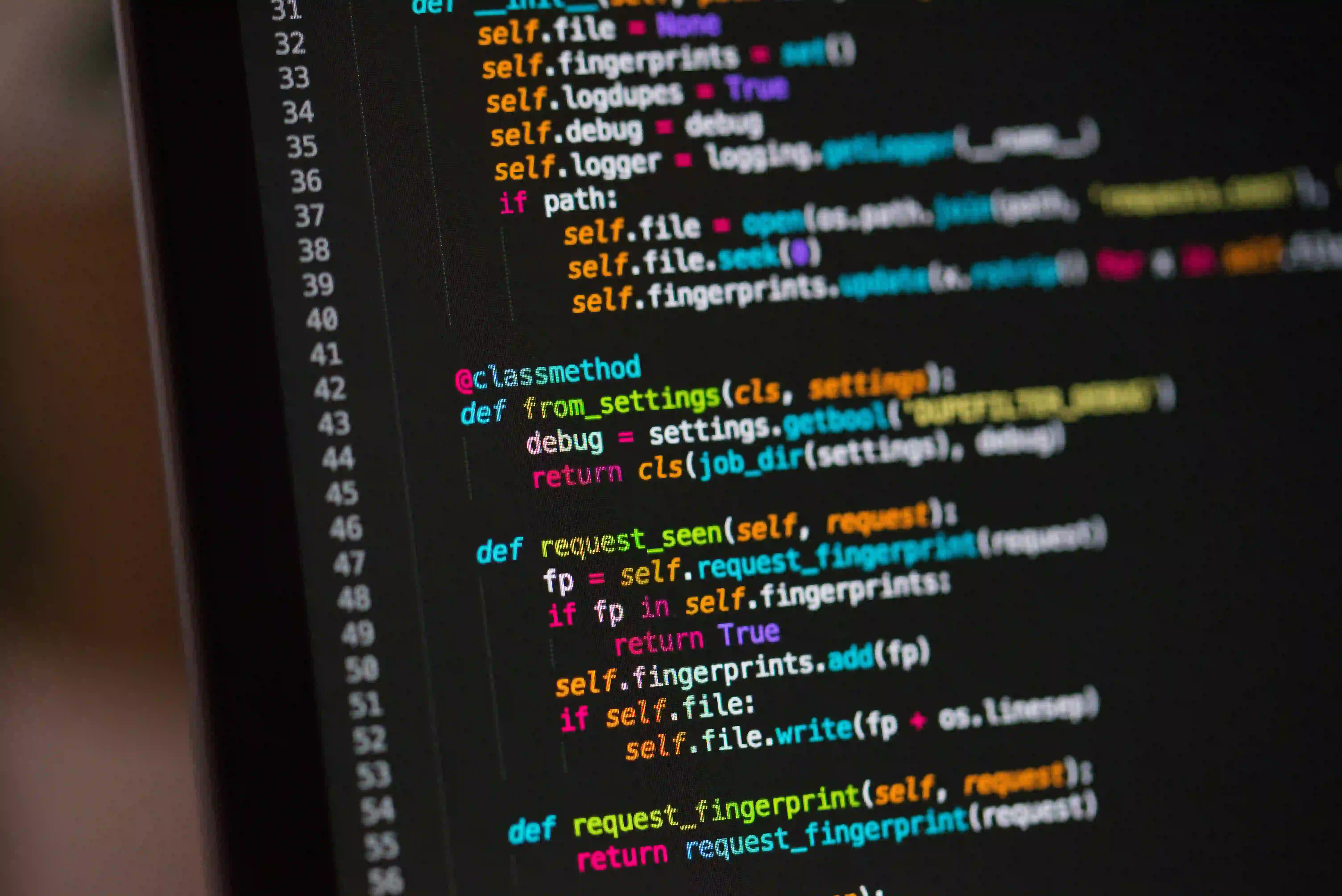
Lock It Down: Securing Spring Boot Metrics with Prometheus
When it comes to monitoring and gathering metrics from your Spring Boot applications, Prometheus is a popular choice among developers. Prometheus provides powerful querying and alerting capabilities, making it a valuable tool for understanding the behavior of your applications in real-time. However, as you expose metrics to Prometheus, it's crucial to ensure that your endpoints are secure to prevent unauthorized access or potential attacks. In this post, we'll delve into the process of securing Spring Boot metrics with Prometheus, ensuring that your monitoring data remains safe and reliable.
Why Secure Your Spring Boot Metrics Endpoint?
Exposing your application metrics to an external system like Prometheus without adequate security measures can pose serious risks. Unauthorized access to your metrics could lead to data breaches, performance degradation, or even potential exploitation of vulnerabilities. By securing your metrics endpoint, you can control who has access to your monitoring data and prevent malicious actors from tampering with your application's metrics.
Exporting Metrics with Spring Boot Actuator
Spring Boot Actuator is a powerful feature that allows you to monitor and interact with your application. It provides several built-in endpoints to access metrics, health checks, and other information about your application. When combined with Prometheus, Spring Boot Actuator can export metrics in a format that Prometheus can scrape and store for further analysis.
To export metrics from Spring Boot Actuator to Prometheus, you need to include the micrometer-registry-prometheus
dependency in your project.
dependencies {
implementation 'io.micrometer:micrometer-registry-prometheus'
}
The above code snippet demonstrates how to include the micrometer-registry-prometheus
dependency in your build.gradle
file.
By incorporating this dependency, your Spring Boot application will automatically begin exporting metrics in a format that Prometheus can understand.
Securing the Metrics Endpoint
Now that your Spring Boot application is exporting metrics to Prometheus, it's crucial to secure the metrics endpoint to prevent unauthorized access. To achieve this, you can utilize Spring Security, a highly customizable and powerful authentication and access control framework.
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/actuator/prometheus").hasRole("PROMETHEUS")
.and()
.httpBasic();
}
}
In the code snippet above, we define a custom SecurityConfig
class that extends WebSecurityConfigurerAdapter
. By using the authorizeRequests
method, we specify that access to the /actuator/prometheus
endpoint is restricted to users with the PROMETHEUS
role, and we enforce HTTP basic authentication.
With this configuration, only users with the PROMETHEUS
role will have access to the metrics endpoint, ensuring that unauthorized users are unable to retrieve sensitive monitoring data.
Managing User Roles and Credentials
To complement the security configuration, you need to manage user roles and credentials to regulate access to the metrics endpoint. This can be achieved by defining an in-memory user store with specific roles and credentials.
@Configuration
public class InMemorySecurityConfig {
@Bean
public UserDetailsService userDetailsService() {
UserDetails user = User
.withUsername("prometheus")
.password("{noop}password")
.roles("PROMETHEUS")
.build();
return new InMemoryUserDetailsManager(user);
}
}
In the code snippet above, we define an InMemorySecurityConfig
class and use a UserDetailsService
bean to create a user with the username "prometheus", password "password", and the role "PROMETHEUS". The password is prefixed with {noop}
to specify that the password is not encoded.
By managing user roles and credentials in this manner, you can control access to the metrics endpoint and ensure that only authorized users with the designated role can retrieve monitoring data.
Lessons Learned
In conclusion, securing your Spring Boot metrics endpoint when integrating with Prometheus is essential for safeguarding your monitoring data. By leveraging Spring Security and managing user roles and credentials, you can control access to the metrics endpoint and prevent unauthorized access. This ensures that your monitoring data remains secure and reliable, enabling you to gain valuable insights into the behavior of your applications without compromising on security.
By following the steps outlined in this post, you can confidently integrate Spring Boot with Prometheus for monitoring and metrics gathering while upholding the highest standards of security.
Now you are ready to enhance the security of your Spring Boot metrics with Prometheus and ensure the protection of your valuable monitoring data.
So, what are you waiting for? Secure your Spring Boot metrics endpoint with Prometheus today!
To dive deeper into Spring Boot, consider checking out Spring Boot official documentation and Prometheus user guide, both of which provide comprehensive insights into the topics covered in this post.
Stay secure, stay monitoring!