Mastering Koin: Tackling Kotlin Dependency Hell
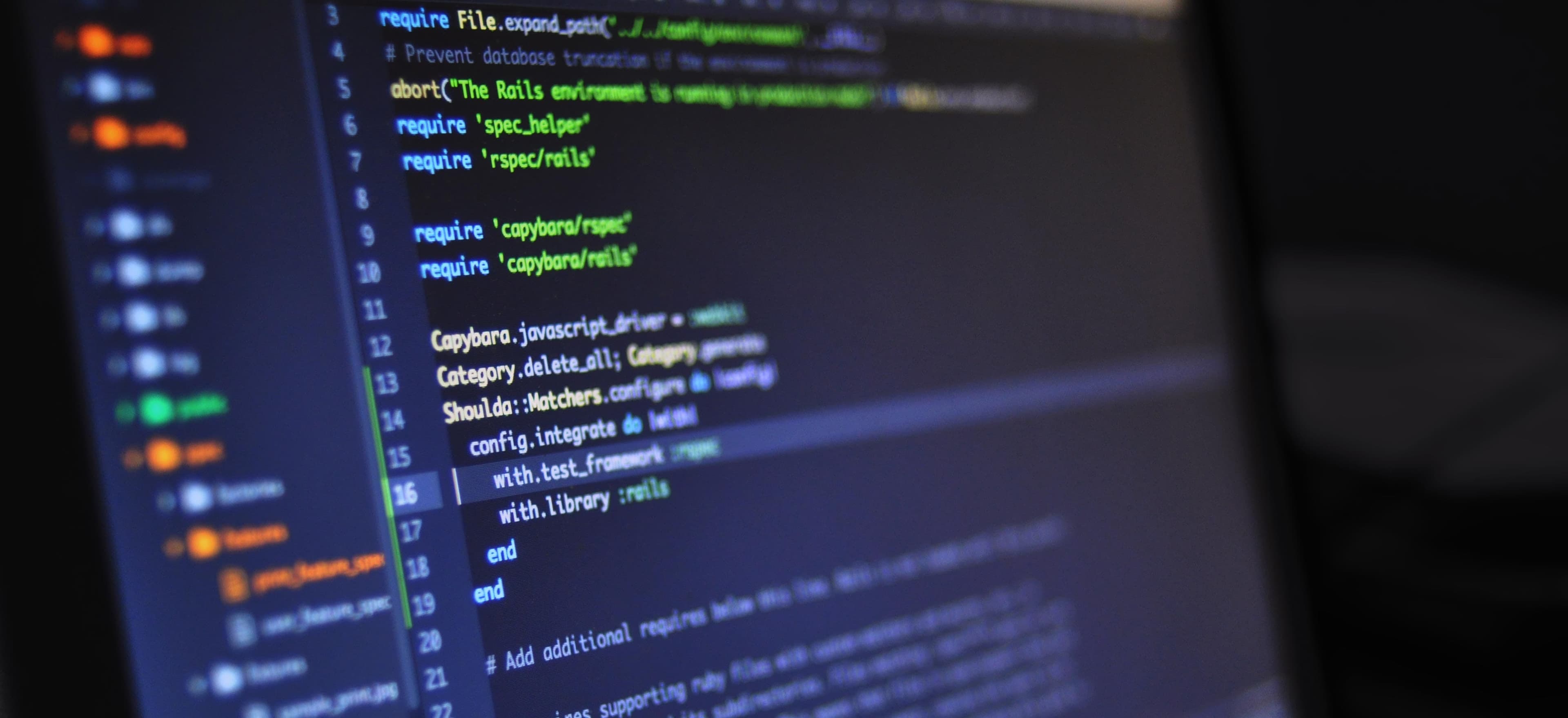
- Published on
Mastering Koin: Tackling Kotlin Dependency Hell
When it comes to Kotlin dependency injection, developers often find themselves at a crossroads. With a multitude of options available, choosing the right framework can be challenging. However, Koin, a pragmatic lightweight dependency injection framework for Kotlin, has gained significant traction due to its simplicity and ease of use.
In this post, we will delve into the world of Koin, exploring its features, advantages, and how it can help you conquer Kotlin's infamous "dependency hell." We will also demonstrate how Koin simplifies the process of managing dependencies in Kotlin applications and discuss best practices for leveraging its capabilities effectively.
Understanding the Dependency Hell
Before we delve into Koin, let's briefly address the concept of "dependency hell." In software development, "dependency hell" refers to the complexities and challenges associated with managing dependencies within an application. This includes issues such as handling object creation, managing component lifecycle, and organizing the interdependencies between various modules.
In Kotlin, managing dependencies traditionally involved using frameworks such as Dagger or manual dependency injection, both of which can introduce complexity and boilerplate code. This is where Koin comes to the rescue, offering a lightweight, pragmatic solution that simplifies dependency injection in Kotlin applications.
Introducing Koin
Koin is a pragmatic lightweight dependency injection framework for Kotlin, developed with the primary goal of making dependency injection straightforward and intuitive. Unlike traditional DI frameworks, Koin leverages the power of Kotlin's DSL (Domain Specific Language) to provide a concise and expressive syntax for managing dependencies.
Key Features of Koin
1. Kotlin-Centric DSL:
Koin embraces Kotlin's language features, allowing developers to define their dependencies using a concise and expressive DSL. This makes the code more readable and less verbose compared to traditional DI frameworks.
2. No Reflection:
Unlike some DI frameworks, Koin does not rely on reflection, resulting in improved runtime performance and reduced overhead.
3. Modular Architecture:
Koin encourages a modular approach to dependency management, making it easy to organize and encapsulate related components within different modules.
4. Simplicity and Pragmatism:
Koin follows the principle of simplicity and pragmatism, prioritizing ease of use and developer experience without compromising flexibility.
With these features in mind, let's dive into the practical aspects of using Koin for dependency injection in a Kotlin application.
Getting Started with Koin
Adding Koin to Your Project
To start using Koin in your Kotlin project, you need to add the Koin dependency to your Gradle build file. Here's how you can do it:
implementation 'org.koin:koin-core:3.1.2'
implementation 'org.koin:koin-android:3.1.2' // if you are working on an Android project
Once you have added the dependency, sync your project to ensure that the Koin library is downloaded and integrated into your project.
Defining Modules
In Koin, modules are used to declare and define dependencies. This is where you specify how your components should be created and provide the necessary configuration for dependency resolution. Let's take a look at an example of defining a Koin module for a network service:
val networkModule = module {
single { NetworkService() }
factory { ApiClient(get()) }
}
In this example, we define a networkModule
using the module
function provided by Koin. Inside the module, we use the single
and factory
functions to declare our dependencies. The single
function denotes that a single instance of NetworkService
will be created, while the factory
function indicates that a new instance of ApiClient
will be created each time it is requested.
Injecting Dependencies
Once you have defined your modules, you can inject dependencies into your classes using the by inject()
property delegate provided by Koin. Here's an example of how you can inject the NetworkService
into a ViewModel:
class MyViewModel : ViewModel() {
private val networkService by inject<NetworkService>()
// ViewModel code
}
In this example, we use the inject
property delegate to obtain an instance of NetworkService
within the MyViewModel
class. Koin takes care of resolving the dependency and providing the necessary instance.
Using Qualifiers
In some scenarios, you may have multiple implementations of the same interface and need to specify which implementation to use when injecting the dependency. Koin allows you to use qualifiers for this purpose. Here's an example of using a qualifier to specify a named dependency:
interface AnalyticsService
class FirebaseAnalyticsService : AnalyticsService
class AppCenterAnalyticsService : AnalyticsService
val analyticsModule = module {
single<AnalyticsService>(named("firebase")) { FirebaseAnalyticsService() }
single<AnalyticsService>(named("appCenter")) { AppCenterAnalyticsService() }
}
In this example, we define two implementations of the AnalyticsService
interface and use the named
qualifier to distinguish between them.
Scoping Dependencies
Managing the scope of dependencies is crucial in many applications. Koin provides support for scoping dependencies, allowing you to define different lifecycles for your components. Here's an example of scoping a dependency to a specific Android activity:
val myModule = module {
scope<MyActivity> {
scoped { MyScopedService() }
}
}
In this example, we use the scope
function to define a scope tied to the MyActivity
class. We then use the scoped
function to specify a MyScopedService
dependency scoped to the MyActivity
lifecycle.
Advantages of Using Koin
Now that we have explored the basics of using Koin for dependency injection in Kotlin, let's discuss some of the key advantages it offers:
Simplified Syntax
Koin's Kotlin-centric DSL provides a clean and concise syntax for defining and resolving dependencies, making the code more readable and maintainable.
No Boilerplate Code
With Koin, you can eliminate much of the boilerplate code typically associated with traditional DI frameworks, allowing you to focus more on creating valuable application logic.
Improved Testability
The modular and explicit nature of Koin's module definitions makes it easier to write unit tests for your components, as the dependencies are clearly defined and isolated.
Android Support
Koin provides dedicated support for Android, allowing you to seamlessly integrate dependency injection into your Android applications without added complexity.
Performance
By avoiding the use of reflection, Koin offers improved runtime performance and reduced overhead compared to some other DI frameworks.
Best Practices for Using Koin
While Koin simplifies dependency injection in Kotlin, it's essential to follow best practices to make the most of its capabilities:
Modularization
Embrace a modular architecture when defining Koin modules, organizing related components within separate modules for better isolation and encapsulation.
Scoped Dependencies
Utilize Koin's support for scoping dependencies to manage the lifecycle of components effectively, especially in Android applications where lifecycle-aware components are crucial.
Testing
Write unit tests for your modules to ensure that dependencies are resolved correctly and that the application logic behaves as expected. Koin's explicit module definitions make testing more straightforward.
Qualifiers and Named Dependencies
Use qualifiers and named dependencies judiciously to distinguish between multiple implementations of the same interface, providing clarity and flexibility in your codebase.
Error Handling and Logging
Handle dependency resolution errors gracefully and incorporate logging to track any issues that may arise during the resolution process.
Bringing It All Together
Koin offers a refreshing approach to dependency injection in Kotlin, serving as a pragmatic and lightweight alternative to traditional DI frameworks. With its Kotlin-centric design, straightforward syntax, and support for Android, Koin simplifies the process of managing dependencies and promotes a modular, testable, and maintainable codebase.
In conclusion, mastering Koin can empower Kotlin developers to conquer the challenges of dependency hell and elevate their application development experience.
Start your journey with Koin today and embrace a more streamlined approach to dependency injection in Kotlin!
Checkout our other articles