Switch Up Your Java: Mastering Switch Expressions
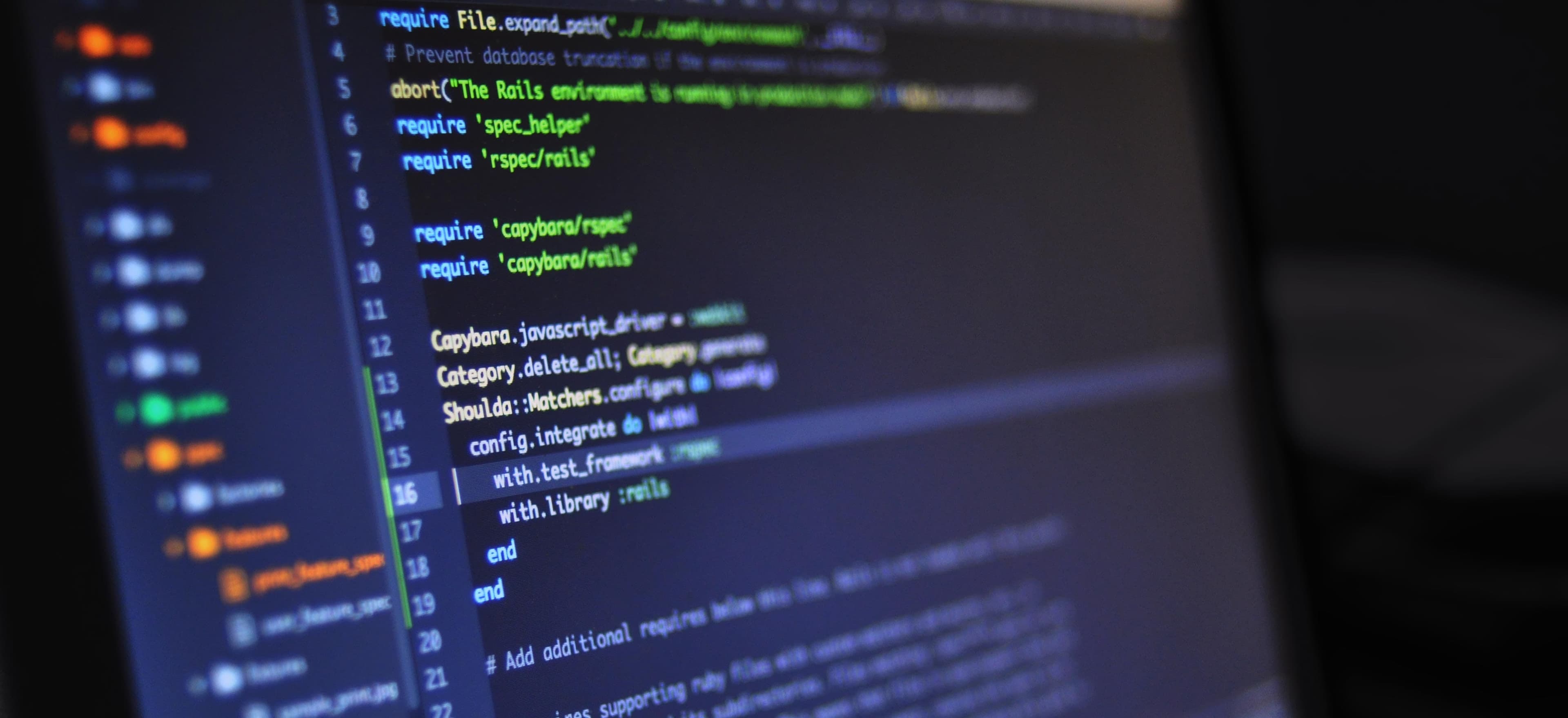
- Published on
Switch Up Your Java: Mastering Switch Expressions
When it comes to writing clean, readable, and efficient code in Java, the switch statement has been a valuable tool. However, in the past, switch statements were limited in functionality and often resulted in verbose, repetitive code. With the introduction of switch expressions in Java 12, developers now have a more powerful and concise alternative. In this post, we will dive into the world of switch expressions, explore their benefits, and understand how they can elevate your Java coding experience.
Understanding Traditional Switch Statements
Before delving into switch expressions, let's first revisit traditional switch statements in Java. The switch statement has been commonly used to perform different actions based on the value of a variable. Here's a simple example of a traditional switch statement:
int day = 3;
String dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
// additional cases
default:
dayName = "Invalid day";
break;
}
System.out.println("The day is: " + dayName);
In this example, the code assigns a day name based on the value of the day
variable. While switch statements are widely used, they have limitations. One major downside is that each case requires a break
statement to prevent fall-through, making the code longer and more error-prone.
Introducing Switch Expressions
With the introduction of switch expressions in Java 12, developers have a more streamlined way to write switch statements with improved functionality. Let's take a look at how the previous example can be transformed into a switch expression:
int day = 3;
String dayName = switch (day) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> "Wednesday";
// additional cases
default -> "Invalid day";
};
System.out.println("The day is: " + dayName);
In this updated code, the switch expression eliminates the need for break
statements. It uses the arrow symbol (->
) to map a case to a value. The result is a much more concise and readable code block.
Handling Default Cases and Non-constant Expressions
Switch expressions also provide the ability to handle default cases more effectively. In traditional switch statements, the default
case is required to be at the end of the block. With switch expressions, the default
case can be placed anywhere within the expression. This allows for more flexibility in organizing the code and handling exceptional cases.
Additionally, switch expressions support non-constant expressions in cases. This means that instead of being limited to using constant expressions like literal values or enumerated constants, developers can now use more complex expressions as case labels. This flexibility enhances the versatility of switch expressions, allowing for more dynamic and expressive code.
Leveraging the Yield Keyword
Another powerful feature that comes with switch expressions is the yield
keyword. The yield
keyword is used within switch expressions to produce a value that becomes the overall value of the switch expression. Let's modify our previous example to include the yield
keyword:
int day = 3;
String dayName = switch (day) {
case 1 -> "Monday";
case 2 -> "Tuesday";
case 3 -> {
String message = "Wednesday";
yield message;
}
// additional cases
default -> "Invalid day";
};
System.out.println("The day is: " + dayName);
In this modified code, the value of the dayName
variable is determined by the result of the yield
keyword within the case block. This allows for more complex logic and computations to be performed within a case, providing greater flexibility in handling different scenarios.
Compatibility and Best Practices
While switch expressions offer significant benefits, it's important to note that they are fully backward-compatible with existing switch statements. This means that developers can choose to gradually migrate their code to switch expressions without requiring a complete overhaul of existing codebases.
When using switch expressions, it's essential to follow best practices to ensure maintainability and readability. It's recommended to use switch expressions when the code benefits from the concise syntax and improved flow control. For simpler, single-purpose switches, traditional switch statements may still be the preferred choice.
Final Thoughts
In conclusion, switch expressions have brought a new level of clarity and efficiency to Java coding. With their concise syntax, flexibility in handling default cases and non-constant expressions, and the inclusion of the yield
keyword, switch expressions offer an enhanced alternative to traditional switch statements.
As Java continues to evolve, embracing new features like switch expressions is crucial for staying current and maximizing productivity. By mastering switch expressions, developers can write cleaner, more expressive code that is easier to maintain and understand.
So, the next time you find yourself reaching for a traditional switch statement, consider switching things up with switch expressions and elevate your Java coding experience.
Now that you've learned about switch expressions, you might want to explore Java's record feature to further streamline your code.
Checkout our other articles