Overcoming Resistance: Agile Adoption in Traditional Teams
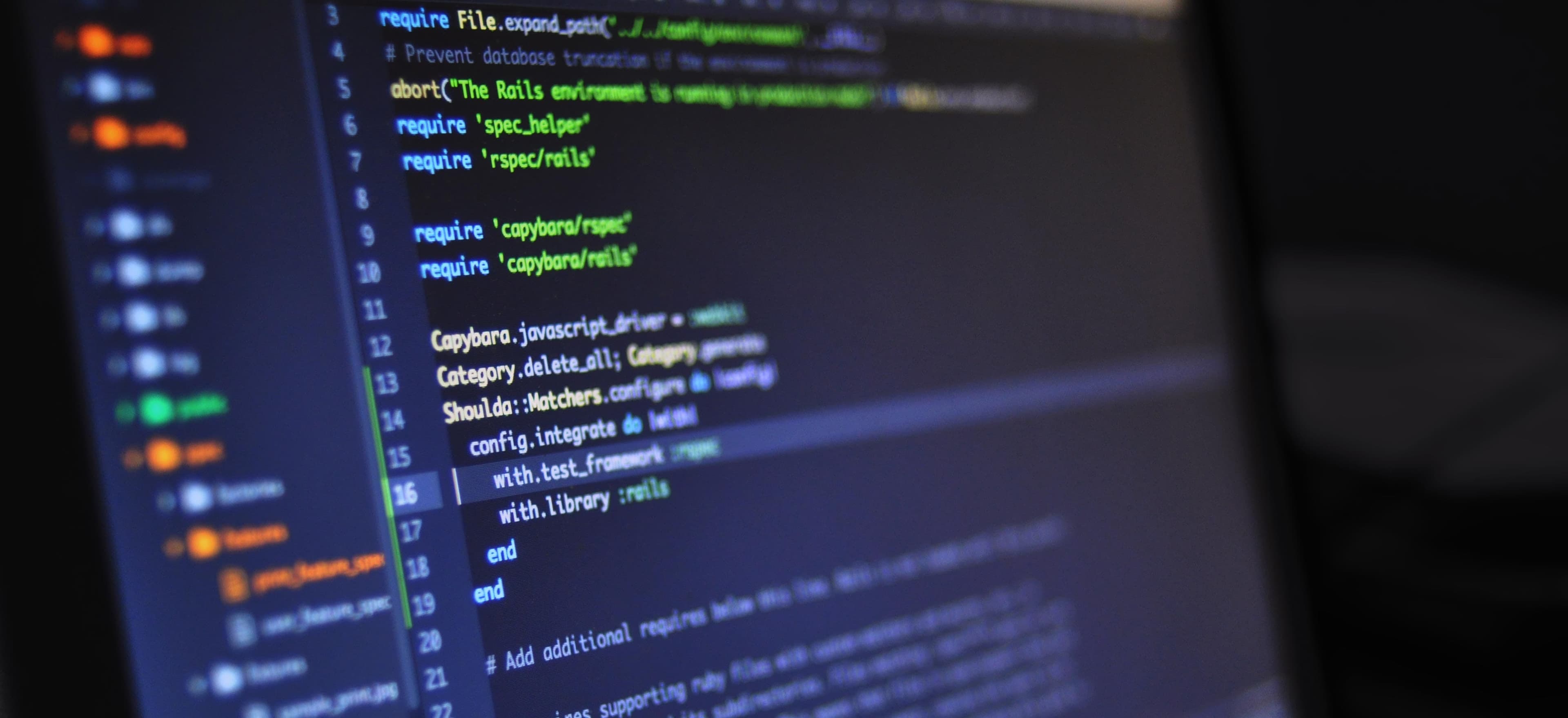
- Published on
Embracing Agile Principles and Practices in Traditional Java Teams
Agile methodologies have become increasingly popular in the software development industry due to their ability to adapt to changing requirements and deliver high-quality products efficiently. However, adopting Agile practices in traditional Java teams can be met with resistance. In this article, we will explore the challenges faced by traditional Java teams when transitioning to Agile, and we will discuss strategies to overcome these hurdles.
Understanding the Resistance
Traditional Java teams often operate within a rigid framework, following waterfall development methodologies and relying on extensive documentation and long development cycles. Transitioning to Agile requires a shift in mindset, as well as changes in processes, communication, and collaboration.
Challenge 1: Change in Mindset
Resistance: Team members may be apprehensive about moving away from the familiar waterfall approach, fearing that Agile might compromise the quality of the code or lead to a lack of documentation.
Solution: Educate the team about the benefits of Agile, such as increased flexibility, faster feedback loops, and improved customer satisfaction. Illustrate how Agile practices can enhance code quality through iterative development and frequent testing.
Challenge 2: Process Adaptation
Resistance: Traditional teams may struggle to adjust to Agile ceremonies, such as daily stand-ups, sprint planning, and retrospective meetings, which may seem disruptive to their established workflow.
Solution: Introduce these ceremonies gradually, explaining their purpose and demonstrating how they promote transparency, accountability, and continuous improvement. Encourage open communication and emphasize the value of regular feedback and adaptation.
Embracing Agile in Java Development
Now that we've identified the challenges, let's delve into the practical strategies for integrating Agile principles and practices into traditional Java development teams.
1. Embracing Test-Driven Development (TDD)
Traditional Java teams often rely on extensive manual testing, leading to longer development cycles and reduced responsiveness to change. By introducing TDD, teams can shift towards a more Agile approach to testing while improving code quality and maintainability.
@Test
public void shouldReturnHelloWorld() {
HelloWorld helloWorld = new HelloWorld();
assertEquals("Hello, World!", helloWorld.getHelloWorld());
}
Why: TDD promotes incremental development by writing tests before the actual code, ensuring that the codebase remains testable and adaptable to changing requirements.
2. Continuous Integration and Delivery (CI/CD)
In traditional Java teams, integration and deployment processes are often cumbersome and time-consuming. Embracing CI/CD practices can significantly enhance agility by automating build, test, and deployment processes, thereby reducing the risk of integration issues and enabling rapid feedback loops.
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'bash deploy.sh'
}
}
}
}
Why: CI/CD streamlines the development workflow, allowing teams to deliver incremental changes rapidly and reliably, aligning with Agile principles of frequent delivery and feedback.
3. Refactoring and Code Reviews
Traditional Java teams often overlook the importance of continuous refactoring and peer code reviews, leading to technical debt and reduced code maintainability. By emphasizing these practices, teams can leverage Agile's focus on sustainability and adaptability.
public class Calculator {
public int add(int a, int b) {
// TODO: Refactor this method for better readability and maintainability
return a + b;
}
}
Why: Refactoring and code reviews promote collaboration, knowledge sharing, and continuous improvement, aligning with Agile values of individuals and interactions over processes and tools.
Final Considerations
Transitioning traditional Java teams to Agile practices involves not only a technological shift, but also a cultural and mindset transformation. By addressing the challenges through education, gradual adaptation, and practical implementation strategies, teams can embrace Agile principles and practices while continuing to leverage their expertise in Java development.
Incorporating TDD, CI/CD, and refactoring/code reviews into traditional Java development not only aligns with Agile values but also enhances the team's ability to respond to change and deliver high-quality software efficiently. Embracing Agile in Java development is a journey that requires commitment, continuous learning, and a willingness to adapt, but the rewards in terms of improved productivity, code quality, and customer satisfaction make it a journey worth pursuing.
With the right mindset and perseverance, traditional Java teams can overcome resistance and thrive in the Agile world, bringing forth a new era of collaboration, innovation, and success.
As you navigate through this transition, keep in mind that the journey to Agile is as valuable as the destination. Embrace the challenges, learn from the experiences, and celebrate the successes, for it is through this evolution that teams truly unlock their potential and excel in delivering exceptional Java software products.
To explore more about Agile principles and practices, and their application in Java development, check out ThoughtWorks' Insights and Martin Fowler's Blog for valuable resources and perspectives.
Let's embrace the Agile journey in Java development and pave the way for a future of innovation, collaboration, and success.