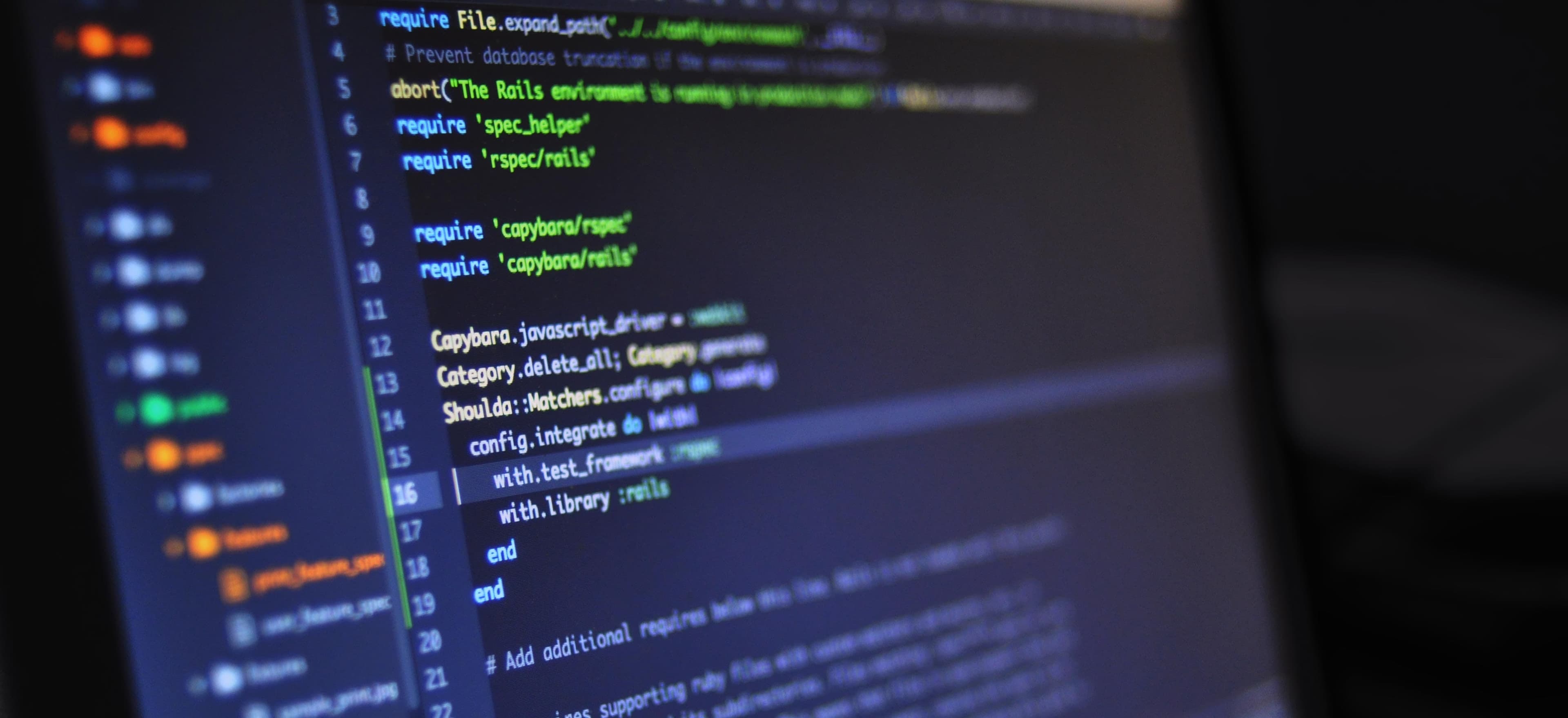
- Published on
Mastering Jetpack Compose: Overcoming Initial Hurdles
If you're into Android app development, you've probably heard about Jetpack Compose - the modern toolkit for building native Android UI. It's designed to simplify and accelerate UI development, providing a declarative way to create UIs. However, mastering Jetpack Compose can be challenging, especially for those accustomed to the traditional imperative approach. In this blog post, we'll explore some initial hurdles developers often face when getting started with Jetpack Compose and how to overcome them.
Understanding the Basics
Before diving into the code, it's essential to grasp the fundamental concepts of Jetpack Compose. Unlike the XML-based layout system in traditional Android development, Jetpack Compose relies on Kotlin to create UI components. It leverages the power of functions and lambdas to describe UI elements in a concise and expressive manner.
Setting up the Environment
To get started with Jetpack Compose, ensure that you have the latest version of Android Studio installed. Additionally, make sure to have the appropriate dependencies in your project's build.gradle
file:
android {
...
buildFeatures {
compose true
}
composeOptions {
kotlinCompilerExtensionVersion '1.0.3'
kotlinCompilerVersion '1.5.21'
}
}
dependencies {
implementation "androidx.compose.ui:ui:1.0.0-rc01"
implementation "androidx.compose.material:material:1.0.0-rc01"
implementation "androidx.activity:activity-compose:1.3.1"
}
With the environment set up, it's time to address the initial challenges faced by developers when transitioning to Jetpack Compose.
Challenge 1: Thinking in Declarative UI
One of the primary hurdles developers encounter when adopting Jetpack Compose is the shift from an imperative to a declarative UI mindset. In traditional Android development, UI components are often created imperatively, specifying the exact steps to build and update the UI. With Jetpack Compose, the focus shifts to declaring what the UI should look like based on its current state, rather than how to achieve that state.
To overcome this challenge, embrace the declarative nature of Jetpack Compose. Think of UI construction as a function of the current state and let the framework handle the underlying changes. Embrace functions like Column
, Row
, and Box
to create UI layouts. Here's an example of a simple Composable function that creates a basic UI layout:
@Composable
fun MyComposable() {
Column {
Text(text = "Hello, Jetpack Compose!")
Button(onClick = { /* Handle button click */ }) {
Text(text = "Click Me")
}
}
}
In this example, the Column
function is used to arrange the Text
and Button
components vertically. The onClick
parameter of the Button
function specifies the action to be taken when the button is clicked. This declarative approach allows for concise and readable UI code.
Challenge 2: Handling State Management
State management is another area where developers may struggle initially. In traditional Android development, managing the UI state often involves interacting with View
objects directly. With Jetpack Compose, state management is approached differently, using the remember
function to track and manage state within Composable functions.
Consider the following example, where a simple counter UI is created using Jetpack Compose:
@Composable
fun Counter() {
var count by remember { mutableStateOf(0) }
Column {
Text(text = "Count: $count")
Button(onClick = { count++ }) {
Text(text = "Increment")
}
}
}
In this example, the remember
function is used to retain the value of count
across recompositions. This ensures that the count
value is preserved even as the UI is redrawn. By leveraging the mutableStateOf
function, changes to the count
value trigger recomposition of the UI, updating the displayed count.
Challenge 3: Navigation and Routing
Navigating between different screens or components is a common requirement in app development. Jetpack Compose provides its own navigation component, which differs from the traditional FragmentManager
and Activities/ Fragments
approach.
To address this challenge, Jetpack Compose offers the NavHost
and NavController
for managing navigation within the app. Here's an example of setting up navigation using Jetpack Compose:
@Composable
fun MyApp() {
val navController = rememberNavController()
NavHost(navController = navController, startDestination = "screen1") {
composable("screen1") { Screen1(navController) }
composable("screen2") { Screen2(navController) }
}
}
@Composable
fun Screen1(navController: NavController) {
Button(onClick = { navController.navigate("screen2") }) {
Text(text = "Navigate to Screen 2")
}
}
In this example, the NavHost
and NavController
are used to manage the navigation flow. When the button in Screen1
is clicked, the navController.navigate
function is called to switch to Screen2
. Embracing the navigation components provided by Jetpack Compose simplifies the implementation of app navigation and routing.
A Final Look
Mastering Jetpack Compose may seem daunting at first, especially for developers accustomed to the traditional Android UI toolkit. However, by understanding the basics, embracing the declarative nature of UI construction, mastering state management, and leveraging the navigation components, developers can overcome the initial hurdles and harness the power of Jetpack Compose to build modern, efficient, and visually appealing Android UIs.
Remember, practice and experimentation are key to mastering any new technology, and Jetpack Compose is no exception. By persistently exploring its features and pushing through the initial learning curve, developers can unlock the full potential of Jetpack Compose in their Android app development journey.
So, dive into Jetpack Compose, tackle those initial hurdles, and unleash your creativity in building captivating Android UIs!
Feel free to explore the official Jetpack Compose documentation for in-depth insights and examples. Happy coding!
In conclusion, Jetpack Compose offers a modern, declarative approach to Android UI development, revolutionizing the way UIs are constructed and managed. While the initial hurdles may seem daunting, understanding the basics, embracing declarative UI, mastering state management, and leveraging navigation components can pave the way to proficiency in Jetpack Compose. So, dive in, experiment, and unleash your creativity in building captivating Android UIs!
Checkout our other articles