Streamline Your Java Apps with Apache Ignite: A Guide
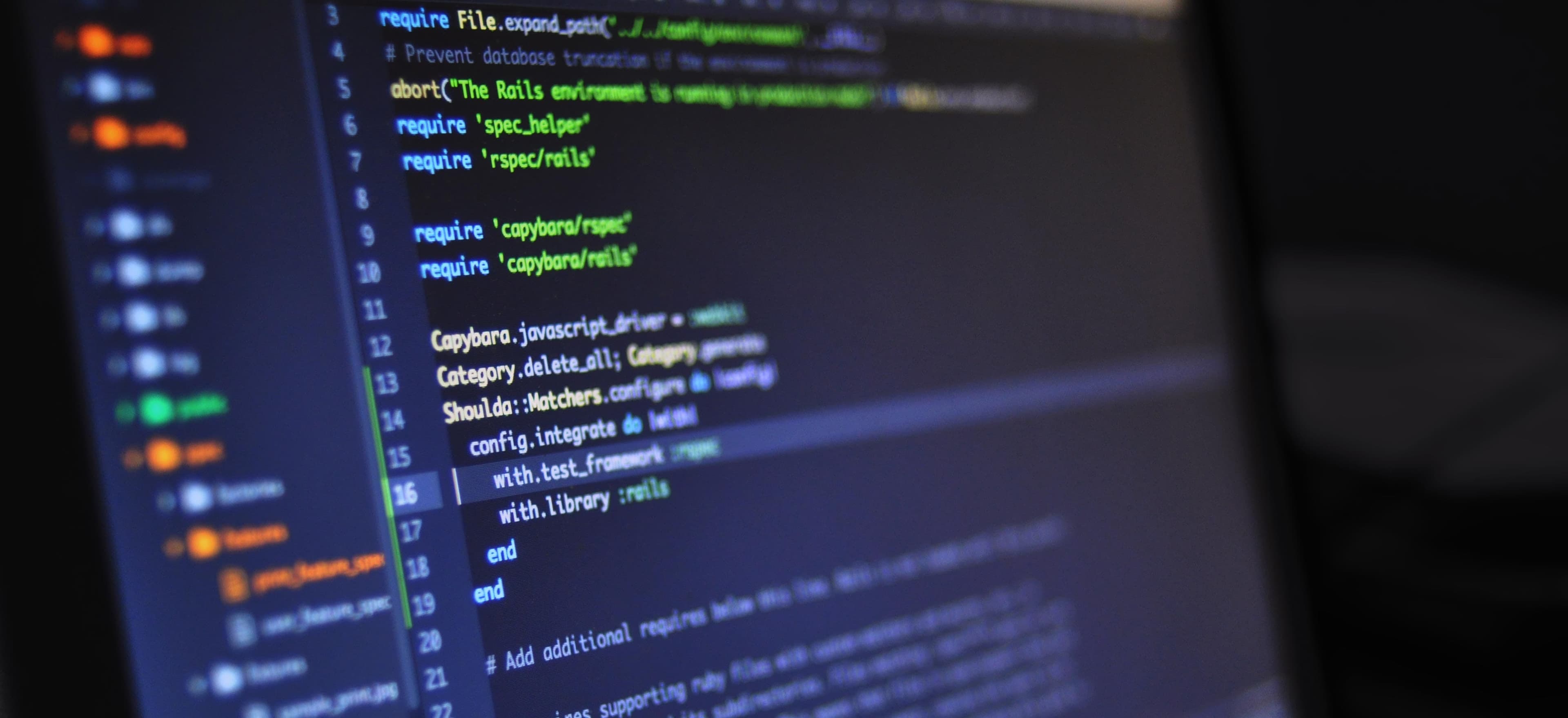
- Published on
Streamline Your Java Apps with Apache Ignite: A Guide
In today’s fast-paced digital world, businesses need to deliver high-performing, scalable, and resilient applications to meet customer demands. Java has been a reliable choice for building these applications for many years. However, as data volumes grow and real-time processing becomes essential, traditional database architectures are often unable to keep up.
Enter Apache Ignite, a memory-centric distributed database, caching, and processing platform. By leveraging Ignite, Java developers can enhance their applications with in-memory data grids, distributed SQL queries, and compute capabilities.
In this guide, we will dive into the powerful features of Apache Ignite and demonstrate how it can be seamlessly integrated into your Java applications to improve performance, scalability, and fault tolerance.
Why Apache Ignite?
Apache Ignite provides a unified platform that integrates seamlessly with Java applications, offering in-memory speed and unlimited horizontal scalability. It is built to handle large datasets with real-time performance, making it an ideal solution for modern, data-intensive applications such as e-commerce platforms, financial systems, and IoT (Internet of Things) networks.
Moreover, Ignite’s distributed architecture allows it to be deployed across a cluster of machines, providing fault tolerance and high availability. This means that even in the event of node failures, the cluster remains operational, ensuring uninterrupted service.
Getting Started with Apache Ignite in Java
To kick things off, let's explore the basic setup for integrating Apache Ignite into a Java application.
Step 1: Add Apache Ignite Dependencies
First, you need to add the Apache Ignite dependencies in your project's pom.xml
(if you're using Maven) or build file:
<dependencies>
<!-- Apache Ignite dependencies -->
<dependency>
<groupId>org.apache.ignite</groupId>
<artifactId>ignite-core</artifactId>
<version>2.8.1</version>
</dependency>
<!-- Add other Ignite modules as per your requirements -->
</dependencies>
Step 2: Create an Ignite Configuration
Next, you need to create an Ignite configuration. Here's a simple example of configuring Ignite with default settings:
IgniteConfiguration cfg = new IgniteConfiguration();
Step 3: Start an Ignite Node
Now, let's start an Ignite node using the configuration we created:
try (Ignite ignite = Ignition.start(cfg)) {
// Node has started
}
By following these steps, you have successfully set up an Apache Ignite node within your Java application. However, this is just the beginning. Let’s delve into some of the key features that make Apache Ignite an invaluable asset for Java developers.
Key Features of Apache Ignite
1. In-Memory Data Grid
Apache Ignite provides a distributed, in-memory key-value store that can be seamlessly integrated into Java applications. By storing data in memory, Ignite enables high-speed access to frequently accessed datasets, significantly improving application performance. This is particularly beneficial for use cases that require low-latency data access, such as real-time analytics and transaction processing.
2. Distributed SQL Queries
With Ignite, you can execute SQL queries across the distributed dataset, allowing you to perform complex analytical operations on large volumes of data with ease. This eliminates the need to manually aggregate data from different nodes, simplifying the development of data-intensive applications.
Let's take a look at a simple example of executing a distributed SQL query in Apache Ignite:
SqlFieldsQuery sql = new SqlFieldsQuery("SELECT * FROM Person WHERE age > ?");
try (QueryCursor<List<?>> cursor = igniteCache.query(sql.setArgs(30))) {
for (List<?> row : cursor)
System.out.println("Person: " + row.get(0) + ", Age: " + row.get(1));
}
In this example, we execute a SQL query to retrieve all Person records where age is greater than 30. The query is distributed across the dataset, and the results are aggregated seamlessly.
3. Compute Grid
One of the standout features of Apache Ignite is its ability to distribute computations across the cluster. This enables parallel processing of tasks, making it ideal for scenarios that involve heavy computational workloads, such as machine learning algorithms and complex calculations. By leveraging Ignite’s compute grid, Java applications can achieve significant performance gains when processing large-scale data.
Let's illustrate the use of Ignite’s compute grid with a simple example:
IgniteCompute compute = ignite.compute();
compute.broadcast(() -> {
// Perform computation on each cluster node
});
In this example, the broadcast
method distributes the computation to all nodes within the cluster, allowing parallel execution of the specified task.
My Closing Thoughts on the Matter
In conclusion, Apache Ignite offers a compelling solution for Java developers looking to enhance the performance, scalability, and fault tolerance of their applications. By seamlessly integrating Ignite into Java applications, developers can leverage its in-memory data grid, distributed SQL queries, and compute capabilities to address the demands of today’s data-intensive environments.
In this guide, we've only scratched the surface of Apache Ignite's capabilities. As you continue to explore and incorporate Apache Ignite into your Java applications, you'll discover even more powerful features that can elevate your applications to the next level of performance and scalability.
To delve deeper into the world of Apache Ignite, and learn about its advanced features and use cases, check out the official Apache Ignite documentation. Happy coding!