new ConcurrentHashMap<>();
Concurrent collections utilize advanced concurrency control mechanisms to allow safe access and modifications from multiple threads without throwing ConcurrentModificationException
.
4. Leverage Java Streams
When processing collections in a single-threaded environment, utilizing Java Streams can provide a concise and safe way to perform operations without encountering concurrent modification issues.
List<String> list = new ArrayList<>();
list.removeIf(element -> condition);
Using stream operations like removeIf()
directly on the collection can help avoid the need for explicit iteration, reducing the likelihood of concurrent modification.
5. Employ Explicit Synchronization
If you're working with custom data structures or need fine-grained control over synchronization, employing explicit synchronization using synchronized
blocks or locks can prevent concurrent modification issues.
List<String> customList = new ArrayList<>();
synchronized (customList) {
// Perform operations on the custom list within the synchronized block
}
By synchronizing access to the collection, you can ensure that modifications are serialized and don't interfere with ongoing iterations or other modifications.
In Conclusion, Here is What Matters
In the world of Java collections, it's essential to be mindful of concurrent modifications and the potential for ConcurrentModificationException
. By leveraging proper iteration techniques, synchronized and concurrent collections, Java Streams, and explicit synchronization, you can effectively prevent this exception and promote the stability and integrity of your applications.
Remember, understanding how modifications and iterations interact is key to writing robust, concurrent-safe code when working with Java collections. By following these best practices, you can confidently handle collections without the fear of encountering ConcurrentModificationException
.
For further reading, you can explore concurrent collections in the Java documentation and advanced synchronization techniques in Java.