Getting Your Favicon Right in Spring MVC: A How-To Guide
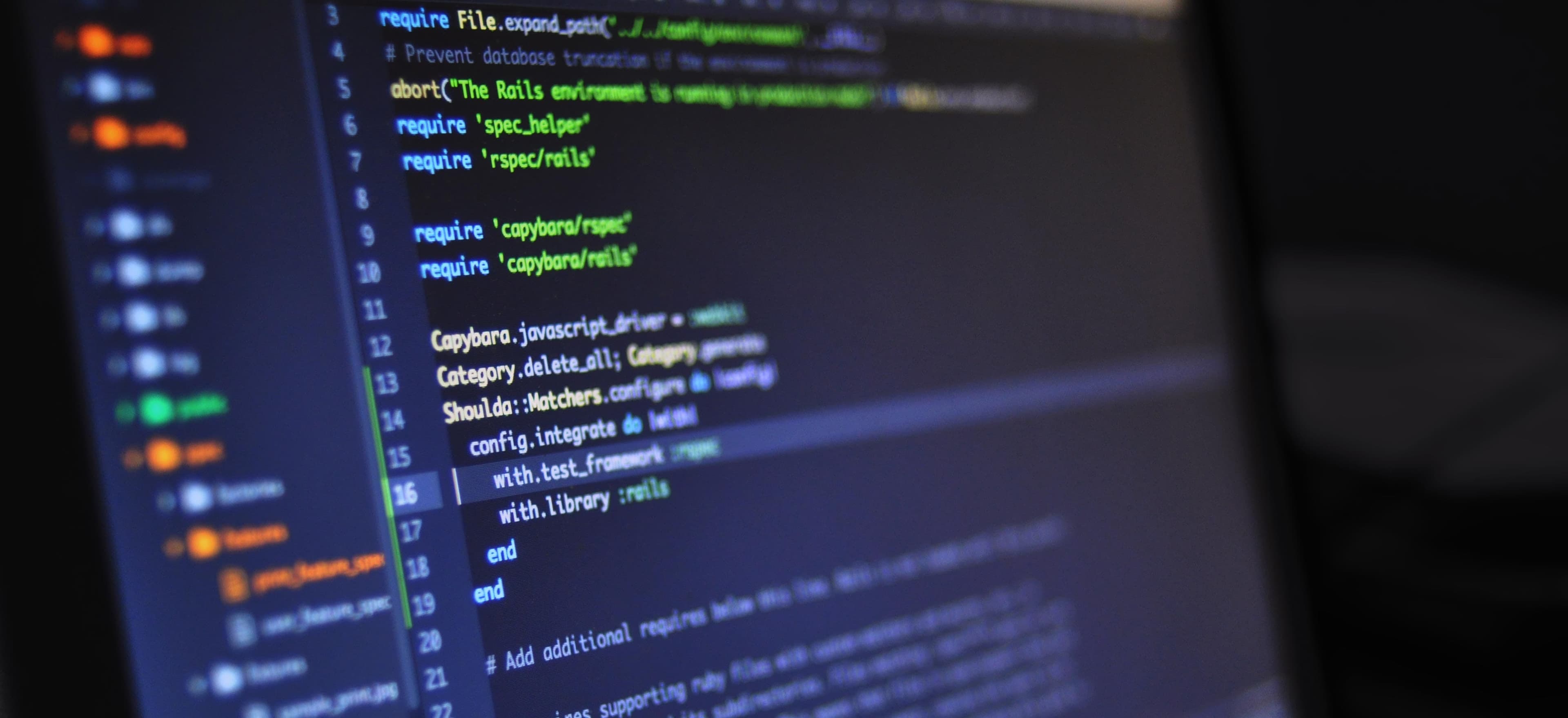
- Published on
Getting Your Favicon Right in Spring MVC: A How-To Guide
In the world of web development, even the smallest details matter. One such detail is the favicon, the tiny icon that appears in the browser tab next to the page title. While it may seem insignificant, having a well-crafted favicon can enhance the overall user experience of your website. In this guide, we will explore how to set up a favicon in a Spring MVC application, ensuring it displays correctly across different browsers and devices.
What is a Favicon?
Before diving into the technical details, let's briefly discuss what a favicon is. A favicon, short for "favorite icon," is a small image typically associated with a website. It is displayed in the browser's address bar, tabs, bookmarks, and other places to help users identify a particular website. Favicon images are usually tiny, typically 16x16 pixels, and are saved in the ICO format, though other image formats such as PNG can also be used.
Setting Up the Favicon in Spring MVC
1. Create the Favicon Image
The first step is to create a suitable favicon image. You can use a graphics editor to design an icon that represents your website or application. Once the icon is created, save it in the ICO format. If the ICO format is not available, you can use online converters to convert your image to ICO format. Alternatively, you can also use a PNG image as a favicon, especially for modern browsers which support PNG favicons.
2. Add the Favicon to the 'src/main/resources' Directory
In a Spring MVC project, we can place the favicon in the 'src/main/resources' directory. This directory is commonly used to store non-Java resources such as images, XML files, and property files. By placing the favicon here, it can be easily accessed by the application.
3. Configure Favicon in Spring Configuration
Next, we need to configure Spring MVC to serve the favicon. We can achieve this by utilizing a WebMvcConfigurer
and overriding the addResourceHandlers
method to expose the favicon to the web. Here's an example of how this can be done:
@Configuration
@EnableWebMvc
public class MvcConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/favicon.ico")
.addResourceLocations("classpath:/");
}
}
In this example, we create a WebMvcConfigurer
class and override the addResourceHandlers
method to map the URL "/favicon.ico" to the location of the favicon in the classpath.
4. Verify the Favicon
Now that the favicon is added to the Spring MVC project and configured to be served, we can verify that it is being displayed correctly. Run the application and navigate to the URL where the favicon should be displayed. Open the page in different browsers to ensure that the favicon appears consistently.
Supporting Different Browsers and Devices
1. Providing Multiple Favicon Sizes
To ensure that the favicon looks sharp on various devices with different pixel densities, it's beneficial to provide multiple sizes of the favicon. This approach, known as favicon.ico and PNG favicon (for modern browsers) can ensure that the favicon looks sharp and clear on all devices and platforms.
<link rel="icon" type="image/x-icon" href="favicon.ico">
<link rel="icon" type="image/png" sizes="32x32" href="favicon-32x32.png">
<link rel="icon" type="image/png" sizes="96x96" href="favicon-96x96.png">
In this snippet, we specify the main favicon in ICO format and provide additional PNG favicons with different sizes for better display on high-density screens.
2. Supporting Older Internet Explorer Versions
For support in older versions of Internet Explorer (IE), a specific markup using the "shortcut icon" can be added within the HTML head as follows:
<!--[if IE]><link rel="shortcut icon" href="favicon.ico"><![endif]-->
Including this within the HTML head ensures compatibility with older versions of IE.
Final Considerations
In this guide, we have discussed the importance of having a well-crafted favicon for your website and walked through the process of setting up a favicon in a Spring MVC application. By following these steps, you can ensure that your favicon displays correctly across different browsers and devices, providing a polished and professional user experience.
Remember, while the favicon may be small, its impact on the overall user experience should not be underestimated. So, whether you are launching a new website or sprucing up an existing one, take the time to get your favicon right. Happy coding!
By implementing these steps, you can ensure that your favicon displays correctly across different browsers and devices, providing a polished and professional user experience.
If you're interested in learning more about Spring MVC or web development, consider exploring Spring's official documentation for in-depth resources.