Java 8's Optional: Solving or Creating Null Problems?
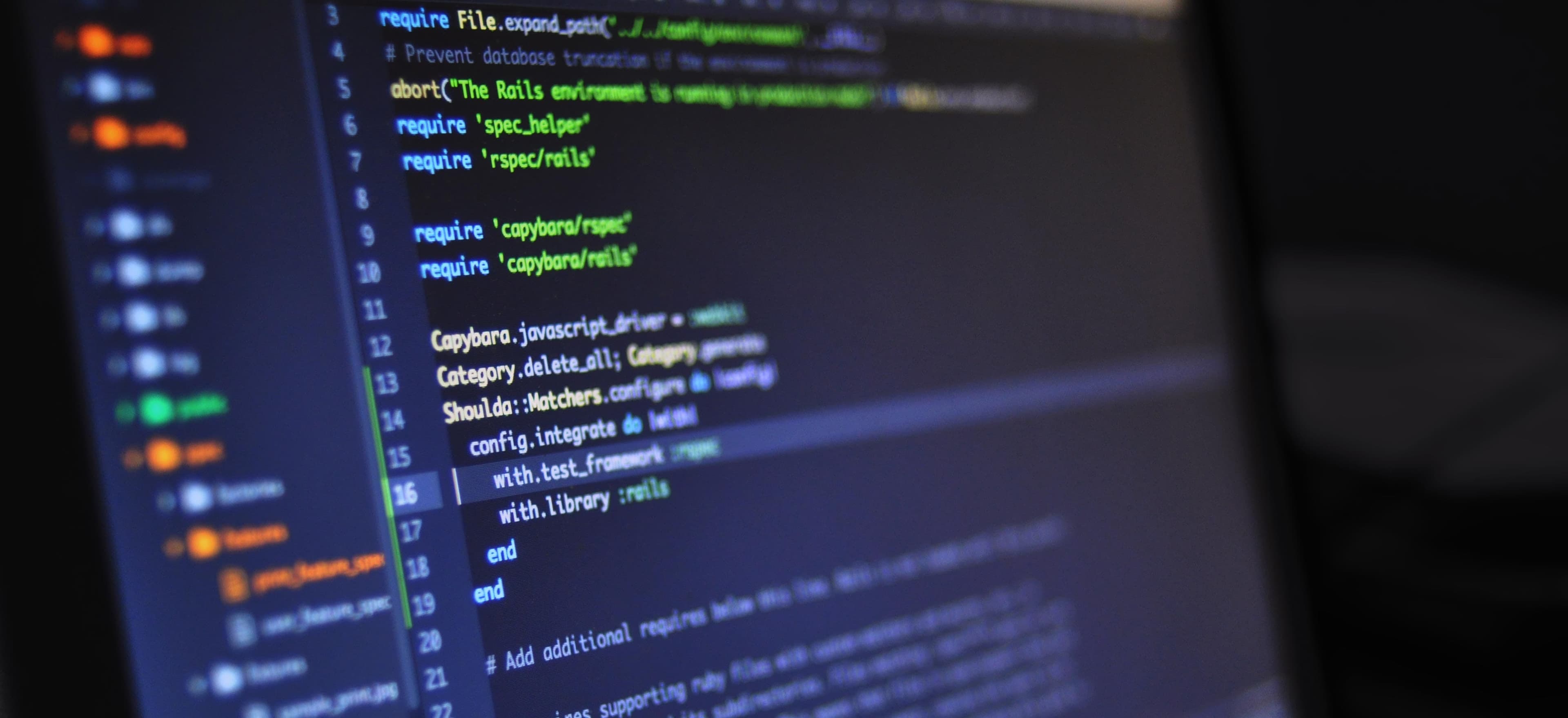
- Published on
Java 8's Optional: Solving or Creating Null Problems?
In Java, dealing with null values can be a common source of bugs and errors. Java 8 introduced the Optional
class to address the issue of null references and provide a more expressive and safer way to represent optional values. However, the use of Optional
has been a topic of debate, with some proponents touting its benefits and others cautioning against its misuse. In this post, we will delve into the features of Java 8's Optional
, discuss its best practices, and explore whether it truly solves the problem of null references or introduces new complexities.
Understanding the Problem of Null References
Before Java 8, handling null references often led to NullPointerExceptions, which are notorious for causing application crashes and unexpected behavior. Consider the following typical scenario:
String name = user.getName();
int length = name.length(); // NullPointerException if name is null
In this case, if user.getName()
returns null, invoking length()
on it will result in a NullPointerException. To avoid this, developers typically write defensive code using if-else checks to ensure that null references are handled properly, which can make the codebase more verbose and error-prone.
Introducing Java 8's Optional
Java 8's Optional
is a container object that may or may not contain a non-null value. It provides methods for handling the presence or absence of a value without needing explicit null checks. The use of Optional
aims to encourage developers to be more explicit about the possibility of a value being absent and to handle it accordingly.
Creating an Optional
You can create an Optional
using its static methods:
Optional<String> optionalName = Optional.of("John");
Optional<String> emptyOptional = Optional.empty();
The of
method creates an Optional
containing a non-null value, while the empty
method creates an empty Optional
.
Retrieving the Value
To retrieve the value from an Optional
, you can use methods like get()
or orElse()
:
String name = optionalName.get(); // Throws NoSuchElementException if empty
String defaultValue = emptyOptional.orElse("Default");
Using get()
directly is discouraged as it can lead to NoSuchElementException if the Optional
is empty. Instead, the orElse
method allows you to provide a default value to be used if the Optional
is empty, thus eliminating the need for explicit null checks.
Conditional Execution
You can perform actions based on the presence or absence of a value using methods like ifPresent
or orElseGet
:
optionalName.ifPresent(val -> System.out.println("Name: " + val));
String processedValue = emptyOptional.orElseGet(() -> performExpensiveOperation());
The ifPresent
method allows you to execute a consumer if the Optional
is non-empty, while orElseGet
enables lazy evaluation of a fallback value, which is useful for expensive computations.
Best Practices and Common Pitfalls
While Java 8's Optional
offers a more explicit and safer way to handle optional values, it is essential to use it judiciously to avoid introducing unnecessary complexity. Here are some best practices and common pitfalls to consider:
Use Case: Return Types
Optional
is suitable as a return type when a method may legitimately return a null value, indicating an absence of value, such as when searching for an item in a collection. It clarifies the intention and forces callers to handle the absence of the value explicitly.
public Optional<User> findUserById(Long id) {
// ...
}
Avoid Nesting Optionals
Avoid nesting Optional
types as it can lead to cumbersome code and reduce readability. Instead, consider using methods like flatMap
to streamline operations on nested Optional
instances.
Optional<Optional<String>> nestedOptional = Optional.of(Optional.of("value"));
String value = nestedOptional.flatMap(Function.identity()).orElse("default");
Embrace Method Chaining
Leverage method chaining to perform sequential operations on Optional
instances, which can lead to more concise and readable code.
String result = optionalName.map(String::toUpperCase).orElse("Default");
Beware of Unnecessary Wrapping
Avoid wrapping non-null values in Optional
unnecessarily, as it can counteract the original intention of Optional
and introduce verbosity.
Optional<String> unnecessaryOptional = Optional.ofNullable("value"); // Unnecessary wrapping
Consider Legacy Code and APIs
When working with legacy code or third-party APIs that return null, carefully evaluate the use of Optional
to avoid conflicts and maintain consistency.
Final Thoughts
Java 8's Optional
provides a more structured approach to dealing with potentially absent values and aims to reduce the likelihood of null pointer exceptions. When used appropriately, Optional
can enhance code clarity and help developers explicitly handle optional values. However, it is crucial to apply Optional
judiciously, considering the context and potential trade-offs, to prevent unnecessary complexity and maintain code readability.
In summary, Java 8's Optional
is a powerful tool for handling optional values, but like any tool, its effectiveness depends on how it is used. By following best practices and understanding its limitations, developers can leverage Optional
to mitigate null reference issues and write more robust and expressive code.
To deepen your understanding of Java 8's Optional, you can explore the official Java documentation and hands-on tutorials like Baeldung's insightful guide on Java Optional Best Practices.