Solving Annotation Handling Challenges in Java Modules
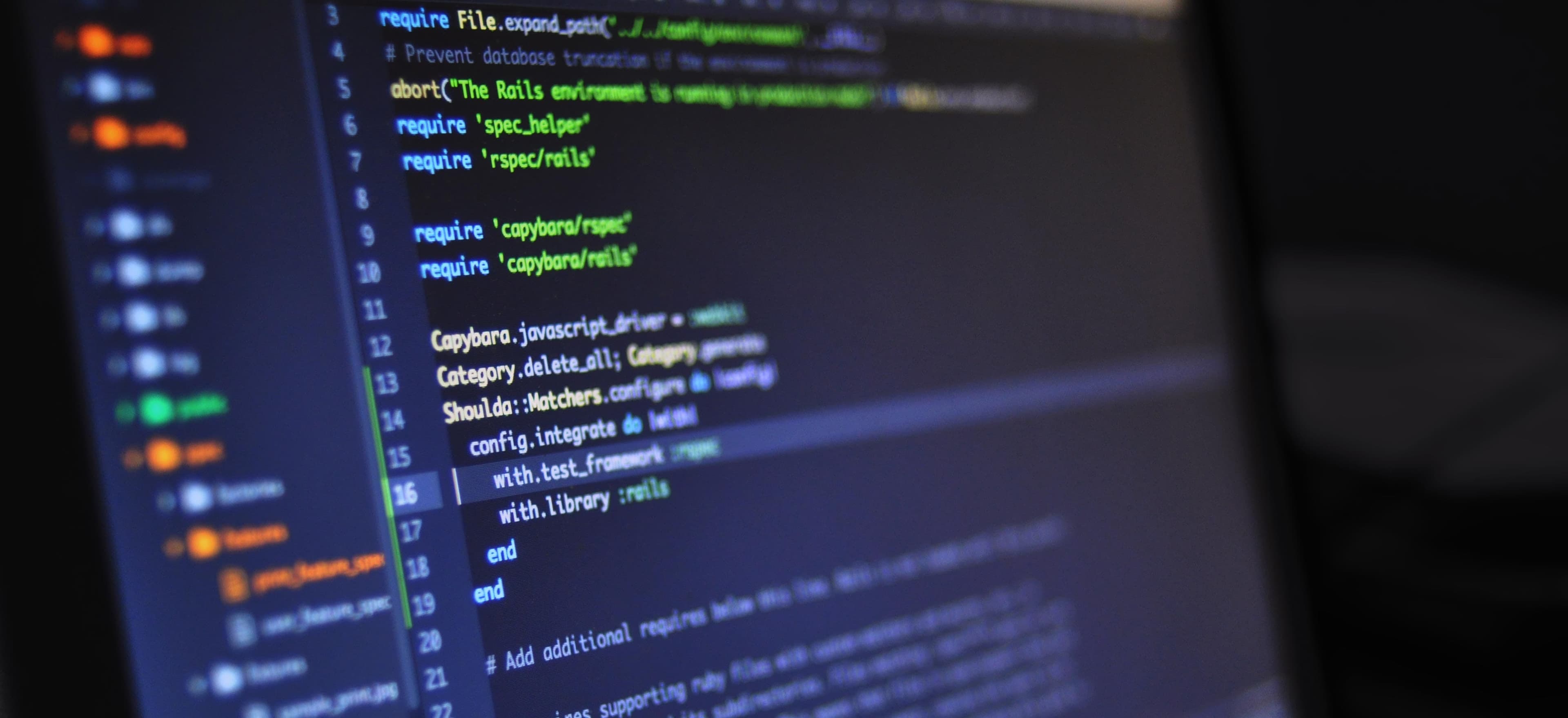
- Published on
Solving Annotation Handling Challenges in Java Modules
When working with Java modules, developers often encounter challenges when it comes to handling annotations. Annotations are a powerful feature in Java, allowing developers to add metadata and other information to the code. However, when it comes to modularizing a Java application, dealing with annotations can become tricky. In this blog post, we will explore some common challenges related to annotations in Java modules and ways to overcome them.
Understanding Annotations in Java
Before we delve into the challenges and solutions, let's quickly recap what annotations are in the context of Java. Annotations provide metadata about the program, which can be embedded within the source code or in separate files. They allow developers to add additional information, such as markers, directives, comments, and so on, to the code. Annotations are extensively used in frameworks like Spring, Hibernate, and JUnit for various purposes such as dependency injection, persistence mapping, and test case management.
Challenge 1: Accessing Annotations in Modularized Code
When modularizing a Java application, one of the primary challenges is accessing annotations from a module. In a modular application, different modules may need to access annotations defined in other modules. However, due to the encapsulation of modules, accessing annotations across module boundaries can be difficult.
To address this challenge, Java provides the opens
directive in module declarations. The opens
directive can be used to open a package to allow runtime access to the annotations it contains. Let's take a look at an example:
module com.example.mymodule {
opens com.example.mypackage to com.clientmodule;
}
In this example, the opens
directive is used to open the com.example.mypackage
package to the com.clientmodule
module, allowing the latter to access the annotations within com.example.mypackage
.
Challenge 2: Retaining Annotations at Runtime
Another common challenge when working with Java modules and annotations is retaining annotations at runtime. In Java, annotations are often processed at compile time, and their retention policy is set to SOURCE
or CLASS
by default. However, in certain scenarios, retaining annotations at runtime becomes necessary, especially when using reflection or runtime introspection.
To retain annotations at runtime, developers can use the @Retention
annotation along with the RetentionPolicy.RUNTIME
parameter. Here's an example:
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.RUNTIME)
public @interface MyRuntimeAnnotation {
// Annotation attributes
}
By specifying RetentionPolicy.RUNTIME
, the MyRuntimeAnnotation
will be retained at runtime, allowing developers to access it programmatically at runtime.
Challenge 3: Processing Annotations in Modularized Environments
In a modularized environment, processing annotations across module boundaries can be challenging, especially when using annotation processors. Annotation processors are commonly used to analyze and generate code based on annotations. However, in a modularized application, annotation processors may face difficulties in accessing annotations from other modules.
To address this challenge, developers can leverage the --module-path
option when invoking the Java compiler (javac
). By including the module path, the compiler can access annotations from other modules during the annotation processing phase. Here's an example of using the --module-path
option:
javac --module-path <path-to-modules> -processor <processor-class> <source-files>
By specifying the module path using the --module-path
option, developers can ensure that the Java compiler can access annotations from other modules during the annotation processing phase.
Challenge 4: Reflecting on Annotations in Modular Java
Reflection is a powerful mechanism in Java that enables runtime introspection of classes, interfaces, methods, and annotations. When working with modular Java applications, reflecting on annotations across module boundaries can pose a challenge, as modules are encapsulated by default.
To address this challenge, developers can use the --add-opens
option when running a modular application with the java
command. The --add-opens
option allows opening specific packages to reflective access at runtime. Here's an example of using the --add-opens
option:
java --add-opens <source-module>/<package>=<target-module>(,<target-module>)*
By specifying the --add-opens
option, developers can grant reflective access to specific packages containing annotations, enabling seamless reflection on annotations across module boundaries.
Bringing It All Together
In this blog post, we discussed some common challenges related to handling annotations in Java modules and explored various techniques to overcome these challenges. By understanding how to access, retain, process, and reflect on annotations in a modularized environment, developers can effectively leverage the power of annotations while building modular Java applications.
As Java continues to evolve, it's essential for developers to stay abreast of best practices and techniques for working with annotations in modular environments. By mastering annotation handling in Java modules, developers can build robust and maintainable modular applications that fully leverage the capabilities of annotations.
Remember, mastering annotation handling in Java modules is not just about overcoming challenges, but also about unlocking the full potential of annotations in modularized applications.
For further reading on Java modules and annotation processing, check out the official Java Platform, Standard Edition Tools Reference and the Java Tutorials by Oracle.
Happy modular coding with Java!