Maximizing Profit: Solving the Knapsack Problem
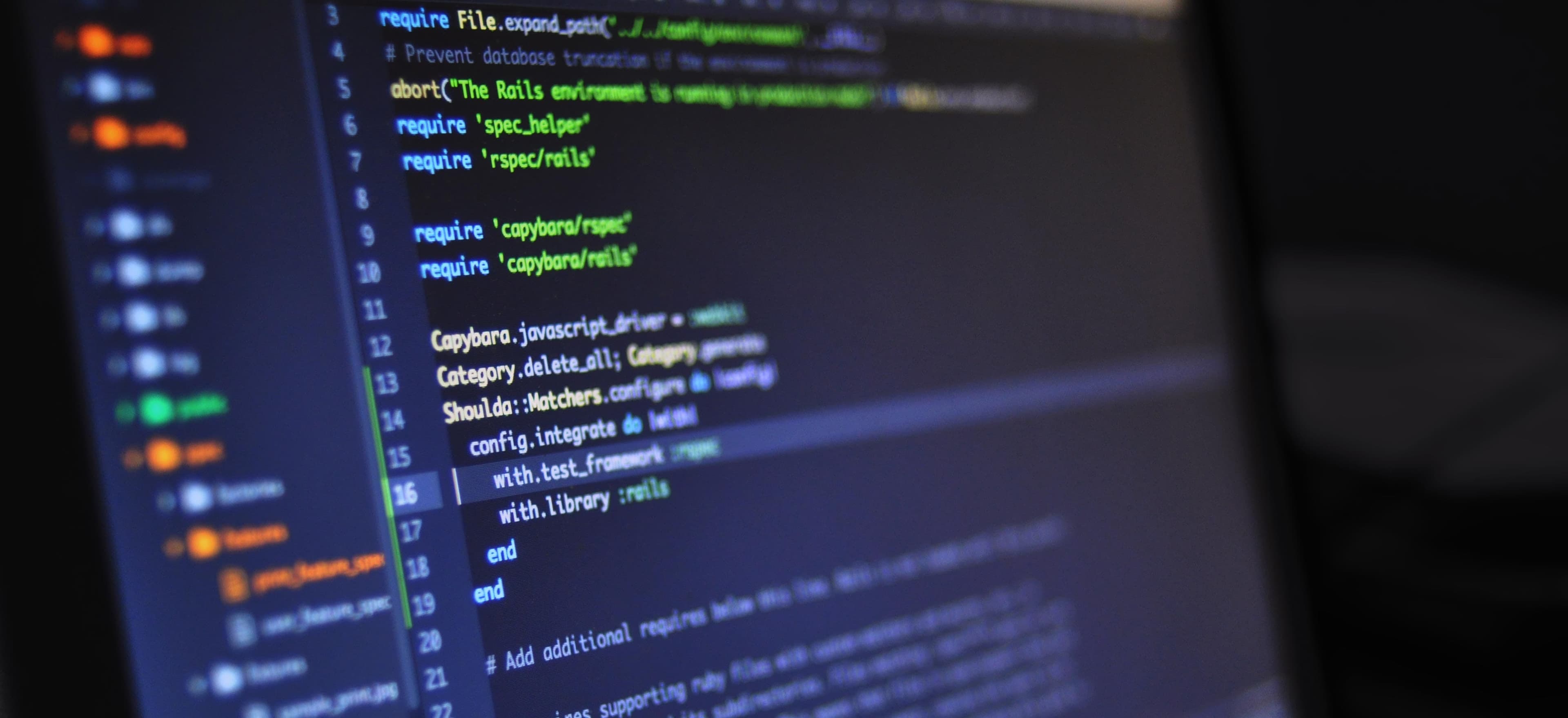
- Published on
Maximizing Profit: Solving the Knapsack Problem
The knapsack problem is a classic optimization problem in computer science and mathematics. It can be defined as follows: given a set of items, each with a weight and a value, determine the number of each item to include in a collection so that the total weight is less than or equal to a given limit, and the total value is maximized. This problem has a wide range of applications, from resource allocation to financial portfolio management.
In this blog post, we will explore how to solve the knapsack problem using dynamic programming in Java. We will start by understanding the problem statement, then delve into the dynamic programming approach, and finally, implement the solution in Java. Let's get started!
Understanding the Knapsack Problem
Imagine you are a thief and want to steal the most valuable items from a store. However, your knapsack (or backpack) has a limited capacity, so you cannot take everything. The knapsack problem is about determining the most valuable combination of items to steal without exceeding the weight limit of your knapsack.
Each item has a weight and a value, and the goal is to maximize the total value of the items included in the knapsack while ensuring that the total weight does not exceed the capacity of the knapsack.
Dynamic Programming Approach
Dynamic programming is a powerful technique for solving optimization problems like the knapsack problem. The key idea behind dynamic programming is to break down a complex problem into simpler subproblems and solve each subproblem only once, storing its result to avoid redundant computations.
For the knapsack problem, we can use dynamic programming to build a table where each cell represents the maximum value that can be obtained with a certain weight and a certain number of items included. By filling in this table, we can efficiently compute the maximum value for any given weight and number of items, ultimately solving the knapsack problem.
Implementing the Solution in Java
Let's dive into the implementation of the knapsack problem using dynamic programming in Java. We will create a class called Knapsack
that encapsulates the logic to solve the problem.
public class Knapsack {
public int solveKnapsack(int[] weights, int[] values, int capacity) {
int n = weights.length;
int[][] dp = new int[n + 1][capacity + 1];
for (int i = 1; i <= n; i++) {
for (int w = 1; w <= capacity; w++) {
if (weights[i - 1] <= w) {
dp[i][w] = Math.max(values[i - 1] + dp[i - 1][w - weights[i - 1]], dp[i - 1][w]);
} else {
dp[i][w] = dp[i - 1][w];
}
}
}
return dp[n][capacity];
}
}
In the solveKnapsack
method, we initialize a 2D array dp
to store the maximum values. We then iterate through the items and capacity, filling in the table based on the maximum value that can be obtained at each step.
Why Dynamic Programming?
Dynamic programming offers an efficient solution to the knapsack problem by avoiding redundant computations and leveraging the optimal substructure property of the problem. The table filled during the dynamic programming process effectively caches the results of subproblems, leading to a significant reduction in time complexity compared to naive recursive or iterative approaches.
By using dynamic programming, we ensure that the solution is both optimal and scalable, making it suitable for real-world applications with large datasets.
Closing Remarks
In this blog post, we have explored the knapsack problem and its dynamic programming solution in Java. We have gained a solid understanding of the problem statement, the dynamic programming approach, and its implementation in Java.
Dynamic programming provides an elegant and efficient way to solve the knapsack problem, making it a valuable tool for tackling a wide range of optimization problems in practice.
To further deepen your understanding of dynamic programming and the knapsack problem, I highly recommend checking out GeeksforGeeks knapsack problem page, which offers additional insights and variations of the problem.
I hope this blog post has provided you with a clear understanding of how to maximize profit by solving the knapsack problem using dynamic programming in Java. Happy optimizing!