Mastering Simulation: Solving Input & Output Challenges
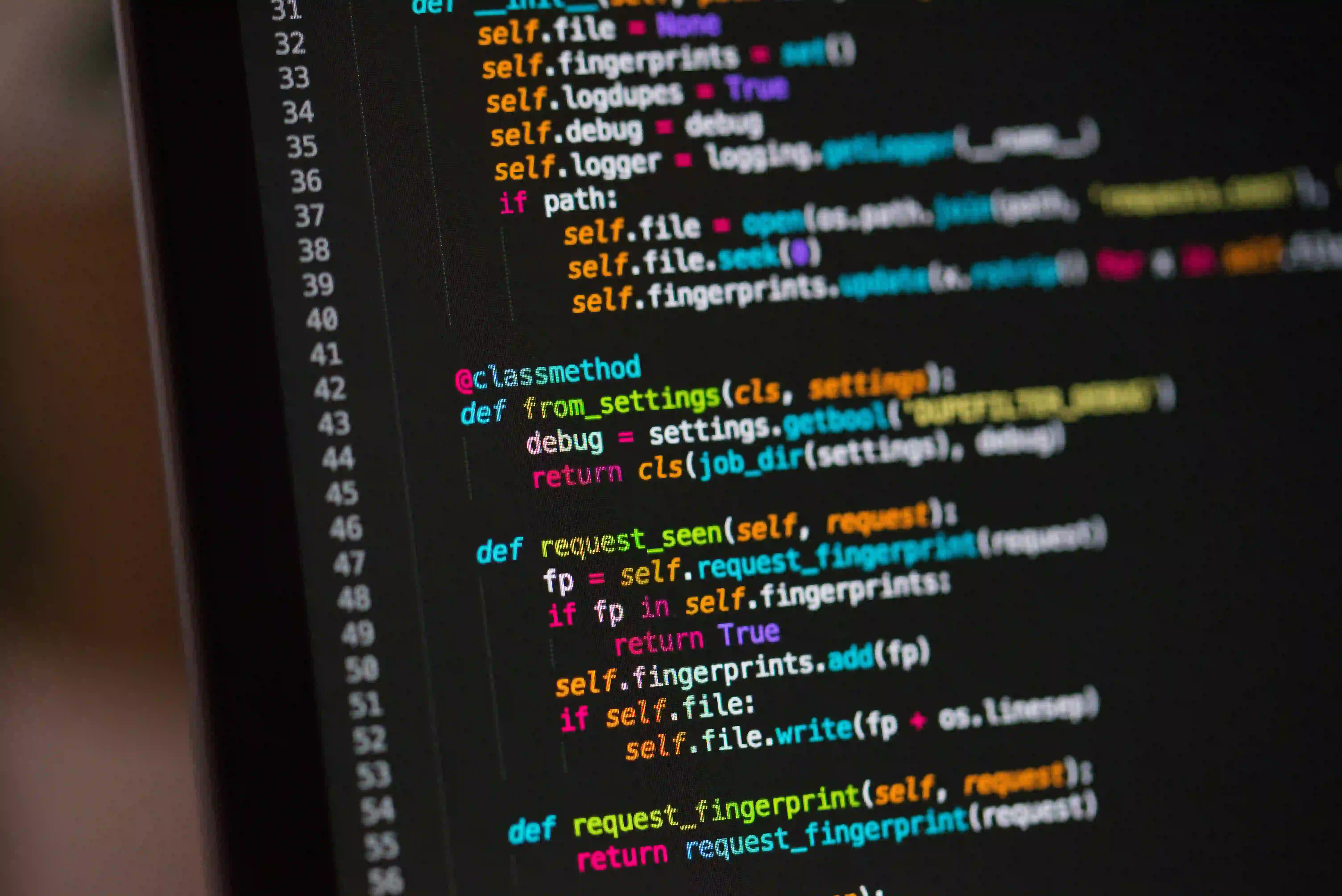
Mastering Simulation: Solving Input & Output Challenges
In the realm of software development, simulations play a vital role in testing, training, and decision-making. Java, with its robust and flexible features, is a popular choice for creating simulations. One of the key aspects of building effective simulations is handling input and output effectively. This article delves into the best practices and strategies for managing input and output in Java simulations.
Understanding the Input-Output Process
Input and output form the bridge between a simulation and the external world. In Java, the java.io
package provides classes for system input and output operations. However, when dealing with simulations, the process becomes more intricate. Simulations often involve large amounts of data and complex interactions, requiring efficient input and output management.
Efficient Input Handling
Reading Input from File
Reading input from a file is a common scenario in simulations. The java.nio.file
package offers comprehensive support for file operations. Let's consider an example where a simulation needs to read input data from a file.
import java.nio.file.*;
import java.io.IOException;
import java.util.List;
import java.util.stream.Collectors;
public class SimulationInputReader {
public List<String> readInputFromFile(String filePath) throws IOException {
return Files.lines(Paths.get(filePath))
.collect(Collectors.toList());
}
}
In this example, we utilize the Files
class to read all lines from the specified file into a List
. This approach efficiently loads the input data, making it suitable for simulations with substantial input requirements.
Parsing and Processing Input
Once the input is read, it often requires parsing and processing. This is crucial for simulations that involve complex data structures. Let's consider a hypothetical scenario where a simulation requires parsing input data containing coordinates.
public class InputProcessor {
public List<Coordinates> parseCoordinates(List<String> input) {
return input.stream()
.map(line -> {
String[] parts = line.split(",");
double x = Double.parseDouble(parts[0]);
double y = Double.parseDouble(parts[1]);
return new Coordinates(x, y);
})
.collect(Collectors.toList());
}
}
In this example, the parseCoordinates
method takes the input data and utilizes Java 8's streaming capabilities to parse it into a list of Coordinates
objects. This streamlined approach enhances the efficiency of input processing in simulations.
Effective Output Management
Writing Output to File
Similarly, writing the simulation output to a file is a crucial aspect. Let's explore an example of writing output data to a file using the Files
class.
import java.nio.file.*;
import java.io.IOException;
import java.util.List;
public class SimulationOutputWriter {
public void writeOutputToFile(List<String> outputData, String filePath) throws IOException {
Files.write(Paths.get(filePath), outputData);
}
}
In this code snippet, the write
method from the Files
class simplifies the process of writing the entire output data to the specified file. This straightforward approach is well-suited for simulations that generate copious amounts of output.
Formatting and Presenting Output
In many simulations, the output data needs to be formatted and presented clearly. Let's consider a scenario where the simulation output comprises various statistics that require proper formatting.
public class OutputFormatter {
public String formatOutputStatistics(Statistics statistics) {
return String.format("Average: %.2f, Maximum: %.2f, Minimum: %.2f",
statistics.getAverage(), statistics.getMaximum(), statistics.getMinimum());
}
}
In this example, the formatOutputStatistics
method takes a Statistics
object and formats its attributes into a clear, human-readable string. This ensures that the simulation output is presented in an intelligible manner.
My Closing Thoughts on the Matter
Effective input and output management are essential for building robust and efficient simulations in Java. By leveraging the capabilities of Java's input-output libraries and employing streamlined processing techniques, simulations can handle large volumes of data with ease. Proper input handling, parsing, output writing, and formatting are vital components for mastering simulation challenges.
Incorporating a systematic approach to input and output management not only enhances the efficiency of simulations but also ensures their scalability and reliability. As simulations continue to play a pivotal role across various domains, mastering input and output challenges remains a fundamental aspect of simulation development in Java.
In conclusion, by applying the discussed techniques and best practices, developers can elevate their simulation projects to new levels of effectiveness and robustness.
To delve deeper into Java input and output management in simulations, explore the official Java documentation and reference materials on simulation development in Java.