Unveiling JDK 14: How JEP 305 Revolutionizes Type Checks
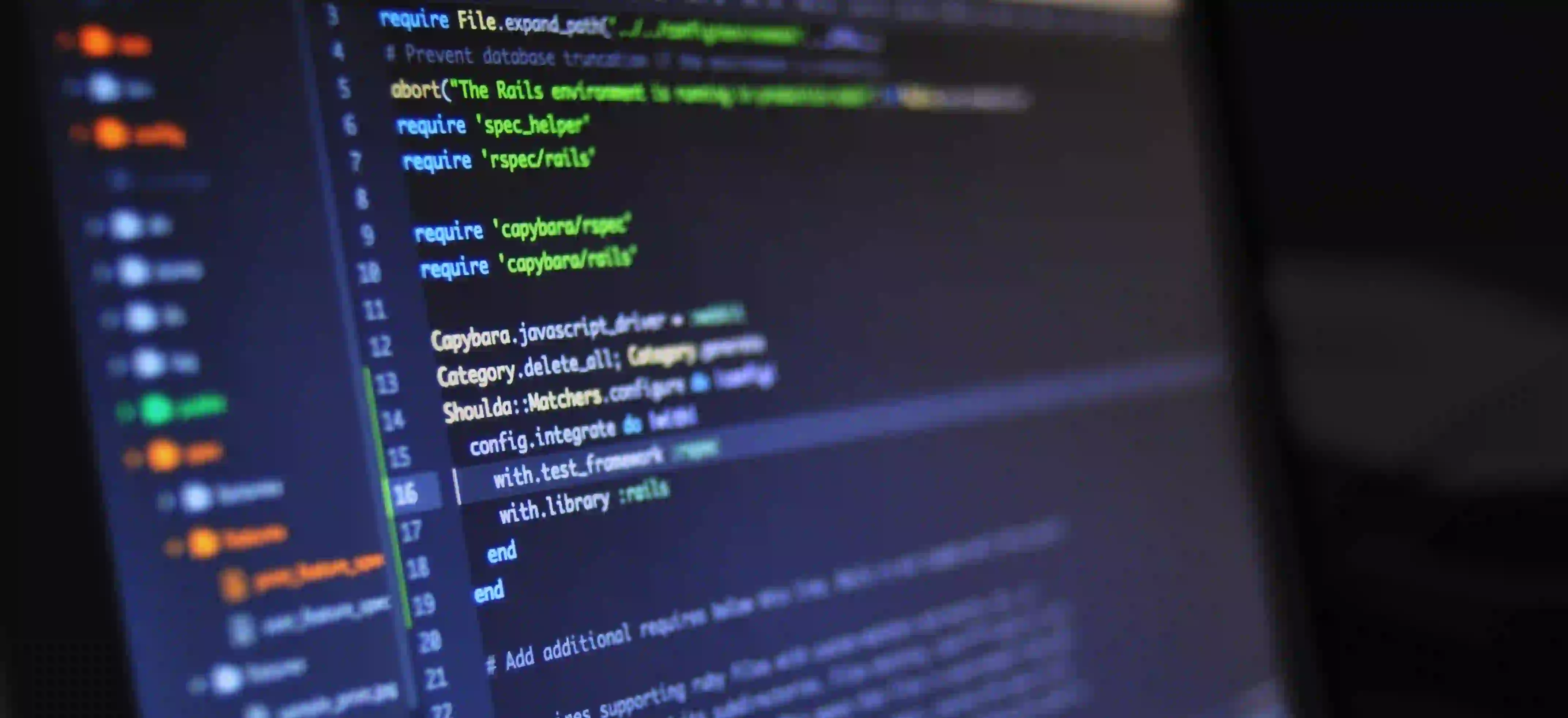
Unveiling JDK 14: How JEP 305 Revolutionizes Type Checks
Java has always been known for its strong typing system, providing robust support for static type checking. With the release of JDK 14, Java Enhancement Proposal (JEP) 305 has revolutionized the way type checks are performed, offering enhanced capabilities and improved developer experience. In this blog post, we will delve into the details of JEP 305 and explore how it has transformed type checks in Java.
Background: Type Checking in Java
Type checking is an essential aspect of any programming language, ensuring that the types of variables and expressions are compatible within a given context. In Java, this process occurs at both compile time and runtime, enabling early detection of type errors and improving the overall reliability of the code.
Historically, performing type checks in Java involved explicit casting and instanceof checks, which could be cumbersome and error-prone, especially when dealing with complex hierarchical class structures. JEP 305 aims to address these limitations and streamline the type checking process through the introduction of a more concise and expressive syntax.
Introducing Pattern Instanceof
One of the key features introduced by JEP 305 is the concept of pattern instanceof, which allows developers to combine type checks and type casts into a single, more succinct syntax. This new language feature simplifies the code and enhances readability, making it easier to express complex type conditions in a clear and concise manner.
Let's take a look at an example to understand the power of pattern instanceof. Consider a scenario where we need to perform type checks and casting for a class hierarchy comprising various subclasses. In traditional Java code, this could lead to verbose and error-prone checks. However, with pattern instanceof, the same logic can be expressed in a much more elegant fashion:
if (obj instanceof String s) {
// Use 's' as a String within this block
} else if (obj instanceof Integer i) {
// Use 'i' as an Integer within this block
} else {
// Fallback logic
}
In the above code snippet, the pattern instanceof construct not only checks the type of the object but also performs an implicit cast, binding the object to a new local variable of the specified type within the corresponding block. This not only eliminates the need for explicit casting but also enhances the clarity and conciseness of the code.
Enhanced Type Inference with Switch Expressions
In addition to pattern instanceof, JEP 305 also enhances the type inference capabilities of switch expressions. Prior to JDK 14, switch statements could be verbose and error-prone, requiring explicit casting and redundant code. With the introduction of pattern matching for switch, the process has been simplified, allowing for more concise and readable code.
Let's consider a scenario where a switch statement is used to handle different types within a class hierarchy. In previous versions of Java, this could result in repetitive type casting and boilerplate code. However, with the improved type inference in JDK 14, the same logic can be expressed with greater brevity:
String result = switch (obj) {
case String s -> "String: " + s;
case Integer i -> "Integer: " + i;
default -> "Unknown type";
};
In the above code snippet, the switch expression leverages pattern matching to handle different types elegantly, extracting the values directly without explicit casting. This not only simplifies the code but also reduces the potential for runtime errors, leading to more robust and maintainable code.
Compatibility and Migration Considerations
As with any language enhancement, it's crucial to consider the implications for existing code and the compatibility of the new features. While JEP 305 brings significant improvements to type checks in Java, it's important to note that these changes may impact the compatibility of pre-existing code and libraries.
Developers intending to adopt JDK 14 and leverage the new type checking features should carefully assess the impact on their existing codebase and ensure compatibility with any third-party libraries or frameworks. Additionally, migrating to the latest version of Java may necessitate adjustments to the codebase to fully benefit from the enhanced type checking capabilities.
In Conclusion, Here is What Matters
JEP 305 introduces a significant evolution in type checking for Java, empowering developers with more concise syntax, improved readability, and enhanced type inference. The introduction of pattern instanceof and the advancements in switch expressions streamline the type checking process, leading to more robust, maintainable, and expressive code.
As Java continues to evolve, it's essential for developers to stay abreast of the latest language features and enhancements, leveraging them to enhance the quality and efficiency of their code. JEP 305 stands as a testament to the commitment of the Java community to continuously improve the language and empower developers with powerful and expressive constructs.
In conclusion, JEP 305 in JDK 14 revolutionizes type checks in Java, paving the way for more elegant and reliable code. Embracing these enhancements can elevate the development experience and contribute to the evolution of Java as a modern, expressive, and developer-friendly language.
To explore more about JEP 305 and its impact on Java development, you can refer to the official JDK 14 release notes and JEP 305 specification.