Master Java EE 8 MVC: Solving Query Parameter Issues
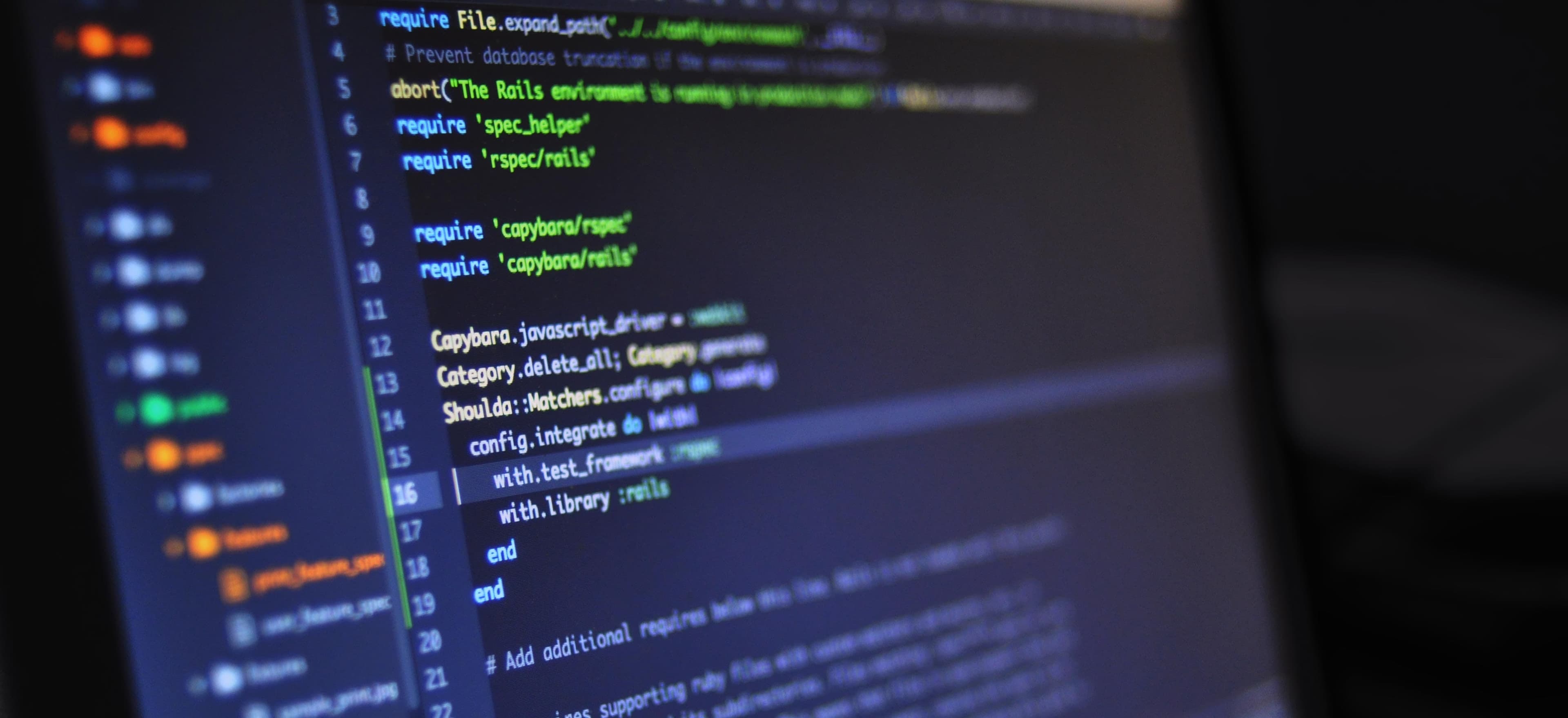
- Published on
Master Java EE 8 MVC: Solving Query Parameter Issues
Java EE 8 MVC (Model-View-Controller) is a powerful framework for building web applications in Java. One common challenge that developers face when working with Java EE 8 MVC is handling query parameters effectively. In this article, we will explore some common query parameter issues and learn how to solve them in Java EE 8 MVC.
Understanding Query Parameters
Query parameters are key-value pairs that are appended to the end of a URL. For example, in the URL https://example.com/search?q=java
, the query parameter is q=java
, where q
is the key and java
is the value. In Java EE 8 MVC, query parameters are commonly used to pass data between the client and the server.
Common Query Parameter Issues
Issue 1: Retrieving Query Parameters
One common issue that developers face is retrieving query parameters from the URL in Java EE 8 MVC. Without the proper handling, the query parameters may not be extracted correctly, leading to errors in the application.
Issue 2: Handling Missing Query Parameters
Another issue is handling cases where the expected query parameters are missing from the URL. Failing to handle such scenarios can result in unexpected behavior or exceptions in the application.
Solving Query Parameter Issues in Java EE 8 MVC
Let's dive into how we can solve these query parameter issues in Java EE 8 MVC.
Solution 1: Retrieving Query Parameters
To retrieve query parameters in Java EE 8 MVC, we can use the @QueryParam
annotation provided by the JAX-RS specification. This annotation can be applied to method parameters in a JAX-RS resource class to extract the value of a query parameter from the URL.
@Path("/search")
@GET
public String search(@QueryParam("q") String query) {
// Use the query parameter value
return "Search results for: " + query;
}
In this example, the @QueryParam("q")
annotation is used to retrieve the value of the q
query parameter from the URL. The query
parameter in the search
method will be populated with the value of the q
query parameter.
Solution 2: Handling Missing Query Parameters
To handle cases where the expected query parameters are missing, we can utilize the @DefaultValue
annotation provided by JAX-RS. This annotation allows us to specify a default value for a query parameter in case it is missing from the URL.
@Path("/search")
@GET
public String search(@QueryParam("q") @DefaultValue("java") String query) {
// Use the query parameter value (defaulted to "java" if missing)
return "Search results for: " + query;
}
In this example, the @DefaultValue("java")
annotation ensures that the query
parameter will be populated with the default value "java" if the q
query parameter is missing from the URL.
The Bottom Line
In this post, we have delved into the common query parameter issues faced in Java EE 8 MVC and provided solutions to address them effectively. By leveraging the @QueryParam
and @DefaultValue
annotations from the JAX-RS specification, developers can retrieve query parameters and handle missing parameters with ease, ensuring the robustness and reliability of their Java EE 8 MVC applications.
By mastering the techniques discussed in this article, developers can enhance their proficiency in Java EE 8 MVC and build web applications that excel in handling query parameters seamlessly.
To dive deeper into Java EE 8 MVC and explore its capabilities, check out Java EE 8 Documentation and Oracle's Java EE 8 Tutorial. Happy coding!
Checkout our other articles