Solving Common Hurdles with Spring Cloud Zuul Proxy
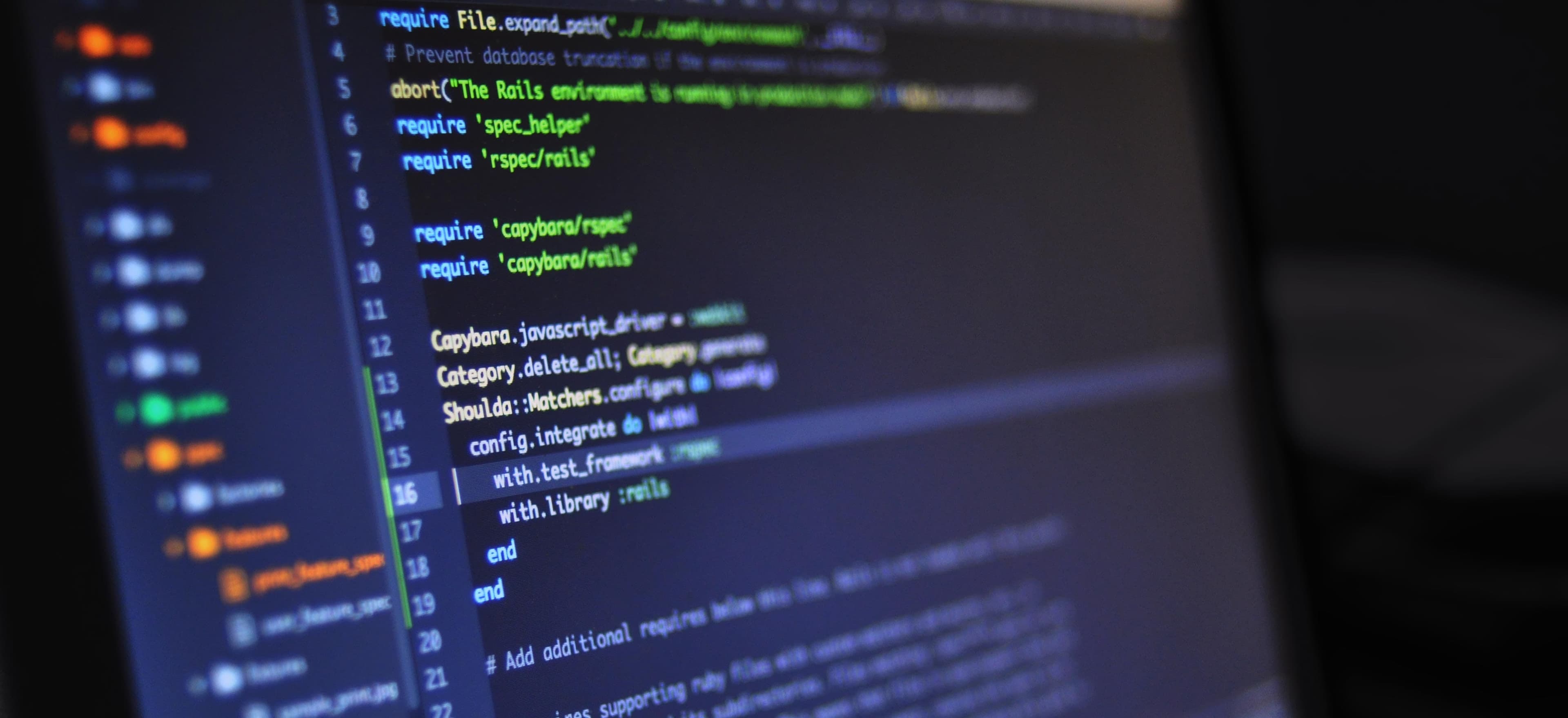
- Published on
Solving Common Hurdles with Spring Cloud Zuul Proxy
As more and more companies embrace microservices architecture, the need for efficient and scalable service-to-service communication becomes increasingly critical. This is where Spring Cloud Zuul Proxy comes into play, offering a robust solution for dynamic routing, monitoring, resiliency, and security. In this blog post, we will delve into some common hurdles encountered while working with Spring Cloud Zuul Proxy and how to effectively address them.
Understanding Spring Cloud Zuul Proxy
Spring Cloud Zuul is a dynamic router and server-side load balancer from Netflix. It provides a single entry point for all service requests, acting as a gateway application that handles all ingress traffic into the microservices system. Zuul also enables dynamic routing, security, monitoring, and resiliency. Leveraging Spring Cloud Netflix, Zuul seamlessly integrates with the Spring Cloud ecosystem, making it a popular choice for microservices architectures.
Hurdle #1: Configuration and Routing
One common hurdle when working with Spring Cloud Zuul is efficiently configuring routing rules for incoming service requests. It's essential to understand how to define routes and microservice mappings effectively.
Solution:
Utilize the application.yml
file to define routes and their respective service mappings. For example:
zuul:
routes:
customers:
path: /mycustomers/**
serviceId: customer-service
In this example, all requests with the pattern /mycustomers/**
will be routed to the customer-service
service.
Why: This approach allows for centralized and flexible route configuration, making it easier to manage route mappings for various services within the application.
Hurdle #2: Handling Cross-Cutting Concerns
Another hurdle that often arises is the need to implement cross-cutting concerns such as logging, authentication, and rate limiting across all services routed through Zuul.
Solution:
Utilize Zuul filters to implement cross-cutting concerns. For example, a Zuul pre-filter can be used for authentication before the request is routed to the respective service, while a post-filter can be employed for logging responses.
@Component
public class AuthFilter extends ZuulFilter {
@Override
public String filterType() {
return "pre";
}
@Override
public int filterOrder() {
return 1;
}
@Override
public boolean shouldFilter() {
// Add your logic for when to run this filter
return true;
}
@Override
public Object run() {
// Add your authentication logic here
return null;
}
}
Why: Implementing filters allows for the encapsulation of cross-cutting concerns, providing a clean and efficient way to apply these concerns uniformly across all routed services.
Hurdle #3: Load Balancing and Resiliency
Ensuring load balancing and resiliency features are properly set up to handle service failures and distribute traffic efficiently is crucial.
Solution:
Utilize Spring Cloud Ribbon and Hystrix for client-side load balancing and fault tolerance. By annotating the service with @EnableCircuitBreaker
and @RibbonClient
, Zuul can leverage Ribbon for load balancing and Hystrix for fault tolerance.
@RibbonClient(name = "customer-service")
@EnableCircuitBreaker
public class MyZuulProxyApplication {
// Application configuration
}
Why: Integrating Ribbon and Hystrix provides an effective way to introduce load balancing and resiliency into the system, enhancing its overall stability and fault tolerance.
The Last Word
Solving common hurdles with Spring Cloud Zuul Proxy involves effectively configuring routing, implementing cross-cutting concerns, and ensuring load balancing and resiliency features are in place. By addressing these challenges, developers can ensure a robust and efficient microservices architecture powered by Spring Cloud Zuul Proxy.
To further explore Spring Cloud Zuul Proxy and its capabilities, refer to the official documentation and Spring Cloud Netflix GitHub repository.
In conclusion, Spring Cloud Zuul Proxy offers a powerful solution for managing service-to-service communication in a microservices architecture, and by effectively addressing common hurdles, developers can harness its full potential for building resilient and scalable systems.