Solving Eureka's Registration Delay in Spring Cloud
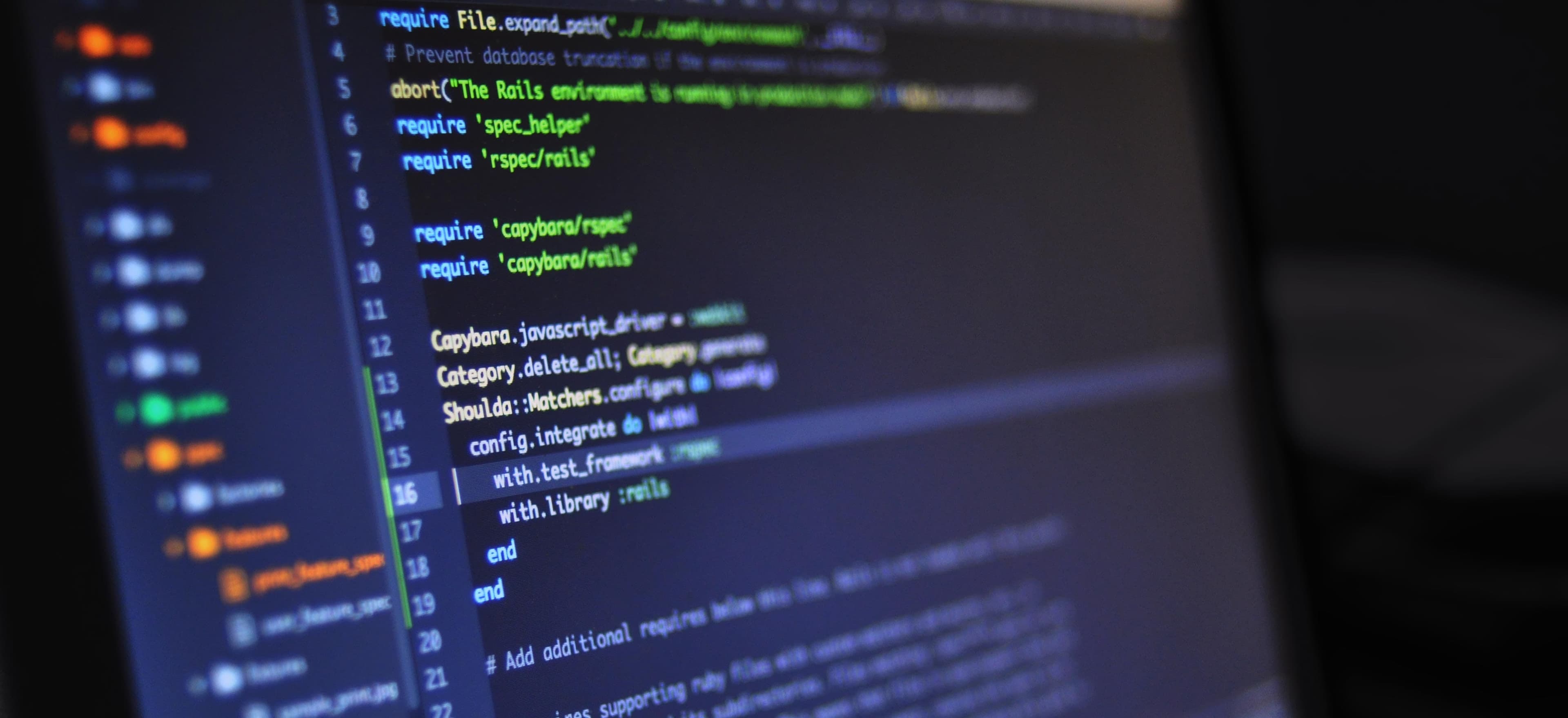
- Published on
Solving Eureka's Registration Delay in Spring Cloud
In a microservices architecture, service discovery is crucial for the services to find and communicate with each other. Eureka, a Netflix OSS component, provides a powerful way to implement service discovery. However, sometimes there might be delays in the registration of services with Eureka.
In this blog post, we will explore how to address the registration delay issue in Spring Cloud Eureka. We will discuss the potential causes of this delay and provide practical solutions to mitigate it.
Understanding the Registration Delay Issue
When a microservice starts up, it registers itself with the Eureka server so that other services can discover and communicate with it. However, in some cases, there might be a delay in the registration process. This delay can lead to instances of services not being immediately available for discovery, causing disruptions in the communication between services.
The registration delay issue can stem from various factors, including network latency, Eureka server load, and misconfigurations in the service registration process. It is essential to diagnose the root cause of the delay before implementing a solution.
Diagnosing the Root Cause
Before diving into the solution, it's crucial to diagnose the root cause of the registration delay. This can be achieved through thorough logging and monitoring of the service registration process. By examining the logs and metrics, you can gain insights into the timing of registration requests and identify any anomalies or bottlenecks in the process.
Furthermore, analyzing the Eureka server's logs and monitoring its resource utilization can provide valuable information about its performance and capacity to handle incoming registration requests. This holistic approach to diagnostics is essential for understanding the underlying reasons for the delay.
Mitigating Registration Delay
1. Adjusting Eureka Server Configuration
One of the primary steps to mitigate registration delay is to adjust the configuration of the Eureka server. Tweaking the server's settings related to registration renewal intervals, eviction timeouts, and response cache expiry can help expedite the registration process.
Example of Eureka Server Configuration in application.yml
:
eureka:
server:
enable-self-preservation: false
responseCacheUpdateIntervalMs: 30000
responseCacheAutoExpirationInSeconds: 60
renewalThresholdUpdateIntervalMs: 30000
evictionIntervalTimerInMs: 30000
By fine-tuning these parameters, you can optimize the Eureka server's behavior and minimize the registration delay.
2. Adjusting Eureka Client Configuration
In addition to tweaking the Eureka server, adjusting the configuration of the Eureka clients (microservices) can also contribute to reducing registration delay.
Example of Eureka Client Configuration in application.yml
:
eureka:
instance:
leaseRenewalIntervalInSeconds: 15
leaseExpirationDurationInSeconds: 30
client:
registryFetchIntervalSeconds: 5
By revising the lease renewal intervals, registry fetch intervals, and other relevant settings, the Eureka clients can establish a more responsive and efficient registration process with the Eureka server.
3. Implementing Retry Mechanisms
Despite optimizing the Eureka configurations, transient network issues or momentary spikes in server load can still cause registration delays. Implementing retry mechanisms in the service registration logic can mitigate the impact of such intermittent issues.
Example of implementing a retry mechanism using Spring's @Retryable
annotation:
@Service
public class EurekaRegistrationService {
@Retryable(maxAttempts = 3, value = {EurekaRegistrationException.class})
public void registerWithEureka() {
// Eureka registration logic
// ...
if (registrationFailed) {
throw new EurekaRegistrationException("Failed to register with Eureka");
}
}
@Recover
public void recoverFromRegistrationFailure(EurekaRegistrationException ex) {
// Recovery logic, e.g., logging, fallback registration, etc.
}
}
By incorporating retry capabilities, the service registration process can gracefully handle temporary impediments and eventually successfully register with the Eureka server.
Monitoring and Alerting
After implementing the mitigation strategies, it is essential to establish comprehensive monitoring and alerting mechanisms. This entails tracking the registration latency, observing trends in the registration process, and setting up alerts for any abnormal patterns or prolonged delays.
By leveraging monitoring tools such as Prometheus, Grafana, or commercial solutions like Datadog or New Relic, you can gain real-time visibility into the registration process and proactively respond to potential delay occurrences.
Closing the Chapter
Addressing the registration delay issue in Spring Cloud Eureka is vital for ensuring the seamless functioning of a microservices environment. By diagnosing the root causes, adjusting the Eureka server and client configurations, implementing retry mechanisms, and establishing robust monitoring, the impact of registration delays can be significantly minimized.
In conclusion, proactive management of the service registration process is essential for maintaining the reliability and performance of a microservices architecture utilizing Eureka for service discovery. By following the strategies outlined in this post, organizations can optimize their service registration process and enhance the overall stability of their microservices ecosystem.
Checkout our other articles