Unpacking Apache Ant: Mastering Single File Programs
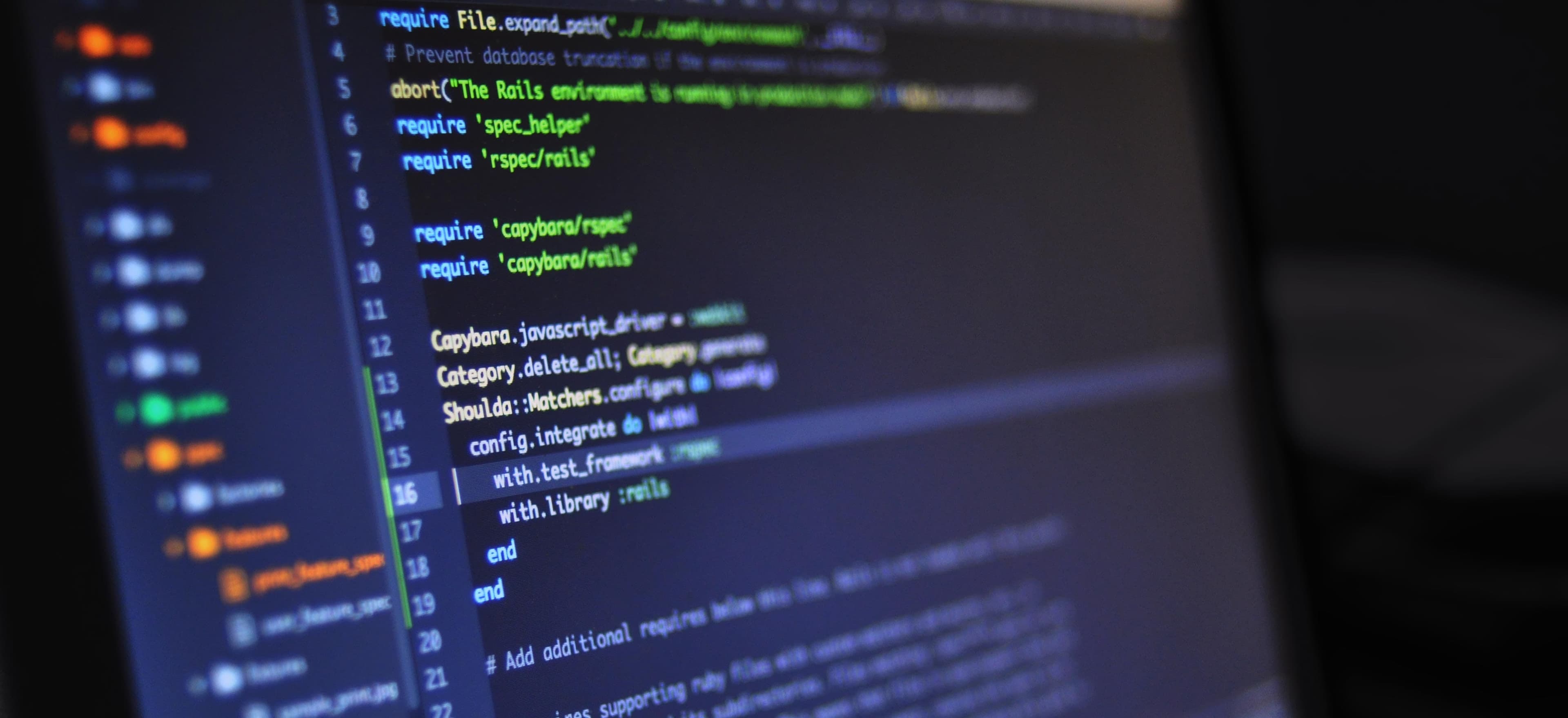
- Published on
Unpacking Apache Ant: Mastering Single File Programs
Apache Ant is a Java library and command-line tool used for automating software build processes. While it is typically used for building and deploying larger software projects, it can also be leveraged to streamline the development of single file programs. In this post, we will delve into the process of setting up and utilizing Apache Ant for managing and automating the build process of single file Java programs.
Understanding Apache Ant
Apache Ant is a versatile and powerful tool for automating software build processes. It employs XML-based configuration files to define the build process and manages dependencies, compilation, testing, and packaging of applications. While Ant is commonly used in enterprise-scale projects, its capabilities can effectively streamline the development of smaller, single file Java programs.
Setting Up Apache Ant
Step 1: Download and Install Apache Ant
To begin, download the latest version of Apache Ant from the official website (https://ant.apache.org/). Once downloaded, follow the installation instructions for your operating system to set up Ant on your machine.
Step 2: Configure Environmental Variables
After installing Apache Ant, set the ANT_HOME
environmental variable to the directory where Ant is installed. Additionally, add the Ant bin
directory to your system's PATH
variable to enable running Ant commands from any directory in the command line.
Creating a Single File Java Program
Let's start by creating a simple single file Java program that we will use to demonstrate the build process with Apache Ant.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
The above code snippet represents a basic "Hello, World!" program in Java. Save this code in a file named HelloWorld.java
.
Creating the build.xml File
Now, we will create a build file named build.xml
, which will define the build process for our single file Java program.
<project name="HelloWorld" basedir="." default="compile">
<target name="compile">
<javac srcdir="." destdir="." includeantruntime="false" />
</target>
<target name="run" depends="compile">
<java classname="HelloWorld" />
</target>
</project>
In the above build.xml
file, we have defined two targets - compile
and run
. The compile
target utilizes the javac
task to compile the HelloWorld.java
file, while the run
target executes the compiled program.
Running the Build Process
Compiling the Program
To compile the single file Java program, navigate to the directory containing the build.xml
and HelloWorld.java
files in the command line and run the following command:
ant compile
The ant compile
command triggers the build process defined in the build.xml
, compiling the HelloWorld.java
file and generating the corresponding HelloWorld.class
file.
Running the Program
After successfully compiling the program, execute it by running the following command:
ant run
The ant run
command executes the Java program, producing the output Hello, World!
.
Bringing It All Together
In this post, we explored the utilization of Apache Ant for automating the build process of single file Java programs. By setting up Apache Ant, creating a build file, and executing the build process, we successfully compiled and ran a simple Java program. Incorporating Apache Ant into the development workflow of single file programs can significantly enhance efficiency and reproducibility.
In conclusion, while Apache Ant is often associated with complex build processes, its capabilities can be harnessed to streamline the development of smaller-scale Java applications. By understanding and leveraging the features of Apache Ant, developers can master the automation of single file programs, fostering an organized and efficient development environment.