Navigating Oracle's JDK 8: Life without Collection Literals
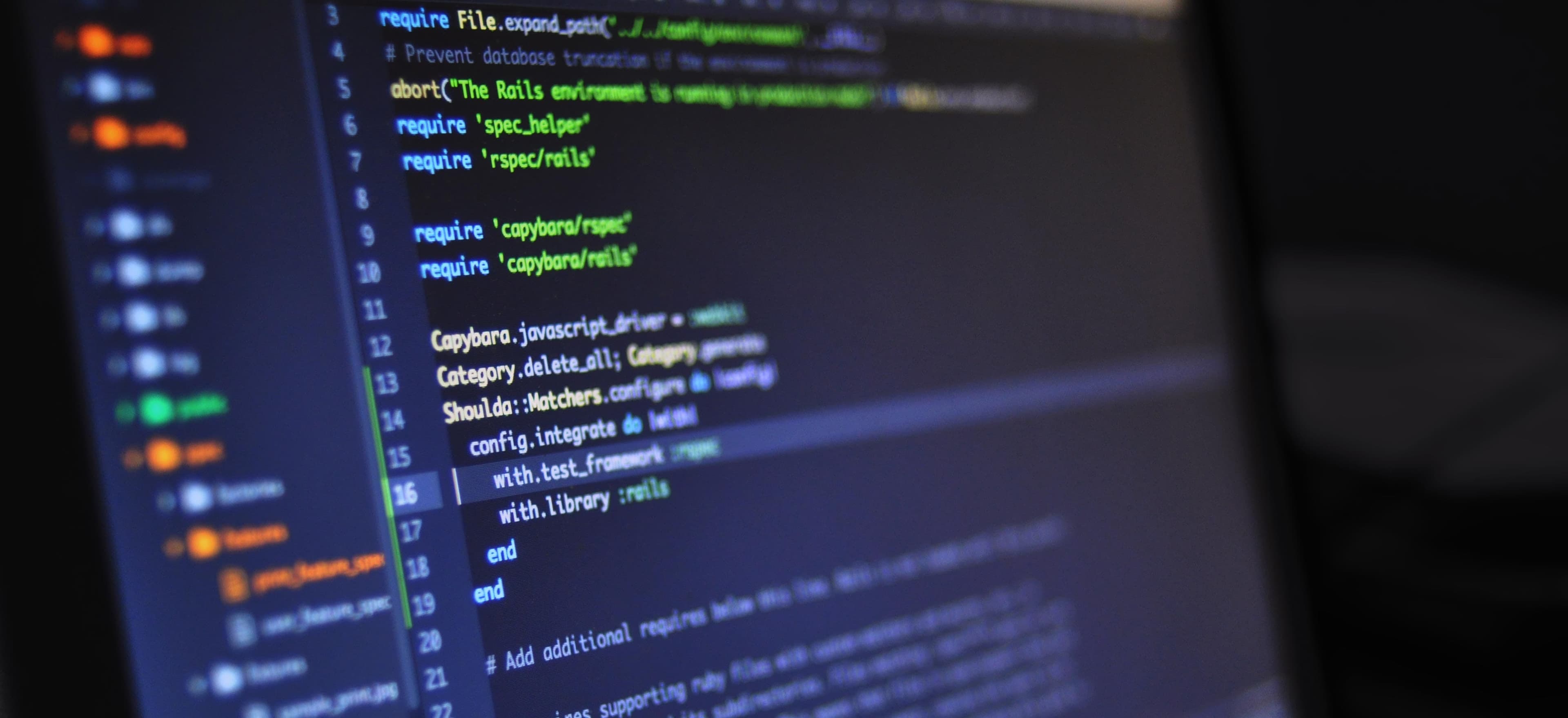
- Published on
Navigating Oracle's JDK 8: Life without Collection Literals
In Java, Collection Literals are a concise way to represent a list of elements. However, Oracle's JDK 8 doesn't provide native support for Collection Literals, causing developers to come up with workarounds. In this article, we will explore the absence of Collection Literals in Oracle's JDK 8 and how developers can navigate this limitation.
Understanding Collection Literals
Collection Literals are a convenient way to initialize collections (such as lists, sets, and maps) in a concise and readable manner. For example, in languages like Python or JavaScript, you can simply define a list as [1, 2, 3]
or a map as { "key1": "value1", "key2": "value2" }
. However, Java, especially in Oracle's JDK 8, lacks this native support, making the initialization of collections more verbose and less readable.
The Absence of Collection Literals in Oracle's JDK 8
Unlike some modern programming languages, Oracle's JDK 8 does not provide built-in syntax for Collection Literals. This means that when working with collections, developers often have to resort to traditional instantiation and population methods, leading to verbose and less readable code.
Workarounds and Solutions
Using Arrays and Converting Them to Collections
In the absence of Collection Literals, one common approach is to use arrays and then convert them to collections using utility methods provided by the java.util.Arrays
class. This approach requires more code and is less intuitive than using Collection Literals, but it gets the job done.
// Using arrays and converting them to collections
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
Using Double Brace Initialization
Another workaround involves using double brace initialization, which is a somewhat controversial technique due to its potential impact on performance and memory usage. It involves creating an anonymous inner class with an instance initializer block.
// Double brace initialization
List<String> names = new ArrayList<String>() {{
add("Alice");
add("Bob");
add("Charlie");
}};
Third-Party Libraries
Alternatively, developers can opt to use third-party libraries such as Guava or Apache Commons, which provide more expressive and concise ways of initializing collections.
// Using Guava library for collection initialization
List<String> names = Lists.newArrayList("Alice", "Bob", "Charlie");
Pros and Cons of Workarounds
While the aforementioned workarounds allow developers to achieve collection initialization in the absence of Collection Literals, each approach has its own set of pros and cons.
Pros
- Compatibility: These workarounds are compatible with Oracle's JDK 8 and do not require migrating to a newer Java version.
- Flexibility: Developers have the flexibility to choose the approach that best fits their coding style and project requirements.
Cons
- Verbosity: The workarounds often lead to more verbose code, reducing readability and maintainability.
- Performance Impact: Some workarounds, like double brace initialization, may have potential performance and memory usage implications.
Looking Toward the Future
As the Java language evolves, newer versions such as JDK 9 and beyond may introduce language features to address the absence of Collection Literals in Oracle's JDK 8. Until then, developers can make use of the discussed workarounds or consider migrating to a newer Java version for native support of Collection Literals.
Final Considerations
The absence of Collection Literals in Oracle's JDK 8 presents a challenge for developers who seek concise and readable ways to initialize collections. While workarounds such as using arrays, double brace initialization, and third-party libraries provide alternatives, they come with their own set of trade-offs. As the Java ecosystem progresses, it's essential for developers to stay updated on language enhancements and explore the best practices for working with collections in Oracle's JDK 8 and beyond.
Remember, while Collection Literals may not be natively supported in Oracle's JDK 8, there are always creative solutions to simplify the process of working with collections.
For more insights on Java development and best practices, feel free to delve into Oracle's official documentation on Java Collections and keep up with the latest updates from the OpenJDK community.
Checkout our other articles