Simplifying REST in Vert.x: Master Your HTTP Headers!
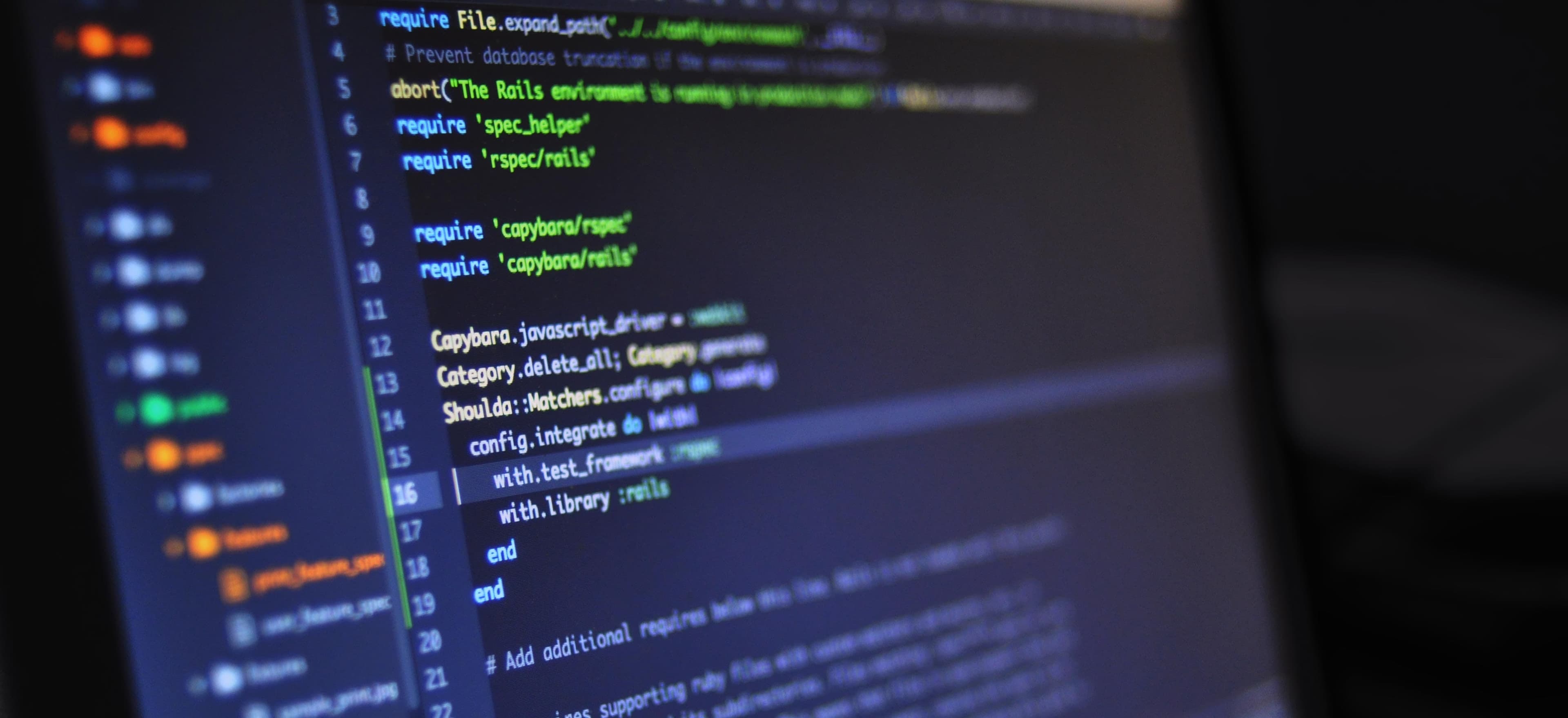
- Published on
Simplifying REST in Vert.x: Master Your HTTP Headers!
In the world of web development, creating robust and efficient RESTful APIs is a crucial aspect of building modern applications. However, the process of handling HTTP headers can often be cumbersome and prone to error. This is where Vert.x, a toolkit for building reactive applications on the JVM, comes into play. With its powerful features and intuitive design, Vert.x simplifies the handling of HTTP headers, allowing developers to focus on building great APIs without getting bogged down in the details.
Understanding the Importance of HTTP Headers
Before we delve into how Vert.x simplifies HTTP header handling, it's essential to understand the significance of HTTP headers in the context of RESTful APIs. HTTP headers carry vital information about a client request or server response, ranging from content type and encoding to authentication tokens and caching directives.
One of the key challenges in RESTful API development is effectively managing and manipulating HTTP headers to ensure proper communication between clients and servers. This includes handling common tasks such as content negotiation, security, and caching, all of which are heavily reliant on HTTP headers.
How Vert.x Streamlines HTTP Header Handling
Vert.x provides a straightforward and efficient way to work with HTTP headers through its built-in HttpServerRequest
and HttpServerResponse
interfaces. These interfaces encapsulate the HTTP request and response objects, offering a rich set of methods for interacting with headers.
Let's take a look at how Vert.x simplifies common HTTP header operations:
Accessing Incoming HTTP Headers
router.route().handler(routingContext -> {
HttpServerRequest request = routingContext.request();
MultiMap headers = request.headers();
String contentType = headers.get("Content-Type");
// Perform operations based on the content type
});
In the above code snippet, we access the incoming HTTP headers from a client request. By using the request.headers()
method, we retrieve all the headers as a MultiMap
data structure, which allows for easy retrieval and manipulation of individual header values. This simplifies the task of extracting specific headers, such as Content-Type
, and making decisions based on their values.
Setting Outgoing HTTP Headers
router.route().handler(routingContext -> {
HttpServerResponse response = routingContext.response();
response.putHeader("Content-Type", "application/json");
// Set other headers as needed
response.end("Response Body");
});
In the above code, we set the outgoing HTTP headers for a server response. By using the response.putHeader()
method, we can easily add or overwrite specific headers, such as setting the Content-Type
for the response. This straightforward approach simplifies the process of ensuring that the appropriate headers are included in the server response.
Leveraging Vert.x for Advanced HTTP Header Operations
Beyond basic header access and manipulation, Vert.x offers advanced features for handling HTTP headers in RESTful APIs. These include:
Content Negotiation
Vert.x provides support for content negotiation, allowing APIs to respond with different representations of a resource based on the client's preferred content types. By examining the Accept
header from the client request, developers can determine the most suitable response representation and tailor the output accordingly.
Caching Directives
Managing caching directives is crucial for optimizing the performance of RESTful APIs. Vert.x simplifies this process by exposing methods for setting caching-related headers, such as Cache-Control
and Expires
, to control the caching behavior of client and proxy servers.
Best Practices for Effective HTTP Header Handling
While Vert.x streamlines HTTP header operations, adhering to best practices is essential for ensuring robust and maintainable RESTful APIs. Here are some tips to consider:
Use Constants for Header Names
To avoid hard-coding header names throughout the codebase, define constants for commonly used headers. This promotes consistency and reduces the likelihood of typos or mismatches when working with headers.
Validate and Sanitize Header Inputs
Always validate and sanitize incoming header values to prevent security vulnerabilities such as injection attacks. Input validation ensures that the headers conform to expected formats and do not pose potential risks.
Leverage Vert.x's Async Capabilities
Given Vert.x's asynchronous nature, make full use of its non-blocking I/O to handle HTTP headers efficiently. Asynchronous processing allows for concurrent handling of header operations without blocking the event loop.
Lessons Learned
With Vert.x's seamless handling of HTTP headers, building and maintaining RESTful APIs becomes more manageable and less error-prone. By leveraging its intuitive interfaces and advanced features, developers can focus on crafting robust and performant APIs without being encumbered by the complexities of HTTP header management.
In the dynamic landscape of web development, mastering HTTP header handling is a critical skill that sets the foundation for secure and efficient communication between clients and servers. With Vert.x as a powerful ally, developers can elevate their RESTful API development to new heights, delivering exceptional experiences to users while maintaining code clarity and conciseness.
To explore more about HTTP header handling and Vert.x, refer to the official Vert.x documentation.
Start harnessing the power of Vert.x's HTTP header handling capabilities and elevate your RESTful API development today!
Checkout our other articles