Boosting Performance: Optimizing Microservices with API Gateways
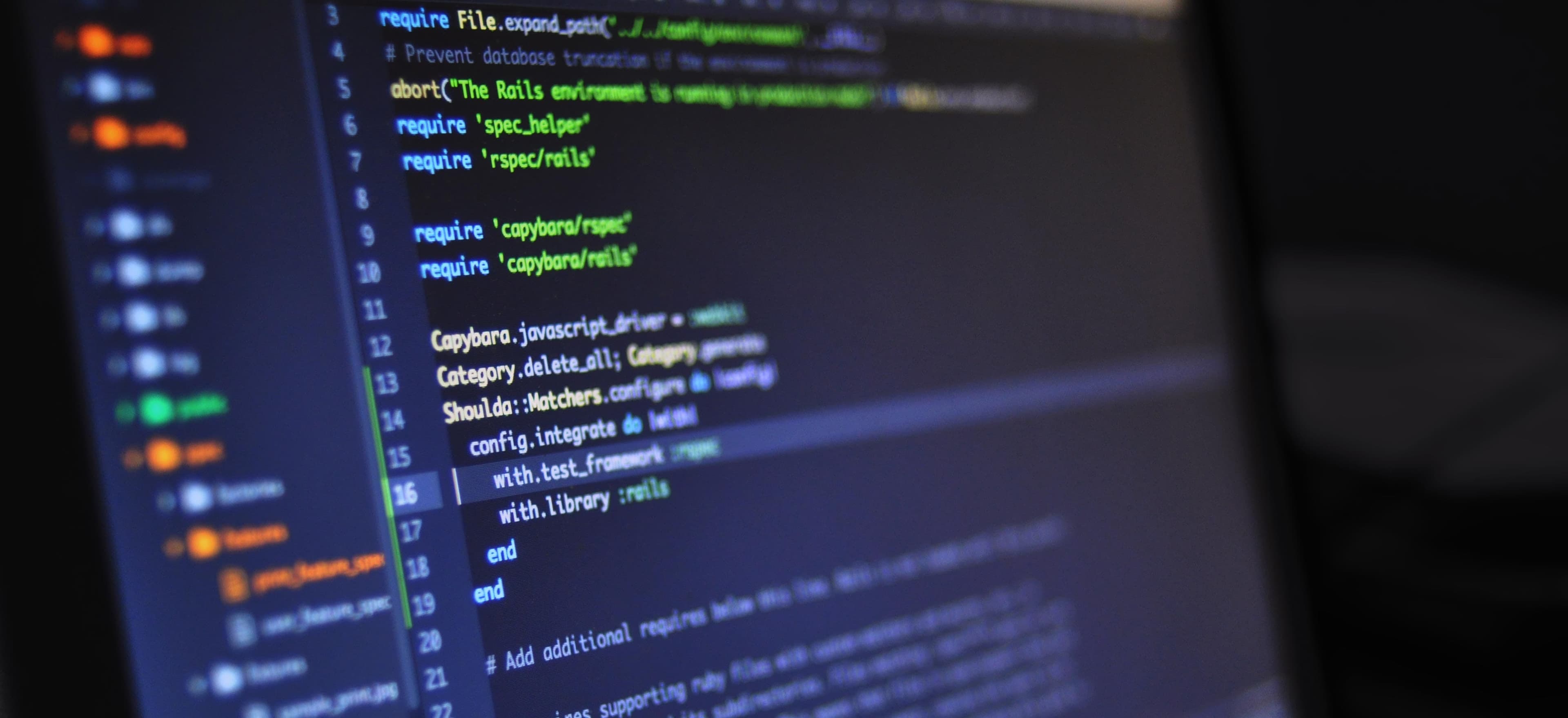
- Published on
Boosting Performance: Optimizing Microservices with API Gateways
In today's fast-paced digital world, microservices architecture has become increasingly popular due to its scalability, flexibility, and modularity. However, as the number of microservices grows within an application, managing communication between them becomes complex and can lead to performance bottlenecks. This is where API gateways come into play, serving as a central communication hub for microservices.
Understanding the Role of API Gateways
API gateways act as a single entry point for all client requests. They encapsulate the internal structure of the application, providing a unified interface to the clients while handling requests and routing them to the appropriate microservices. By doing so, API gateways streamline the communication process, improve security, and enhance the overall performance of the microservices architecture.
Performance Optimization Techniques
1. Caching
API gateways can implement caching mechanisms to store responses from microservices. This reduces the need for redundant requests to the microservices, thereby improving response time and lowering the overall load on the system. By caching frequently accessed data, API gateways can significantly enhance the performance of microservices.
// Example of caching configuration in an API gateway using Spring Boot and Hazelcast
@Configuration
@EnableCaching
public class CachingConfiguration {
@Bean
public CacheManager cacheManager() {
return new HazelcastCacheManager(hazelcastInstance());
}
@Bean
public HazelcastInstance hazelcastInstance() {
// Hazelcast configuration setup
Config config = new Config();
config.setInstanceName("microservice-cache");
// Additional configuration settings
return Hazelcast.newHazelcastInstance(config);
}
}
2. Rate Limiting
To prevent abuse of microservices and ensure fair usage of resources, API gateways can enforce rate limiting. By setting thresholds on the number of requests a client can make within a specific time frame, API gateways protect microservices from being overwhelmed by an excessive number of requests. This not only enhances performance but also improves the overall stability of the system.
// Example of rate limiting implementation using Spring Cloud Gateway
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("limited_route", r -> r.path("/limited-service/**")
.filters(f -> f.requestRateLimiter(c -> c.setRateLimiter(redisRateLimiter())))
.uri("http://limited-service"))
.build();
}
3. Load Balancing
API gateways can incorporate load balancing algorithms to distribute client requests evenly across multiple instances of a microservice. This ensures optimal resource utilization and prevents any single instance from being overwhelmed, thereby improving the overall performance and reliability of the microservices architecture.
// Example of load balancing configuration in an API gateway using Netflix Zuul
@Bean
@LoadBalanced
RestTemplate restTemplate() {
return new RestTemplate();
}
4. Request Aggregation
By aggregating multiple requests from clients into a single request to the microservices, API gateways can minimize the number of round trips and reduce network overhead. This approach optimizes performance by efficiently consolidating related requests and fetching the necessary data in a single operation.
// Example of request aggregation using GraphQL in an API gateway
@RequestMapping(value = "graphql", method = RequestMethod.POST)
public Mono<Map> executeQuery(@RequestBody String query) {
// Process and aggregate multiple requests
// Return aggregated response
}
The Bottom Line
API gateways play a vital role in optimizing the performance of microservices architecture. By implementing caching, rate limiting, load balancing, and request aggregation, API gateways effectively enhance the overall efficiency, scalability, and reliability of microservices. Employing these optimization techniques within API gateways is crucial for delivering high-performing and resilient microservices-based applications in today's competitive digital landscape.
As organizations continue to embrace microservices architecture, the role of API gateways in performance optimization becomes increasingly crucial. It is essential to leverage the capabilities of API gateways to ensure that microservices operate at peak efficiency, providing a seamless and responsive experience for users.
To delve deeper into the world of microservices and API gateways, check out this insightful article on Performance Optimization in Microservices and this comprehensive guide on API Gateway Design Patterns.