Solving Common Pitfalls in GitHub-Jenkins Integration
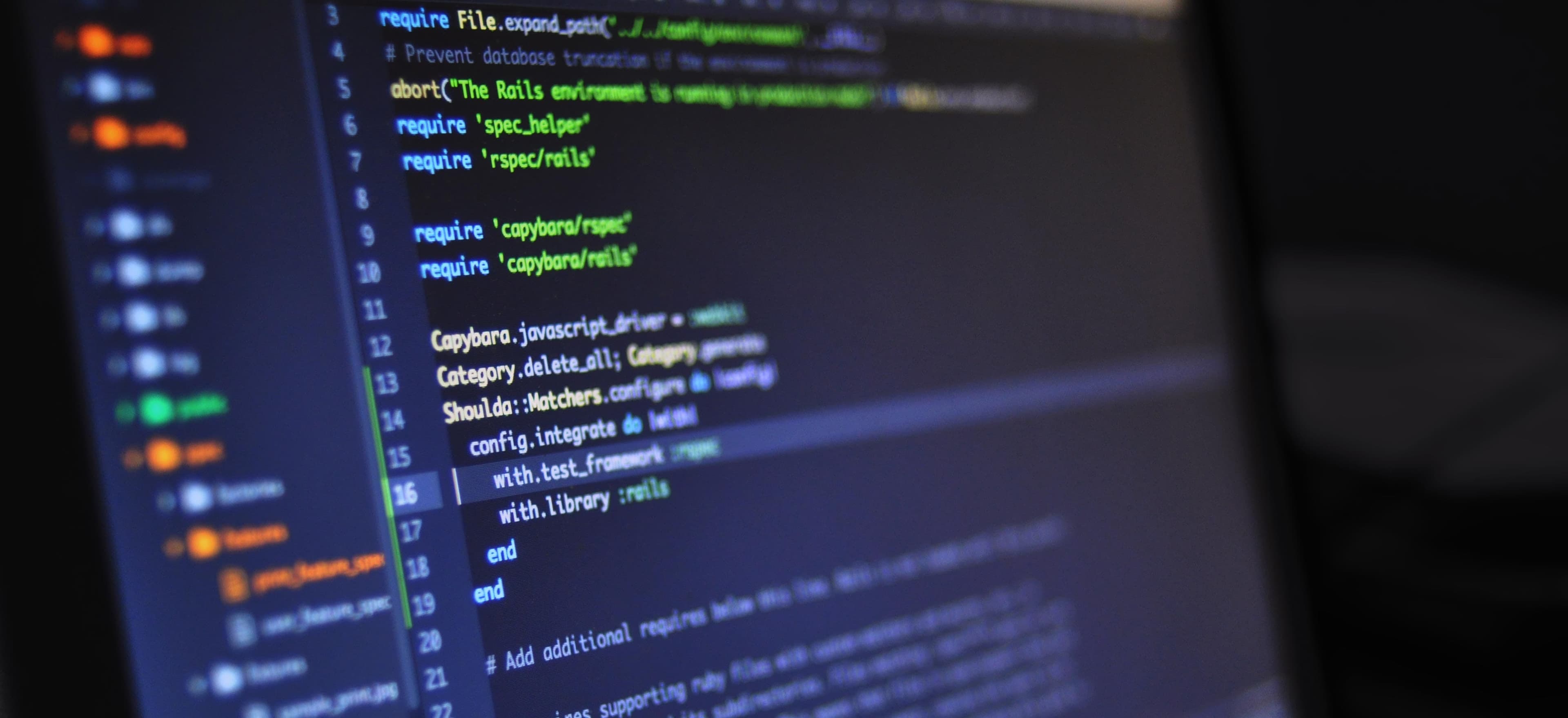
- Published on
Solving Common Pitfalls in GitHub-Jenkins Integration
GitHub and Jenkins are two essential tools in the software development lifecycle. GitHub serves as a version control system and collaboration platform, while Jenkins is an automation server used for continuous integration and continuous delivery. Integrating these two tools can streamline the development process, but it's not without its challenges.
In this blog post, we'll discuss some common pitfalls in GitHub-Jenkins integration and how to solve them effectively.
Setting Up Webhooks
One common issue when integrating GitHub with Jenkins is setting up webhooks. Webhooks are a way for GitHub to notify Jenkins when a change is pushed to the repository. Without proper webhook configuration, Jenkins won't be triggered to start the build process when a new commit is pushed.
To set up webhooks in GitHub, navigate to your repository settings, select "Webhooks," and add a new webhook. Enter your Jenkins server URL followed by "/github-webhook/" in the Payload URL field. Select the events you want to trigger the webhook, typically "Just the push event" for basic integration. Finally, make sure the webhook is active and save your settings.
In Jenkins, make sure that the GitHub plugin is installed. Create a new job, navigate to the "Build Triggers" section, and check the "GitHub hook trigger for GITScm polling" option. This configuration tells Jenkins to start the build process when it receives a webhook notification from GitHub.
By setting up webhooks correctly, you ensure that Jenkins is triggered to build whenever there's a new commit in the GitHub repository.
Managing Credentials
Another common challenge in GitHub-Jenkins integration is managing credentials. Jenkins needs access to the GitHub repository to pull the source code and perform the build. Storing sensitive information such as usernames and passwords directly in Jenkins configuration is a security risk.
One solution to this problem is to use Jenkins credentials plugin to securely store and use the GitHub credentials. Create a new "Username with password" type credential in Jenkins, providing the GitHub username and password. Then, in your Jenkins job configuration, use the credentials you created to authenticate with GitHub.
withCredentials([usernamePassword(credentialsId: 'github-creds', usernameVariable: 'GITHUB_USERNAME', passwordVariable: 'GITHUB_PASSWORD')]) {
// Your build steps here
}
By utilizing Jenkins credentials plugin, you can securely manage GitHub credentials without exposing them in your Jenkins configuration.
Branch Management
Dealing with multiple branches in GitHub and Jenkins can lead to integration challenges. You may want to build different branches in different ways or trigger specific actions based on the branch that received a push.
Jenkins provides the flexibility to handle branch management effectively through its plugins. One such plugin is the "GitHub Branch Source Plugin," which allows Jenkins to automatically discover, manage, and build branches in a GitHub repository.
After installing the "GitHub Branch Source Plugin," create a new multibranch pipeline in Jenkins and connect it to your GitHub repository. Jenkins will automatically discover all branches in the repository and create a separate pipeline for each branch. This allows you to set up specific build and behavior configurations for each branch, easing the branch management process.
properties([
pipelineTriggers([
[$class: 'GitHubPushTrigger']
])
])
By leveraging Jenkins plugins like "GitHub Branch Source Plugin," you can effectively manage branches and streamline the integration process.
Automated Pull Request Builds
When working with a collaborative GitHub workflow, it's essential to automate builds for pull requests. This ensures that changes introduced through pull requests are validated before merging into the main codebase.
Jenkins provides the ability to build pull requests automatically using the "GitHub Pull Request Builder Plugin." This plugin allows Jenkins to build pull requests as if they were normal branches, providing feedback on the proposed changes.
After installing the "GitHub Pull Request Builder Plugin," configure your Jenkins job to build pull requests from your GitHub repository. By selecting the "Build pull requests" option in your job configuration, Jenkins will automatically build pull requests and report the build status back to the pull request on GitHub.
properties([
pipelineTriggers([
[$class: 'GitHubPRTrigger']
])
])
Automating pull request builds with Jenkins ensures that proposed changes are tested and validated, contributing to a more robust codebase.
Conclusion
Integrating GitHub with Jenkins can significantly enhance the efficiency and reliability of the software development process. By addressing common pitfalls such as webhook setup, credential management, branch management, and automated pull request builds, you can ensure a smooth integration experience.
Solving these challenges not only improves the integration between GitHub and Jenkins but also contributes to the overall quality and stability of the software being developed.
Remember, successful integration is not just about connecting tools; it's about enabling seamless collaboration and automation throughout the development lifecycle.
To deepen your understanding of Jenkins, you can explore Jenkins' official documentation for comprehensive insights and updates. Additionally, you can dive into GitHub's official guide for detailed information on integrating GitHub with other tools.
Mastering the integration of GitHub and Jenkins is a valuable skill that can elevate your software development processes and contribute to the success of your projects.
Checkout our other articles