Tackling Slow Queries in Apache Cassandra: A Guide
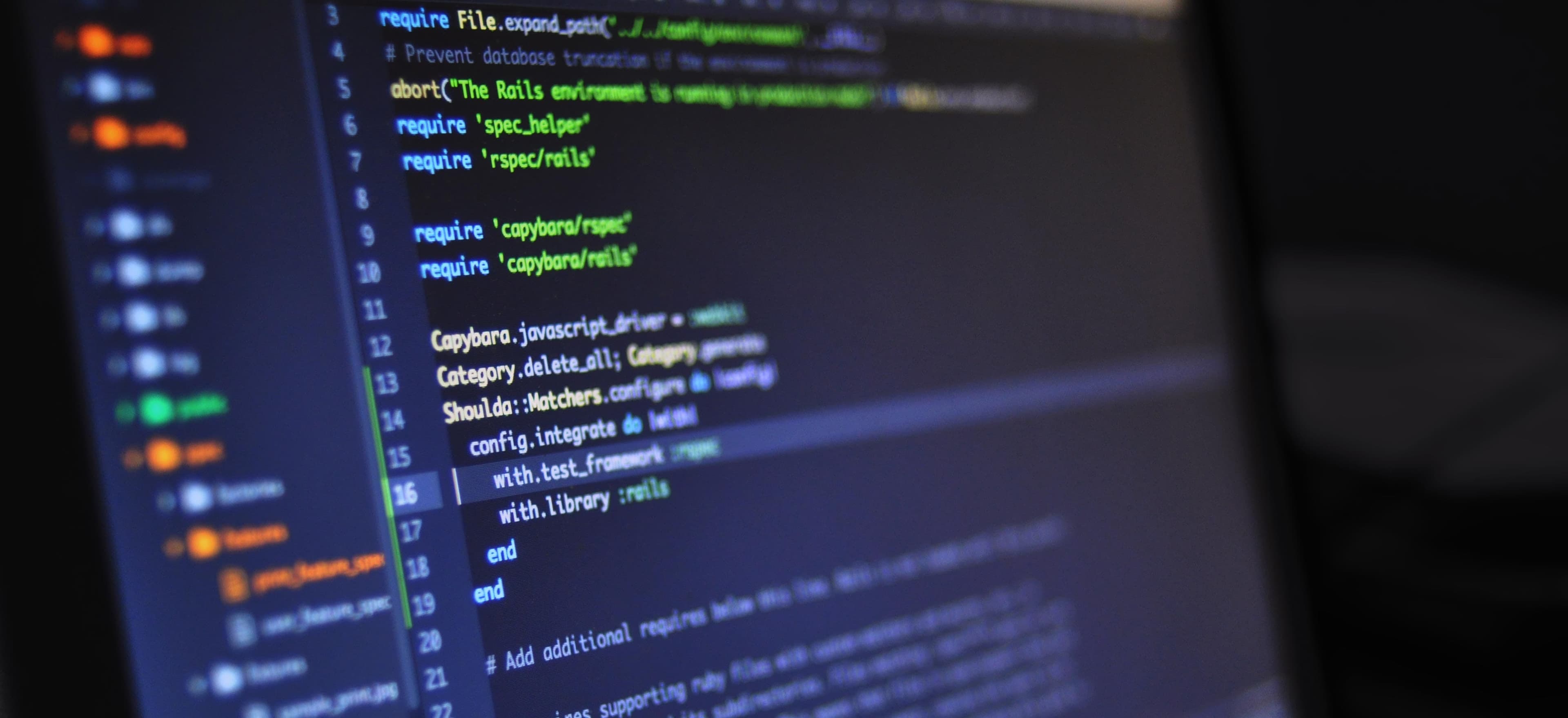
- Published on
Tackling Slow Queries in Apache Cassandra: A Guide
Apache Cassandra is a high-performing NoSQL database, known for its fault tolerance and linear scalability. However, as with any database system, it's essential to optimize query performance. In this guide, we'll explore common causes of slow queries in Cassandra and provide practical solutions to address them.
Understanding Slow Queries in Cassandra
1. Inefficient Data Modeling
One of the primary reasons for slow queries in Cassandra is inefficient data modeling. When designing a data model, it's crucial to align it with the query patterns.
CREATE TABLE IF NOT EXISTS users (
user_id UUID PRIMARY KEY,
username TEXT,
email TEXT
);
In this example table, the users
table is modeled to directly query by user_id
. This efficient data modeling helps optimize query performance.
2. Inadequate Use of Secondary Indexes
Using secondary indexes in Cassandra can lead to slow queries if not used judiciously. It's important to keep in mind that Cassandra is optimized for querying by primary key, and the use of secondary indexes should be limited to specific use cases.
CREATE INDEX IF NOT EXISTS ON users (email);
In this example, use of a secondary index on the email
column can lead to slower queries, especially with large datasets and high write throughput.
3. Overreliance on ALLOW FILTERING
The ALLOW FILTERING
directive in Cassandra allows querying on non-indexed columns. While it can be useful in certain scenarios, relying on it excessively can result in slow queries, as it entails scanning the entire table.
SELECT * FROM users WHERE username = 'john' ALLOW FILTERING;
In this example, the ALLOW FILTERING
directive can cause a full table scan, leading to performance degradation, especially on large datasets.
Techniques to Optimize Slow Queries
1. Proper Data Modeling
To address inefficiencies in data modeling, it's crucial to understand the query patterns and design the data model according to those patterns. Denormalization, using composite keys, and optimizing for specific queries can significantly improve query performance.
2. Limited Use of Secondary Indexes
Instead of relying on secondary indexes, consider denormalizing the data or using materialized views to support specific query patterns. This approach can reduce the reliance on secondary indexes and improve overall query performance.
CREATE MATERIALIZED VIEW IF NOT EXISTS users_by_email AS
SELECT * FROM users WHERE email IS NOT NULL AND user_id IS NOT NULL
PRIMARY KEY (email, user_id);
In this example, a materialized view is used to support querying users by email, improving the query performance compared to using a secondary index.
3. Avoiding ALLOW FILTERING
To avoid the pitfalls of ALLOW FILTERING
, it's essential to design the data model to support the required queries. This may involve denormalizing the data or creating additional tables tailored to specific query patterns.
CREATE TABLE IF NOT EXISTS users_by_username (
username TEXT,
user_id UUID,
email TEXT,
PRIMARY KEY (username, user_id)
);
In this example, a separate table is created to support querying users by username, eliminating the need for ALLOW FILTERING
.
Monitoring and Profiling Queries
1. Using Tracing
Cassandra provides a powerful tracing feature that allows you to track the execution of queries. By enabling tracing for specific queries, you can gain insights into query performance and identify any bottlenecks.
Session session = cluster.connect();
ResultSet rs = session.execute("SELECT * FROM users WHERE username = 'john'").enableTracing();
Enabling tracing for a query provides detailed information about its execution, including the time taken for each operation and any coordination between nodes.
2. Monitoring with Prometheus and Grafana
Integrating Cassandra with monitoring tools like Prometheus and Grafana can provide real-time visibility into query performance, resource utilization, and cluster health. This proactive approach allows you to identify slow queries and performance issues before they impact the application.
The Last Word
Optimizing query performance in Apache Cassandra is crucial for ensuring the scalability and responsiveness of your application. By understanding the common causes of slow queries and employing effective optimization techniques, you can enhance the overall efficiency and reliability of your Cassandra database.
Remember, efficient data modeling, limited use of secondary indexes, and careful query design are key to achieving optimal performance in Apache Cassandra.
Incorporating monitoring and profiling tools further empowers you to proactively identify and address any potential performance bottlenecks, ensuring smooth operation of your Cassandra cluster.
By following the guidelines outlined in this guide, you can effectively tackle slow queries in Apache Cassandra, resulting in a robust and high-performing database system for your applications.
Checkout our other articles