Mastering Java 8 Lambda for Efficient Parallelism
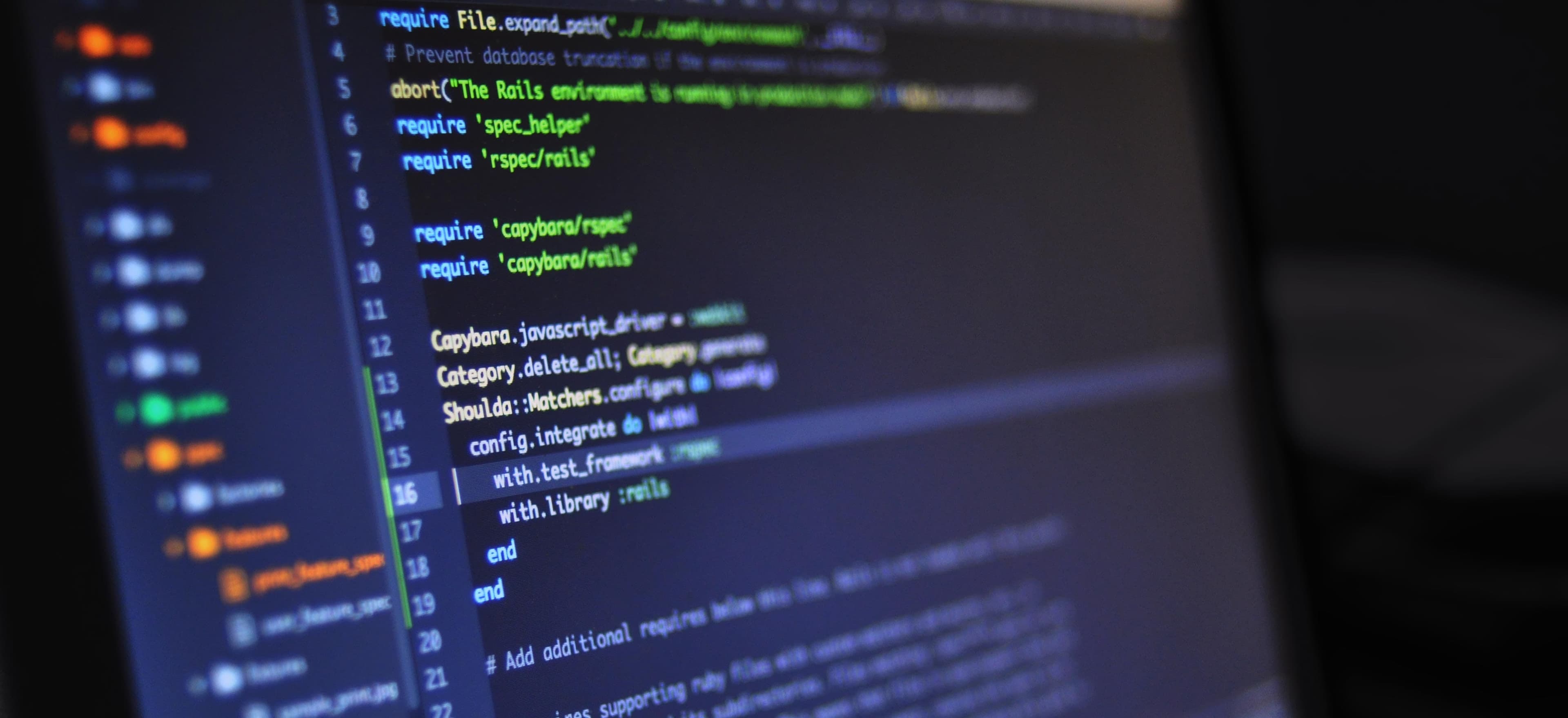
- Published on
Mastering Java 8 Lambda for Efficient Parallelism
Java 8 Lambda expressions have revolutionized the way developers write code by introducing functional programming to the language. With Lambda expressions, Java has taken a huge leap forward, allowing for more concise and readable code. Moreover, the introduction of Stream API has enabled efficient parallelism, making it easier to leverage the multi-core processors of modern machines. In this post, we will delve into the world of Java 8 Lambdas and explore how to harness their power for efficient parallelism.
Understanding the Basics of Lambda Expressions
Lambda expressions are anonymous functions that allow you to treat behavior as a method argument or code as data. In Java, a Lambda expression consists of parameters, an arrow (->), and a body. This concise syntax allows for the implementation of functional interfaces more easily than ever before.
// Simple example of a Lambda expression
() -> System.out.println("Hello, Lambda!");
Here, the () -> System.out.println("Hello, Lambda!")
represents a Lambda expression with no parameters and a single statement in its body.
Leveraging Lambda Expressions for Parallelism
One of the most powerful features of Java 8 Lambda expressions is their ability to enable parallelism. The Stream API, introduced in Java 8, allows for declarative-style parallelism, making it simpler to perform operations concurrently.
Let's consider a scenario where we have a collection of elements and we want to perform a time-consuming operation on each element. Using Lambda expressions and the Stream API, we can easily parallelize this operation to take advantage of multiple cores on the processor.
List<String> elements = Arrays.asList("element1", "element2", "element3");
elements.parallelStream()
.forEach(element -> performTimeConsumingOperation(element));
In this example, the parallelStream()
method converts the collection into a parallel stream, and the forEach()
method applies the Lambda expression in parallel to each element of the stream.
Best Practices for Efficient Parallelism
While parallelism offers the potential for significant performance improvements, it also comes with its own set of challenges. Here are some best practices to keep in mind when leveraging Java 8 Lambdas for parallelism.
1. Ensure Thread Safety
When performing operations in parallel, it's crucial to ensure that shared data is accessed safely to avoid race conditions and inconsistencies. Utilize concurrent data structures or synchronize access to shared resources where necessary.
2. Avoid Stateful Lambda Expressions
Stateful Lambda expressions, which rely on and modify external state, can lead to unexpected behavior when executed in parallel. It's better to encapsulate and manage state within the parallel operations to maintain deterministic behavior.
3. Consider Performance Trade-offs
While parallelism can improve the performance of certain operations, it's essential to benchmark and analyze the actual benefits. Not all operations are well-suited for parallel execution, and the overhead of parallelism may outweigh the gains in some scenarios.
4. Use Proper Parallelism Control
Java 8 provides mechanisms for controlling parallelism, such as the parallel()
and sequential()
methods. Understanding when to switch between parallel and sequential execution can significantly impact performance.
Harnessing the Power of Lambda Expressions with Libraries
In addition to the Stream API, several libraries leverage Java 8 Lambda expressions to enable efficient parallelism and simplify concurrent programming. The CompletableFuture class in Java 8 provides a powerful way to compose asynchronous computations using Lambda expressions.
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
// Perform asynchronous task using a Lambda expression
System.out.println("Asynchronous task executed by Lambda expression");
});
Moreover, frameworks like RxJava and Project Reactor extend the concept of functional programming to enable reactive and parallel programming paradigms in Java.
The Last Word
With Java 8 Lambda expressions and the Stream API, parallelism in Java has become more accessible and convenient than ever before. By embracing the functional programming paradigm and following best practices, developers can unlock the full potential of parallelism in their applications. Whether it's performing computationally intensive tasks or leveraging multiple cores for improved throughput, Java 8 Lambdas pave the way for efficient and scalable parallelism.
In conclusion, mastering Java 8 Lambda for efficient parallelism opens up a world of possibilities for building high-performance, concurrent applications. By understanding the principles of parallelism and leveraging the features of Java 8, developers can take their coding skills to new heights.
For more in-depth understanding, you can explore the official Java documentation on Lambda Expressions and Stream API. Happy coding!