Demystifying Java JSON Schema Validation Issues
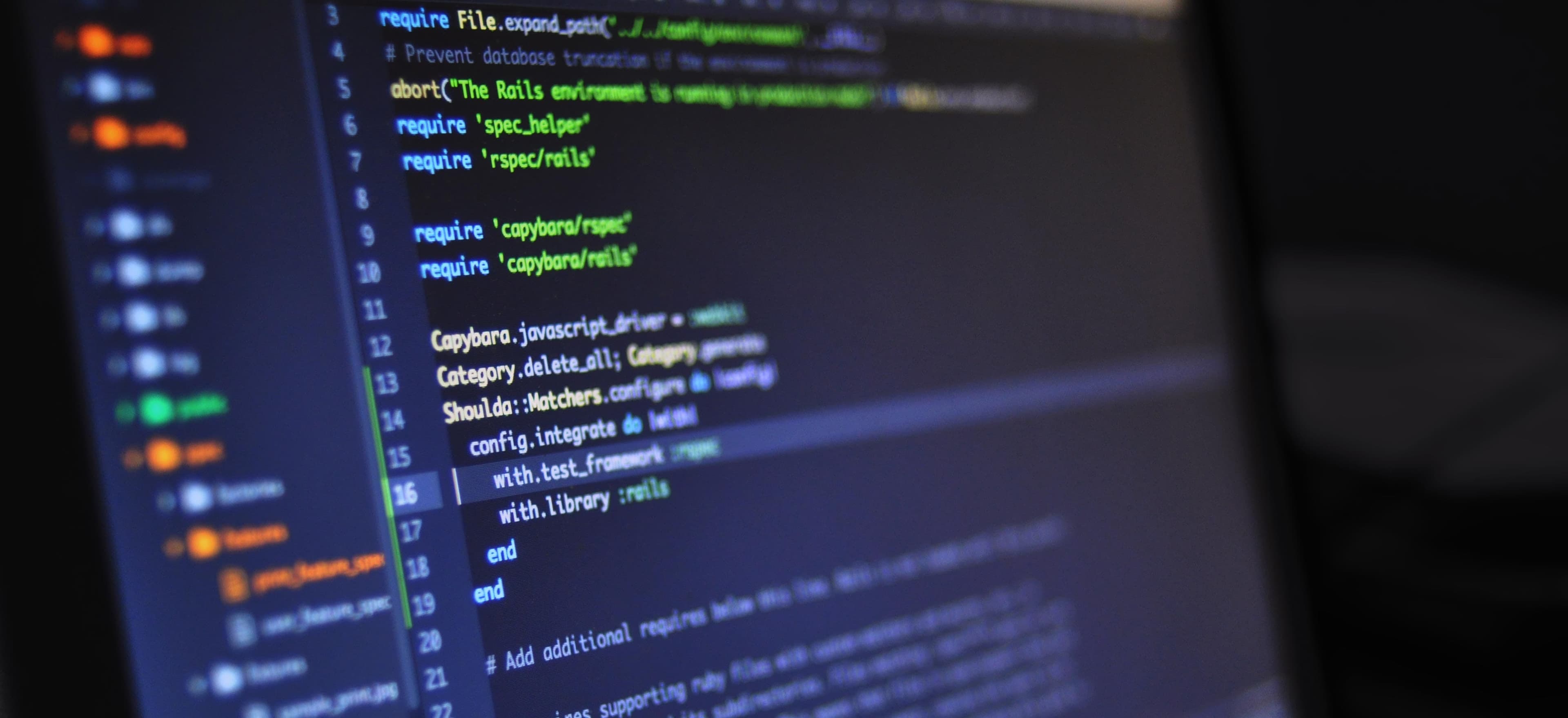
- Published on
Demystifying Java JSON Schema Validation Issues
JSON (JavaScript Object Notation) has become a ubiquitous part of modern web development, and Java has robust support for working with JSON data. However, validating JSON data against a defined schema is crucial for ensuring its integrity and accuracy. In this post, we will explore the ins and outs of JSON schema validation in Java, discuss common issues developers face, and provide solutions to demystify the process.
Understanding JSON Schema Validation
Before delving into Java-specific implementations, let's understand the concept of JSON schema validation. JSON Schema is a vocabulary that allows you to annotate and validate JSON documents. It provides a clear, human-readable way to express what data is allowed and how it should be structured. Think of it as a blueprint for your JSON data, outlining its expected shape, types, and constraints.
Leveraging Java Libraries for JSON Schema Validation
In the Java ecosystem, several libraries offer JSON schema validation capabilities. One popular choice is the org.everit.json.schema
library, which provides full support for JSON schema validation according to the JSON Schema standard. Another contender is com.github.fge:json-schema-validator
, which also offers a robust set of features for validating JSON data against a schema.
Example: Using org.everit.json.schema
import org.everit.json.schema.Schema;
import org.everit.json.schema.ValidationException;
import org.everit.json.schema.loader.SchemaLoader;
import org.json.JSONObject;
public class JsonSchemaValidator {
public static void validateAgainstSchema(JSONObject json, String schemaString) {
try {
Schema schema = SchemaLoader.load(new JSONObject(schemaString));
schema.validate(json);
System.out.println("JSON is valid against the schema.");
} catch (ValidationException e) {
System.out.println("JSON is invalid against the schema: " + e.getMessage());
}
}
}
In this example, we utilize org.everit.json.schema
to validate a JSON object against a provided schema. The SchemaLoader.load
method loads the schema from a JSON object, and schema.validate
checks the input JSON for compliance with the schema. If validation fails, a ValidationException
is thrown, providing valuable insights into the issues encountered.
Common Challenges and Solutions
1. Loading Schema from a File
Challenge: Often, JSON schemas are stored in separate files, and loading them into Java applications for validation can be tricky.
Solution: Utilize Java's file handling capabilities to read the schema from a file and pass it to the schema validation library.
2. Handling Complex Schemas
Challenge: Dealing with complex JSON schemas with nested structures and intricate validation rules can lead to confusion and errors.
Solution: Break down complex schemas into smaller, manageable components and utilize referencing within the schema to maintain clarity and reusability.
3. Integrating with Frameworks and APIs
Challenge: Integrating JSON schema validation seamlessly with existing frameworks and APIs can be a daunting task.
Solution: Leverage Java frameworks such as Spring Boot, Micronaut, or JAX-RS, which provide built-in support for integrating JSON schema validation into RESTful services and API endpoints. This ensures consistent validation across the application.
Best Practices for Effective JSON Schema Validation
To ensure a smooth and effective JSON schema validation process in Java, consider the following best practices:
-
Modularize Schemas: Divide complex schemas into modular components and reference them as needed to maintain clarity and reusability.
-
Use Enumerations: Utilize enumeration constraints in schemas to define a list of allowed values for specific properties, enhancing data quality and integrity.
-
Error Handling: Implement robust error handling mechanisms to provide clear and actionable feedback when validation fails, aiding in troubleshooting and resolution.
-
Testing: Thoroughly test JSON schema validation with various input scenarios, including edge cases and boundary conditions, to validate its correctness and reliability.
-
Documentation: Document the schema structure, validation rules, and usage guidelines to aid developers who interact with the JSON data and schemas.
My Closing Thoughts on the Matter
In this post, we have demystified JSON schema validation in Java, exploring its significance, common challenges, best practices, and solutions. By leveraging Java libraries such as org.everit.json.schema
and adhering to best practices, developers can robustly validate JSON data against schemas, ensuring its integrity and adherence to predefined rules. Embracing JSON schema validation as an integral part of Java applications empowers developers to handle JSON data confidently and reliably, paving the way for robust and secure data handling.
Remember, JSON schema validation is not just about ensuring compliance; it's about instilling confidence in the integrity of your JSON data, ultimately strengthening the foundation of your Java applications.
For further exploration of JSON schema validation and its role in modern Java development, consider diving into the detailed specifications provided by JSON Schema and the wealth of resources offered by the official Java JSON Processing API. Happy coding and validating!
Checkout our other articles